Starting in the programming world can be difficult. There might be a lot you don’t know in the field and it may take some time to figure it all out. While you can learn a lot at coding bootcamps, college, or through online courses, there are certain things that you will still have to learn on the job. Knowing coding best practices can save you from extra stress.
This article aims to serve as a guide on some essential coding practices. You will also get relevant information on critical coding concepts, common coding challenges, and resources to learn coding best practices. You will learn how to avoid making costly coding mistakes and break into the tech field by the end of this article.
What Is Coding?
Coding, also known as computer programming, is the process whereby a computer or machine gets a set of instructions on actions to perform or carry out. It is the way that humans generally communicate with computers and machines. With coding, you can create websites, applications, and other advanced technologies.
Most of today’s technological advancements, such as social media platforms, video games, mobile applications, and operating systems, are created through coding with a programming language. However, so much more can be done with coding knowledge, such as data analysis, web development, and web design.
10 Concepts You Need to Understand for Coding Best Practices
To fully understand and apply coding best practices in your daily routine as a programmer, you need to be familiar with certain concepts. This section includes a few of them, which will also be helpful throughout your coding career.
- Variables. A variable is one of the essential aspects of coding. It is a container that stores data or bits of data and information for later use or reference by computer programs. Every programmer uses variables as labels to reference data and make it easily understood by anyone reading the code.
- Modules. Modules separate a program’s functionality into small independent and interchangeable bits that contain important information needed for executing coding projects. Programmers use these modules, each comprising a different program, to make their jobs easier.
- Headers. Headers, also known as payload or body in data transmission, are additional data added at the top of a block of stored or transmitted data. It is an integral part of a data block that contains transparent information about the file or transmission.
- Hardcode. A hardcode is a part of a computer program that you can only change by changing the program’s source code. It is an incorrect coding practice because when software has been compiled and is ready for execution, the program’s hardcode doesn’t change, regardless of how you manipulated the software.
- Tools. Computer programming relies heavily on tools. They are pieces of software that enable programmers to write code faster. They contribute to process simplification and accuracy. An example of a programming tool is Integrated Development Environment (IDE).
- IDE. IDE is a software application that allows computer programmers to develop software. It usually comes with a source code editor, builds automation tools, and a debugger.
- Source Code. Source code is the human-readable code for your program that you write as a programmer using a text editor or a visual programming tool and then save in a file. The source code is then compiled and converted into binary machine code.
- Deep Nesting. Nesting refers to a code that exists to perform a specific function and is contained within a code that performs a broader function. Deep nesting refers to the use of control structures to nest statement blocks. It occurs when information is organized in layers and objects containing objects similar to one another.
- Bug. A bug is an error in a computer program that causes that program to malfunction or produce incorrect or unexpected results. They can cause a whole computer system to crash if not addressed promptly and accurately. You must run multiple tests to detect bugs and then fix them.
- Constants. The data in a computer program is referred to as a constant or a variable. Constants come in two types, named or literal, and include data values that can’t be altered or changed during execution.
5 Common Challenges That Coding Guidelines Can Address
There are particular challenges that all programmers come across, even with years of experience. You must ensure that your code has a friendly user interface while still achieving your ultimate goal. Some of these challenges are highlighted below and can be addressed by following coding guidelines.
Debugging
Bugs are quite common in computer programming. Regardless of how great your code is, you are still likely to come across a couple of bugs. The solution to this is debugging, which removes bugs or errors in a software or computer program. While debugging isn’t always difficult, most bugs are tough to eliminate, which could be problematic for new programmers.
Compiler Error Messages
An error occurs when a compiler fails to compile a piece of computer program source code. These particular error messages could happen because of problems within the blocks of code or in the compiler itself. Compilation error messages are often valuable for debugging source code for programmers and typically come as a series of messages.
The first message is usually the correct message and what programmers should fully listen to. The other messages occur as a result of the confused state of the compiler. Compiler errors can be very difficult to figure out, especially for beginners.
Multithreading
Multithreading is when a programmer divides a single program into multiple threads and runs them as separate programs simultaneously. While this is an excellent way to finish your program quickly, you will most likely encounter numerous errors because the codes will run separately and can be unpredictable. You can overcome this problem by using tools like monitors and semaphores.
Encryption
Encryption converts a plain text message into ciphertext that retains the original meaning and can be decoded back into the original message. It is a procedure used to keep your data secure. However, a single blunder could jeopardize the entire process, leaving your data open or lost for good.
Writing Unreadable Code
As a beginner, it could be pretty easy to get carried away and show off your skills by writing code that no one else can read or understand. However, this shows how smart you are but also leaves you with a code that is inefficient and unusable by anyone. Well-written code should be efficient and readable, no matter your level of coding expertise.
Top 10 Coding Best Practices and Guidelines
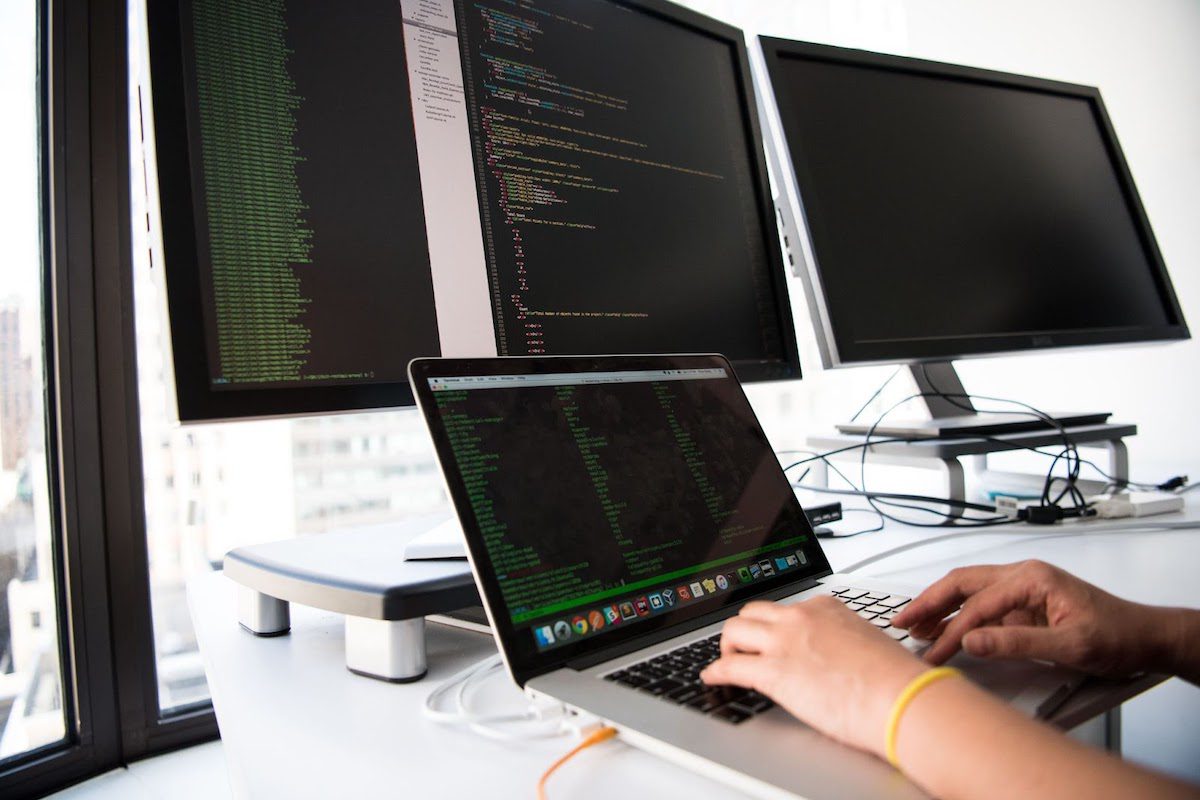
This section contains some of the most essential coding best practices to help you write highly efficient and usable code. Adhering to them will help you avoid mistakes that you are likely to make when beginning your programming career.
Write Readable Code
It is important to write code that anyone can read. This makes it easy for your code to be understood, followed, and easily integrated within new systems. Writing code that you and your colleagues understand makes it easier for others on your team to help with software projects.
Even though code readability takes time and space, it is still easier to be understood and followed by others. This includes using appropriate variable names and notes within your code to specify what each function is doing. As a beginner, get in the habit of conducting a code review after running your script to ensure there are no bugs for future users.
Stick to Your Style Guide
Every programming language has a style guide to give you details on how to write a code. It also includes indenting code, placing braces and spaces, and naming conventions. Following a style guide will make your code more readable and easier for others to follow.
It is essential to follow the style guide rules and not cut corners. This promotes consistency and makes it easy to detect errors and bugs. Aside from programming languages, companies also have their own style guides that their developers have to work with. This ensures that the team is on the same page and that the code is as efficient as possible.
Adhere to Industry-Specific Coding Standards
Each industry has its required coding standards that you have to follow as a developer. You should ensure that you find out the standards for the industry you’re writing the code for and adhere to them. This will help you write efficient and satisfactory code. It will also meet the expectations of the user as well as the product.
Use Consistent Headers for Different Modules
Using the same headers for different modules means that your modules have the same format, making your code easier to understand and maintain. This way, it’s easier for anyone on your team to recognize and read your code. You may also use descriptive names to make it easy for yourself and other programmers to understand what your code does.
Test Your Code and Respond to Errors
Testing your code doesn’t make you any less of an expert in coding, it only makes you efficient. Regardless of your skills, your code still needs to be tested to ensure no avoidable errors will come back later on. While this might take some time, it will only help you provide an even better code that others can use.
You should always respond to errors as soon as possible. Even though they might make your code longer and less readable, it is better to have an error-free code than a readable code that can’t be used. There are automated testing tools available that you can utilize to make this a more straightforward process for you.
Try Pair Programming
Pair programming is a technique that involves two programmers working together on a code. This is an excellent way to write even better, readable, and more efficient code. By doing this, you learn from each other and find unique approaches to coding.
Other than pair programming, you can also collaborate with other members of your team. This helps you see things from a different perspective, but more importantly, it will help your team progress. Having multiple people working on a project can improve the code because there will always be someone with a unique idea that could lead to a breakthrough.
Comment and Document
You should make inline comments on your code while working on it from the beginning. This helps you to stay consistent and make comments throughout the code. The comments should include relevant information regarding possible errors, functions and their parameters, and the code string’s design.
This is even more important if your code is complex. You should also document the entire function of the code to help whoever may use it understand it and its purpose. Your documentation should also include building, testing, installing, and using the code. Your document should be like a guide to help the user understand the exact functions of the code.
Don’t Hardcode
It is important to avoid hardcoding during your project because it will most likely cause issues down the line. This may involve security risks that could jeopardize your project. The only thing that you are allowed to hardcode is constants. This will save you from dealing with significant consequences that you could get from taking shortcuts.
Practice the DRY Principle
The DRY principle is one that you should follow at all times. DRY stands for ‘Don’t Repeat Yourself’ and states that “Every piece of knowledge must have a single, unambiguous, authoritative representation within a system.” The meaning is self-explanatory as it simply means you should never repeat your code. It is also known as DIE, which is ‘Duplication is Evil.’
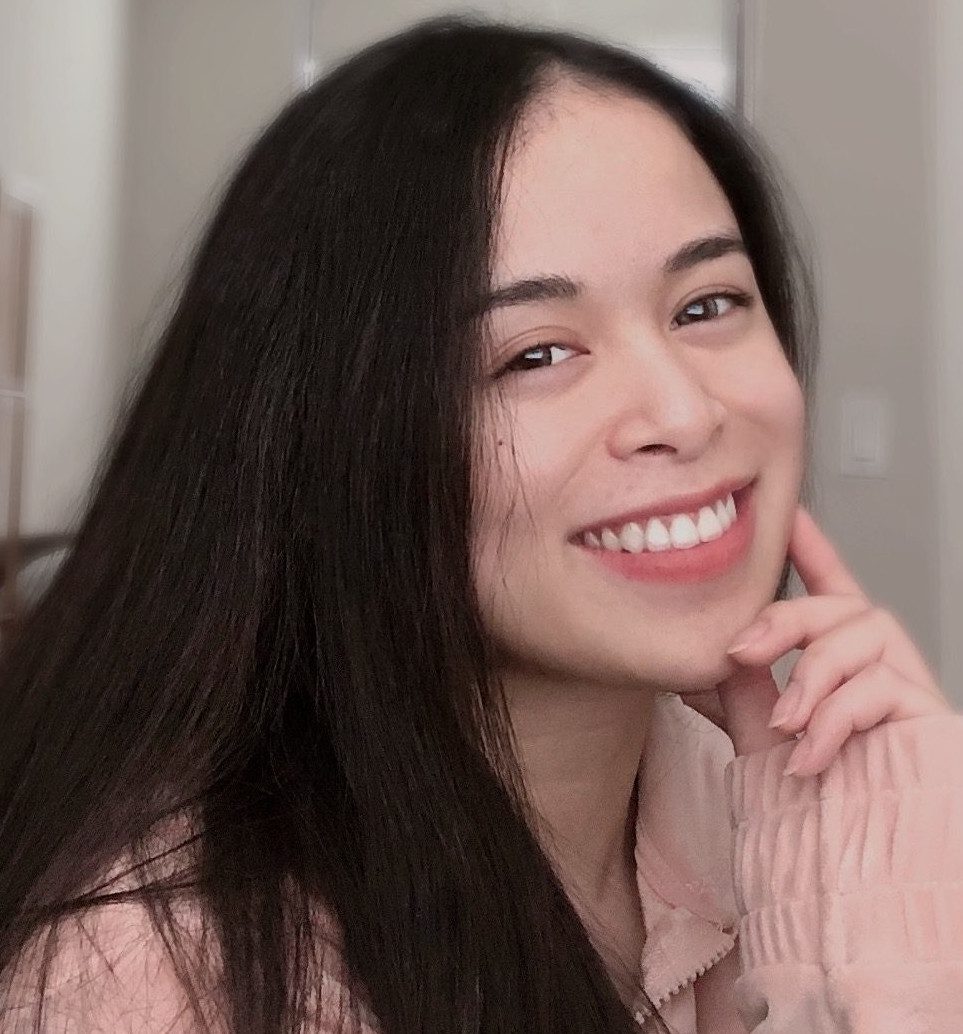
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
If there is any need for it, you can take out the important parts of a code and abstract them into a routine. You can also automate repetitive tasks when necessary, but you should not repeat a piece of code within a script.
Avoid Deep Nesting
Deep nesting can make your code harder to read and follow and should be avoided at all costs. It is possible to make specific changes to your code to reduce nesting and encourage readability.
How to Learn Coding Best Practices
You can utilize many sources to learn the best coding practices. You can attend a coding bootcamp, take an online class, or read relevant books. This section contains a few of the best resources to learn coding best practices.
Can a Bootcamp Help You Learn Coding Best Practices?
A bootcamp can help you learn coding best practices because they are designed to prepare you to start a rewarding tech career. Coding bootcamps are short-term and fast-paced training programs designed to prepare students for careers in technology. With the right approach, you will learn coding practices that will help you write excellent programs.
Beyond offering coding training, most coding bootcamps provide career services to help prepare you for the job market. These services allow you to land good jobs and put your learned skills to good use, furthering your knowledge in the field.
Best Courses and Training Programs to Learn Coding Best Practices
Provider | Course | Price |
---|---|---|
Coursera | Coding for Everyone: C and C++ Specialization | Free |
Flatiron School | Software Engineering Bootcamp | $16,900 |
Pluralsight | JavaScript Core Language | Free |
Thinkful | Software Engineering Bootcamp | $16,000 |
Udemy | Coding for Beginners 1: You Can Learn to Code! | $85 |
Should You Learn Coding Best Practices?
You should learn coding best practices to avoid making mistakes that could strain your career. These coding practices will help you avoid writing code that provides unexpected results or unsatisfactory feedback from users. It also makes it easier for others to use your work. If you don’t learn these practices, you are likely to learn the hard way on the job.
Coding Best Practices and Guidelines FAQ
Coding standards and best practices are guidelines and techniques for writing efficient, readable, and understandable code with minimal errors. They are used by computer programmers to have a consistent coding format and write efficient code. Some of them include comments and documents, sticking to your style guide, and adhering to industry-specific standards.
C# standard coding are standards that C# programmers follow. Some of them include using suitable casing like camelCase or Pascal case, using the right formatting, using access modifiers, and auto-properties.
1. Your code should be safe to use without causing harm
2. It should be secure and not open to security threats
3. It should be reliable enough to function at any time
4. It should be available for testing at any level
5. It should be maintainable even when it grows
6. It should be able to perform excellently regardless of the environment
Coding guidelines exist to ensure that all codes are written so that they can be used, tested, and maintained by anyone at any time. It also makes it possible to prevent each software developer or programmer from writing code that no one understands.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.