In C++ the stoi()
function is used to convert data from a string type to an integer type, or int. If using C++03 or earlier, the stringstream
class is used to convert string to int.
In C++, data types are used to distinguish particular types of data. For example, strings are used to store text, booleans are used to store true/false values, and integers are used to store whole numbers (numbers that do not have a decimal point).
When writing code in C++, there are situations where you need to convert values between different types. For instance, you may want to convert a string to an integer so you can perform mathematical operations on the value.
This tutorial will discuss, with reference to examples, how to convert a string to an integer in C++. We’ll discuss two approaches you can use for a string to integer conversion operation that combined cover all versions of C++.
C++ Data Types
There are a number of data types used in C++ to store data. For example, booleans are used to store true/false values, floats are used to store floating-point (decimal) numbers, and strings are used to store text-based data.
The type of data in which a value is stored affects how the value can be manipulated. For example, string operations can be used to manipulate the contents of strings, but they cannot be used with numerical values. So, when you’re working with data, knowing how to convert values to a different data type can be useful.
String to Int C++
There are two methods you can use to convert a string to an integer in C++.
If you are using C++03 or an earlier version of the programming language, you should use the stringstream class. If you are using C++11 or above, you can use the stoi()
function.
stringstream Class
The stringstream class is used to convert a string to an integer in C++03 and in earlier versions of C++. Before we can use stringstream, we have to import it into our code. We can do so using an include statement like this:
#include <sstream>
Now we’re ready to start using the sstream class. Here’s the syntax we can use to convert a string to an integer using stringstream:
stringstream intValue(stringValue); int number = 0; intValue >> number;
Let’s break down this syntax into its main components, which are as follows:
- stringstream instructs our program to use the stringstream class, which we will use to convert a string to an integer.
- intValue is used as an intermediary and holds the value of our string.
- stringValue is the string we want to convert to an integer.
- int number = 0; declares a variable called number which will hold our new integer value.
- intValue >> number assigns the value stored in intValue to the number variable.
Now, let’s walk through an example to demonstrate this method in action. Suppose we are writing a program that checks whether a customer’s ticket number for a cruise is valid. We have stored the customer’s ticket number as a string, but we want to convert it into an integer for later use in our program. We could convert our string to an integer using this code:
#include <iostream> #include <sstream> using namespace std; int main() { string ticket = "2029"; stringstream intTicket(ticketNumber); int ticketNumber = 0; intTicket >> ticketNumber; cout << "Ticket number: " << ticketNumber; }
Our code returns:
Ticket number: 2029
Let’s break down our code. First, we import the sstream library, which we use to access the stringstream method. We then use the using namespace std
notation to reduce the need to reference std when using methods from the standard library, such as cout.
In our main program, we define a string called ticket which stores the customer’s ticket number as a string value. We then use the stringstream method to convert the customer’s ticket number to a string and assign the new value to the intTicket variable.
Next, we declare a variable called ticketNumber and assign it the value 0. On the next line, we assign ticketNumber the value stored within the intTicket variable. Finally, we print out the statement Ticket number:
, followed by the contents of the ticketNumber variable.
stoi() Function
The stoi()
function is used to convert a string to an integer in C++11 and above.
The stoi()
function makes it much easier to convert a string to an integer, given its more readable syntax. Here’s the syntax for the stoi()
function:
int newValue = stoi(stringValue);
In this syntax, we pass one parameter through the stoi()
method called stringValue. Then, we assign the integer returned by stoi()
to the variable newValue.
Let’s return to the cruise ticket example from earlier. Suppose we want to convert the contents of a customer’s ticket from a string value to an integer. We could do so using this code:
#include <iostream> #include <string> using namespace std; int main() { string ticket = "2029"; int ticketNumber = stoi(ticket); cout << "Ticket number: " << ticketNumber; }
Our code returns:
Ticket number: 2029
As you can see, this program is significantly shorter than our previous example, which shows the efficient syntax offered by stoi()
in action.
Now, let’s break down our code. First, we import the string library which is used to work with strings in our program. We then declare that we are going to use the std namespace, like we did in the first example.
In our main program, we define a variable called ticket and assign it the string value 2029
. Then, we use the stoi()
method to convert the contents of the ticket variable to an integer and assign it to the variable ticketNumber. Finally, we print out the statement Ticket number:
to the console, followed by the value stored in the ticketNumber variable.
Conclusion
The stringstream method is used to convert a string to an integer in C++03 and earlier, and the stoi()
method is used to convert a string to an integer in C++11 and above.
This tutorial discussed, with reference to examples, how to convert a string to an integer using stringstream and stoi()
in C++. Now you’re ready to start converting strings to integers in C++ like a professional developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
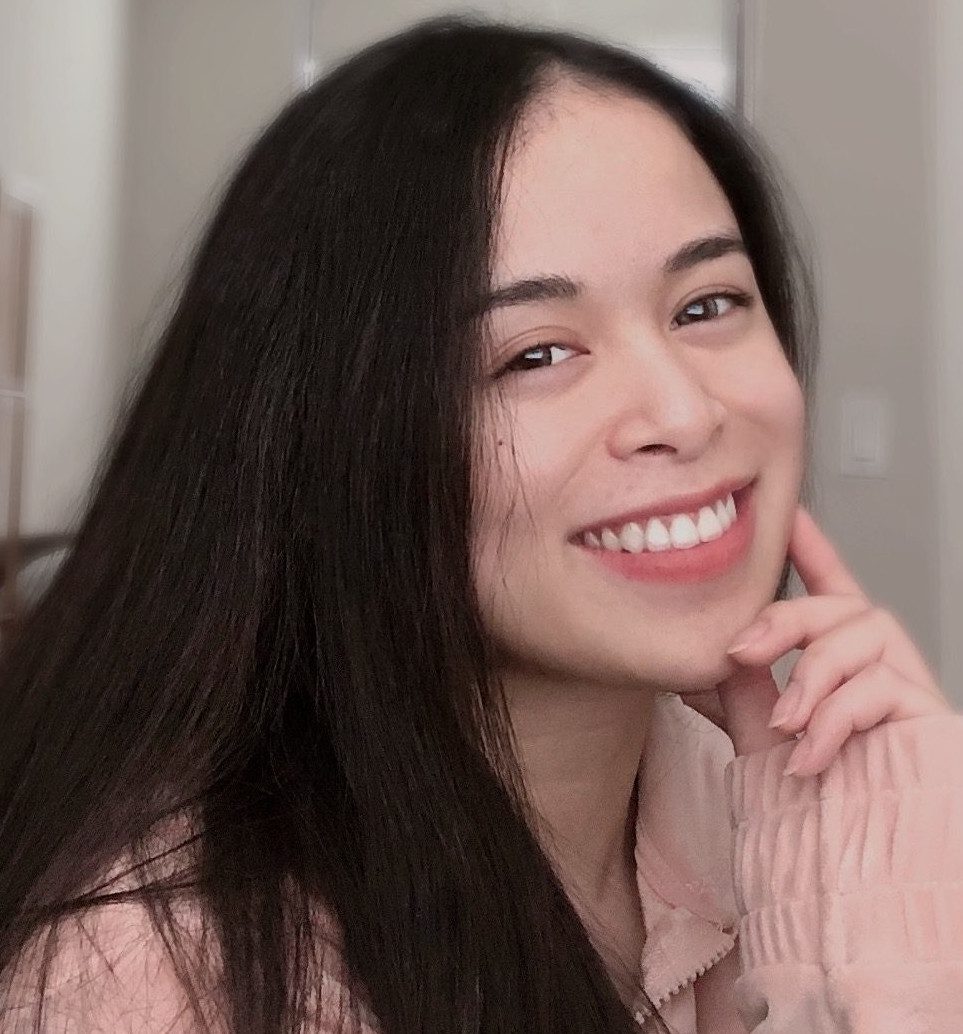
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot