When choosing which of the many programming languages you want to learn, going with the one that’s arguably the most widely-used is a pretty good bet. And that would be Java. Because Java has been around since the mid 1990s, it is a mature language. Many of the kinks present in the release of any new product have been worked out, and an extensive community of mentors and problem-solvers has grown up around it.
What this means for someone just starting out with coding is that the coding basics of Java can almost certainly be used for whatever you’re interested in (which isn’t true for a language like Ruby), and you’ll be able to find help when you encounter problems.
For all these reasons, Java is a great language to learn. Here, we’re going to learn about coding basics for Java.
What Is Java?
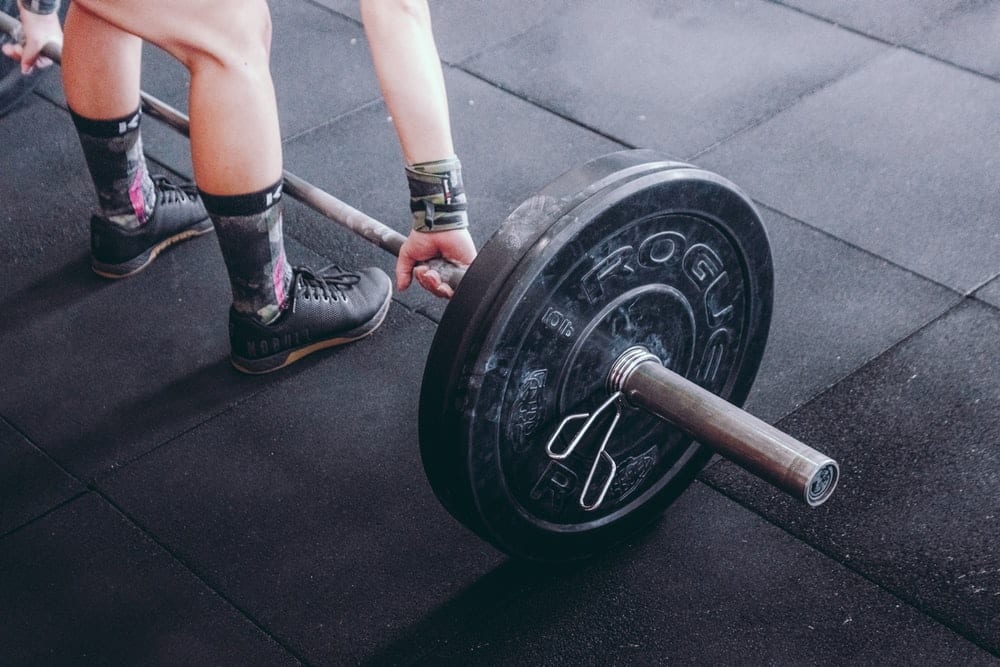
Java is a general-purpose, open-source language that’s been around for almost 15 years. It has found use in data science, machine learning, general AI, web development, and many other domains. Java was designed to be secure and relatively easy to learn. One popular way of gauging a programming language’s complexity is to see how hard it is to print ‘Hello World’. It’s a coder tradition that this be the first thing you do with a new programming language.
Here it is in Java:
- class HelloWorld {
- public static void main(String[] args) {
- System.out.println(“Hello, World!”);
- }
- }
This is more complicated than Ruby or Python, but still reasonably straightforward.
One of the hallmarks of Java is that it is both platform- and architecture-independent. Java uses various technical tricks to ensure that its programs are runnable on many processors, platforms, and systems. This has contributed significantly to its mass adoption.
The Basics of Coding in Java
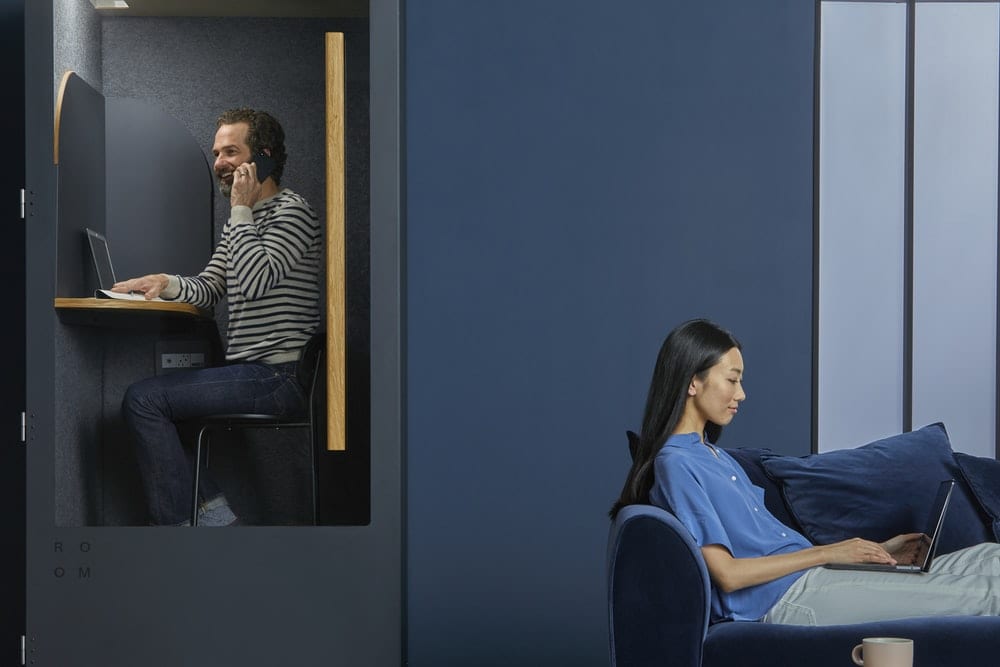
Java is object-oriented, so it’s helpful to understand what this means. In simple terms, object-oriented programming languages treat everything as an object with its own state and methods. Because of the way object-orientation encourages coders to structure their programs, it’s a favored paradigm for larger and more complicated projects.
The absolute best way to learn Java is to start making things with it. We’ve already seen it used for ‘Hello, World’, and a great next step would be to read this edureka Java tutorial. It discusses local, instance, and class variables (very important concepts for beginning programmers to get straight), important Java data types, and many of the features of object-oriented programming in the context of Java.
Learn Java Online is a great website for coders new to programming and new to Java. Most importantly, it’s interactive. Coding well requires that you learn to model the behavior of a program, and the only way to accomplish this is to write plenty of code. Being able to do this in the browser is great, as you won’t have to set up your own environment, and the tutorial covers topics like conditionals and flow control.
As I never tire of pointing out, coding is a living skill that can only be learned through repeated practice. If you don’t want to go through the tutorials listed above, or you want to supplement them, I’d recommend going through a series of projects of escalating difficulty.
Once you’ve printed ‘Hello World’, practice using Java’s native functionality to manipulate strings generally by concatenating a few strings, finding the length of a string, or looping through the characters. Speaking of looping, conditionality is a foundational programming skill. A great next task would be familiarizing yourself with Java for, while, and do while loops.
After you’re comfortable here, I’d move on to defining your own methods and classes. With all of that, you should be well on your way to mastering one of the programming world’s favorite languages!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.