A circular structure is an object that references itself. In the example below, we are referencing the object (obj) as a value for the location key.
let obj = { name: "John", age: 23, gender: "Male", location: obj }
Like XML, JSON (JavaScript Object Notation) is used for storing and exchanging data. JSON is much more easier to parse, or divide, than XML and is preferably used when converting objects into strings with the JSON.stringify()
method.
Error When Trying to Convert Circular Structures into JSON
JSON does not support object references, so trying to stringify a JSON object that references itself will result in a typeerror. It is an error that can be thrown when attempting to change a value that cannot be changed or when using a value in an inappropriate way.
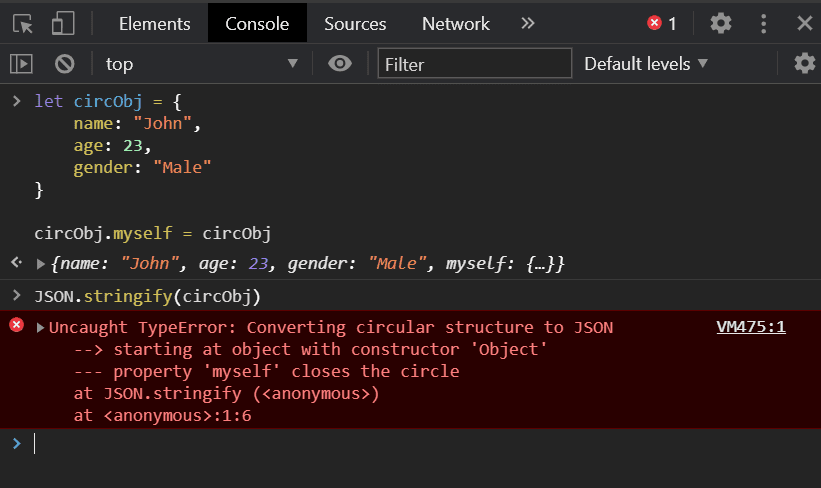
Possible Solutions
JSON.stringify()
not only converts acceptable objects into strings, but it also contains a replacer parameter that can replace values if the function being passed in is specified to do so. Let’s break down the code below to understand how this happens.
const replacerFunc = () => { const visited = new WeakSet(); return (key, value) => { if (typeof value === "object" && value !== null) { if (visited.has(value)) { return; } visited.add(value); } return value; }; }; JSON.stringify(circObj, replacerFunc());
In our replacerFunc
above, we are calling on the WeakSet
object, which is an object that stores weakly held objects, or references to objects. Each object in a WeakSet
may only occur once, thus filtering out repeated or circular data. The new keyword is an operator that creates a blank object.
In our return statement, we have nested if statements. Our first if statement is using the typeof operator which returns the type of primitive (Undefined, Null, Boolean, Number, String, Function, BigInt, Symbol) being evaluated.
If our type of value is strictly equal to an object and that object value is not null, it will continue onto the second if statement, checking to see if the value is in the WeakSet()
.
When we invoke JSON.stringify()
, we pass in both our original circular structure and our replacer function.
let circObj = { name: "John", age: 23, gender: "Male" } circObj.myself = circObj const replacerFunc = () => { const visited = new WeakSet(); return (key, value) => { if (typeof value === "object" && value !== null) { if (visited.has(value)) { return; } visited.add(value); } return value; }; }; JSON.stringify(circObj, replacerFunc());
This will give us our desired stringified result in the console.
"{"name":"John","age":23,"gender":"Male"}"
Some other possible solutions for this error is the utilization of libraries like circular-json, which is a circular JSON parser, or cycle.js, which was created for IE8 users.
Circular JSON serializes and deserializes otherwise valid JSON objects containing circular references into and from a specialized JSON format.
Conclusion
JSON does not support object references, so trying to stringify a JSON object that references itself will result in a typeerror.
A circular structure is an object that references itself. To be able to stringify such objects, developers can utilize the replacer parameter in the stringify() method, making sure the function that is being passed in, filters out repeated or circular data.
Utilizing libraries like circular-json can also be a solution around this error.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.