C#, pronounced C sharp, was developed in 2000 by Danish Software Engineer Anders Hejlsberg for Microsoft. It’s a high-level programming language used by many tech industry professionals. As the demand for C# skills has increased over the years, more tech professionals are using C# exercises and quizzes to improve their skills.
C# is a functional, object oriented programming language, so it’s a good idea for beginners to learn it. If you’re wondering where you can practice C#, you’ve come to the right place. We’ll go over all the resources you can turn to if you want to get help with C#. We’ll also explore the best C# exercises and outline tutorials and websites that can help you.
C# Exercises to Help You Learn C#
If you want to learn C# to develop desktop applications, video games, enterprise software, and mobile apps, you should start with some exercises. Since the language is still in active development, there’s always something new to learn. So even if you’re not new to C#, the 10 exercises below will allow you to practice your skills to keep up with this changing language.
10 C# Exercises and Practice Problems (With Solutions)
1. Online Attendance
This is a popular C# event handling exercise. It requires you to write an online attendance program that includes everyone except Jack, Steven, and Matthew. For most people who sign in, the application should display the message “Welcome [user name].” But when one of the three people mentioned tries to sign in, it should sound an alarm.
Solution: The program will include several C# variables and functions for the user name, the admin’s email, warning alerts, systems to stop the alert, and approved members. Once it’s complete, the program will start beeping every time you input the banned names. You must hit “ctrl +c” to stop the alarm.
2. Hello World
Hello World is a popular practice program for all C languages. It is a simple exercise that introduces the beginner to the C# language and touches on fundamental concepts. In this exercise, you need to insert the missing part of a C# code.
Solution: Your code snippets should include a namespace declaration, class declaration and definition, class members, the main method, and statements of expression.
3. Write Hello World Without Using WriteLine
Unlike the previous exercise, this exercise doesn’t use the WriteLine method. The WriteLine method displays the text representation for specified objects, which is handy for the Hello World program. However, in this exercise, you need to use either the Console.OpenStandardOutput(), BeginWrite(), AsyncWaitHandle.WaitOne(), or Console.ReadKey() method.
Solution: Using the ASCII format, display all of the characters on your string. You will then use the methods above to prepare your codes and print your final output.
4. Reverse a Number
Reversed numbers are common in programming, especially if you’re adding and subtracting numbers. These are whole numbers where the digits of one number are the 0–0s9te of another number. For example, 298 and 892 form reversed numbers. This exercise guides you through how to write a program that can reverse a number using C#.
Solution: You will use loop and arithmetic operators to prepare your code. Input the original number from the user using the public static void, including Console.Write and Console.ReadLine.
5. Find the Length of an Array
An array is a structure comprised of a series of boxes that each hold a piece of data, which can often be an array of integers. Using arrays makes it easier for engineers to manage large amounts of data. In this exercise, you will develop a program that can determine the length of an array using C#.
Solution: You should include classes and use Console.WriteLine and ReadLine, and static void to find out the length of the main array.
6. Add Two Numbers Using Function
Functions allow programmers to break down coding problems into smaller code snippets. Functions also enhance readability and are reusable. Therefore, writing a program that uses functions to add two numbers is only reasonable.
Solution: This exercise uses public class, public static, public static void, and the Writeline method to complete the program.
7. Insert an Element in Array
Generally, an array is a collection of elements that contain the same data type. For example, you can create an array for integers. This exercise facilitates the process of writing a program that can insert an element, including input, output, conditionals, loops, or data structures, into an array.
Solution: You will start by selecting the desired element, then identify the position where the element will be inserted, shift the array elements to create space, and insert your element.
8. Accept a String from Keyboard and Print It
A string is a programming data type used when programming data values feature sequenced characters. Strings can also be invisible, making it difficult to input them via the keyboard. Queue the exercise. You will write a program to identify and accept strings from a keyboard before printing it.
Solution: You will use the public static void to include the main characters and the Writeline method to derive the final output. You can access a simple tutorial on the w3resource website.
9. Convert Celsius to Fahrenheit
Writing a program that converts Celsius Degrees to Fahrenheit is one of the most popular C# exercises. The exercise tests your understanding of several C# concepts and coding efficiency. You will apply variables, data structures, functions, LINQ, and more.
Solution: For this exercise, begin by setting the Celsius temperature using the Writeline method, then convert it to Fahrenheit.
10. Convert Days Into Years
It is not uncommon for people to need to convert a period of time from days into years. This program is a good way to practice adding days to a base date and extracting the components.
Solution: You will input the age as the public static value and create functions that facilitate the conversion. In addition, it’s easiest to use 365 days and ignore leap years.
How to Get Help With C#
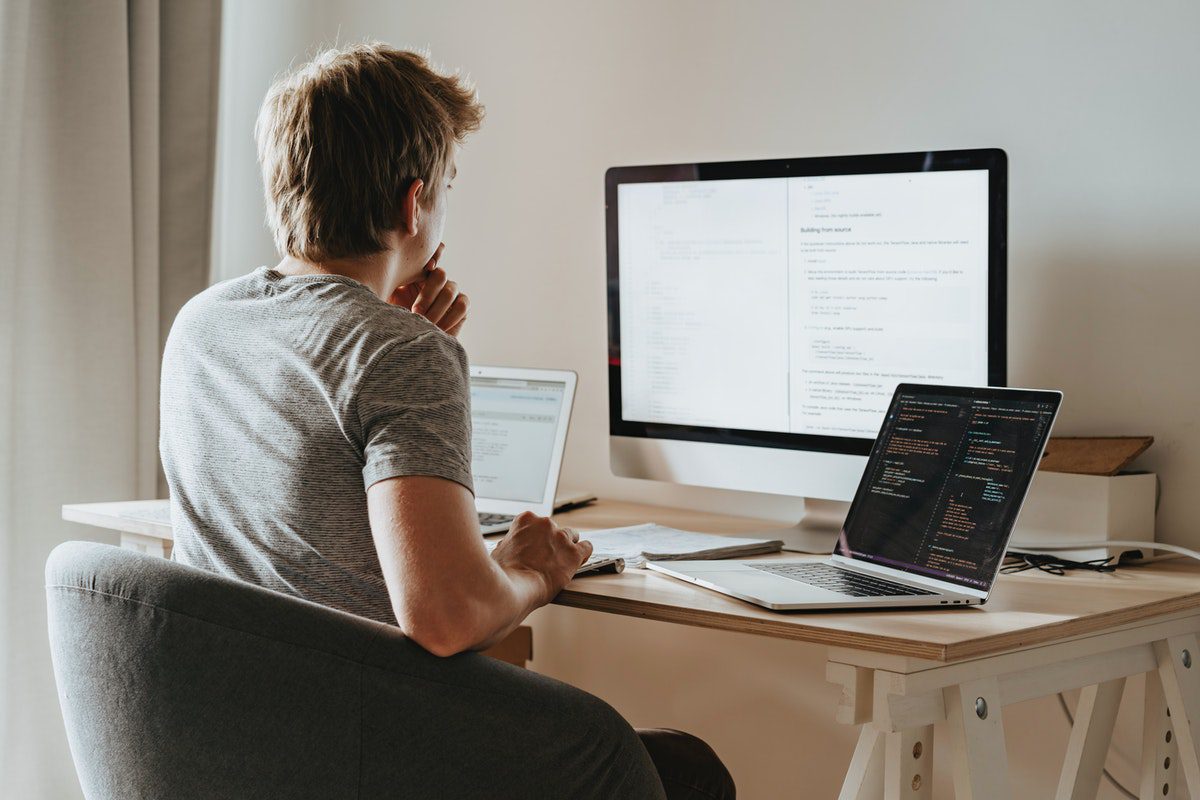
Apart from C# exercises, you can also learn the programming language through projects, quizzes, and blogs. You will find a large community of learners and C# experts who are willing to share ideas and help you understand the ins and outs of C#. Below are some ways you can get help with C#.
C# Exercises
C# exercises help you improve your C# coding skills via a hands-on approach. These exercises cover many different aspects of the C# language, so you can build your skills over time. You can find exercises covering data types, loops, arrays, or functions. The best part is that these exercises usually come with solutions, so you can compare your answers to see how you did.
C# Projects
Creating a C# project portfolio is necessary if you’re looking to become a C# developer. Completing C# projects will help you practice your technical skills in both front end and backend development. Additionally, by working on projects, you will gain practical experience that you’ll be able to apply later in a professional capacity.
You should start with simple, beginner-friendly projects like creating a social media bot or a digital signature app. Then, work your way up to more complex projects like building a 3D game. If you want, you can even participate in a C# hackathon, where you’ll compete with other developers to finish projects within a given time frame.
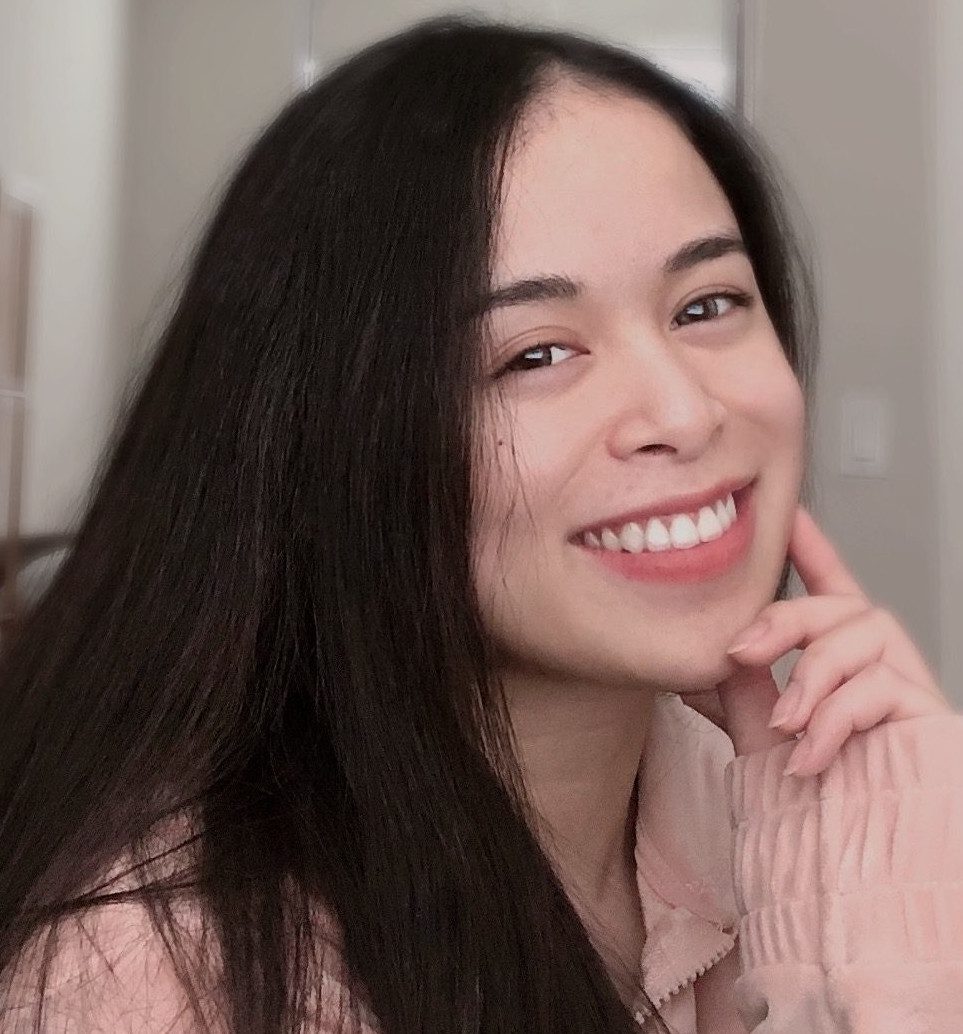
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
C# Quizzes
C# quizzes are an excellent way for you to gauge how far you’ve come in your C# knowledge. You can find them on many C# programming websites. They’re also a good resource if you’re preparing for an interview. They’re a simple way to rate your C# skills in areas like data types, variables, C# classes and objects, methods and arrays, and loops and conditionals.
C# Forums and Blogs
C# forums are interactive communities where people can share ideas and answer one another’s questions. These platforms allow C# learners to participate in discussions with seasoned industry developers and fellow learners. You can also share your exercises and projects, allowing people to contribute to your work or offer advice. Popular forums include Code Project, the C# forum on Stack Overflow, C# Corner, and Reddit’s C# forum.
C# blogs are a good place to learn more and get insight into other developers’ experiences with the coding language. You can even start your own blog to share your journey. You can check out the C# blogs at FoxLearn, Code with Shadman, Bill Blogs in C#, and Csharp Star Blog.
Where Can I Practice C#
As mentioned above, C# is in active development so it’s always being upgraded. Therefore, you must practice your skills continuously. In addition, to become a C# expert, you must get comfortable with complex C# exercises, quizzes, and projects. If you’re wondering, Below are some some resources that will allow you to practice C#.
- W3resource. This website is one of the largest online web development resources for both beginners and experienced C# developers. On it, you can find everything from basic algorithm exercises, string exercises, conditional statement exercises, and file handling exercises.
- Tutorials Teacher. Tutorials Teacher offers step-by-step C# tutorials for beginners and professionals. The tutorials are easy to understand and include C# tips and real-world examples. You can also answer C# coding questions or take a skills test to assess your knowledge level.
- C# Station. C# Station features helpful posts, documents, and tutorials for anyone interested in using C# with .NET. The C# tutorials on this site require that you use a code compiler and code editor, which you can also find on the website.
- W3Schools. This is an online learning platform that covers the fundamentals of web development. W3Schools offers free tutorials on all major programming languages, including C#. You can access C# quizzes, exercises, tutorials, code samples, and do-it-yourself tools that make learning C# easy.
- Tech Study. Tech Study offers over 200 C# exercises with solutions for beginners, intermediate developers, and experts. You can select from a long list of basic programs, conditional programs, array programs, and more.
What’s the Best Way to Learn C#?
The best way to learn C# is by attending a coding bootcamp. The best C# coding bootcamps will be entirely centered around the language. You will be learning from qualified C# professionals as you work on individual or team projects.
You’ll also have access to career coaches who can help you find your dream job in C#. If you’ve already attended a bootcamp, you can keep studying by taking C# courses online, reading C# books, or by visiting Microsoft’s official C# documentation page.
C# Exercises FAQ
Compared to programming languages like Python and C++, C# is not hard to learn. It is a beginner-friendly language because it is easy to read, it’s maintainable, and it comes with an extensive library. Furthermore, because it’s so widely used, you’ll have access to a variety of learning resources and communities that can help you if you get stuck.
Yes, you will need a basic understanding of C# before you can start on C# exercises. However, you’ll also be picking up many tips on how C# operates as you complete the exercises. For example, you’ll learn and practice variables, syntax, comparison operators, data types, logical operators, and classes.
With C# skills, you can work as a web developer, mobile app developer, software developer, video game developer, .NET developer, and more. C# skills are applicable in many industries, giving you the freedom to work where you desire.
C# is an object oriented programming language that allows you to control your data structure and reduce repetition. In addition, learning C# will give you access to high-paying job opportunities. After all, C# is an in-demand skill and employers will prioritize applicants with C# knowledge. So, once you’re well-versed in C#, you’ll be able to apply for a C# remote job or work in a major corporate office.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.