How to Use C++ Stacks: A Guide
A stack is a data structure used to store data in a last-in, first-out (LIFO) order.
When you’re programming in C++, you may encounter a situation where using a stack is the best way to store data.
For instance, if you’re a teacher and you are building an app which tracks the homework assignments you have to grade, you may want to use a stack because you will want to grade the assignments based on the order they are stacked in your pile.
This tutorial will discuss, with reference to examples, the basics of C++ stacks, how to create a stack in C++, and how to store data within and manipulate the contents of a C++ stack. By the end of reading this tutorial, you’ll be an expert at using C++ stacks.
C++ Stacks
Stacks store items in a last-in, first-out (LIFO) order. This means that the last item added to the top of the stack is the first one that will be removed from the stack.
Let’s talk through an example to showcase how data is stored in a stack. Suppose you are an elementary school teacher who has just given their class a test.
At the end of the test, you asked the students to pile up the tests and hand them to you. You have no preference for the order in which the tests should be marked, so you will mark them in the order they have been handed to you. This is an example of a stack.
So, suppose you had three students who handed you their tests. Mark handed his test in first, Linda handed her test in second, and Cali handed her test in last. Here’s how these test would be stored in a stack:
Test |
Cali |
Linda |
Mark |
As you can see, Cali’s name appears at the top of the stack. This is because she handed her test in last, and the last item added to a stack is the first one out.
There are a few other instances where stacks are useful.
Think about how plates are stacked in a school canteen. The plate which is stacked at the top is the first one which will be taken by a student; the plate which is stacked at the bottom will be the last one out. Or think about a pile of books. The book at the top will be the first one you move if you want to look through your book pile.
Now that we know the basics of stacks, we can start to look at how to create and work with stacks in C++.
Create a Stack
Stacks are a type of data structure contained in the C++ stack library. This means that, in order to create a stack in C++, we need to import then reference the stack library. Here’s the code we can use to import a stack into a C++ program:
#include <stack>
Here is the syntax we use to create a stack in C++:
stack <dataType> stackName;
Let’s break this syntax down into its main components:
- stack tells our program to create a stack.
- dataType is the type of data our stack will store.
- stackName is the name we assign to our stack.
Suppose we wanted to create a stack called tests
which stores the tests that an elementary school teacher has to mark. We could use the following code to create this stack:
stack <string> tests;
In the above example, we created a stack called tests which can store string values.
Add an Item to a Stack
The push()
method is used to add an item to a C++ stack. This item will be added to the top of the stack. This means that elements are inserted and the last item inserted will appear at the top of the stack.
The push()
function accepts one parameter, which is the element you want to add to your stack.
Suppose we are creating a stack which stores the names of students whose latest elementary school test needs to be graded. Three students, Graham, Indra, and Sophia, have just handed in their test, and we want to add those tests to our stack in the order they were handed in. We could use the following code to do so:
#include <iostream> #include <stack> #include <string> using namespace std; int main() { stack <string> tests; tests.push("Graham"); tests.push("Indra"); tests.push("Sophia"); cout << tests.top(); }
Our code returns: Sophia
.
Let’s break down our example. First, we import the stack and string libraries which are used to work with the stack structure and the string data type, respectively. We then declare a stack called tests which stores string values.
Next, we use the push()
method to add three items to our stack: Graham, Indra, Sophia. These items are added in that order, and so Sophia is at the top of the stack and Graham is at the bottom of the stack.
On the next line, we use top()
to retrieve the item at the top of the stack and print it to the console. In this case, because Sophia was added last, her name is at the top of the stack, which means it is the one printed to the console.
Remove an Item from a Stack
The pop()
method deletes the item at the top of a stack, or in other words the item that was most recently added to the stack. pop()
accepts no parameters, because it removes the top most item in the stack.
Suppose we are working our way through grading the tests and we have just finished marking Sophia’s test. We want to remove Sophia’s test from our stack so that we can see which test we need to mark next. We could use the following code to remove Sophia’s name from the stack and update our stack of tests:
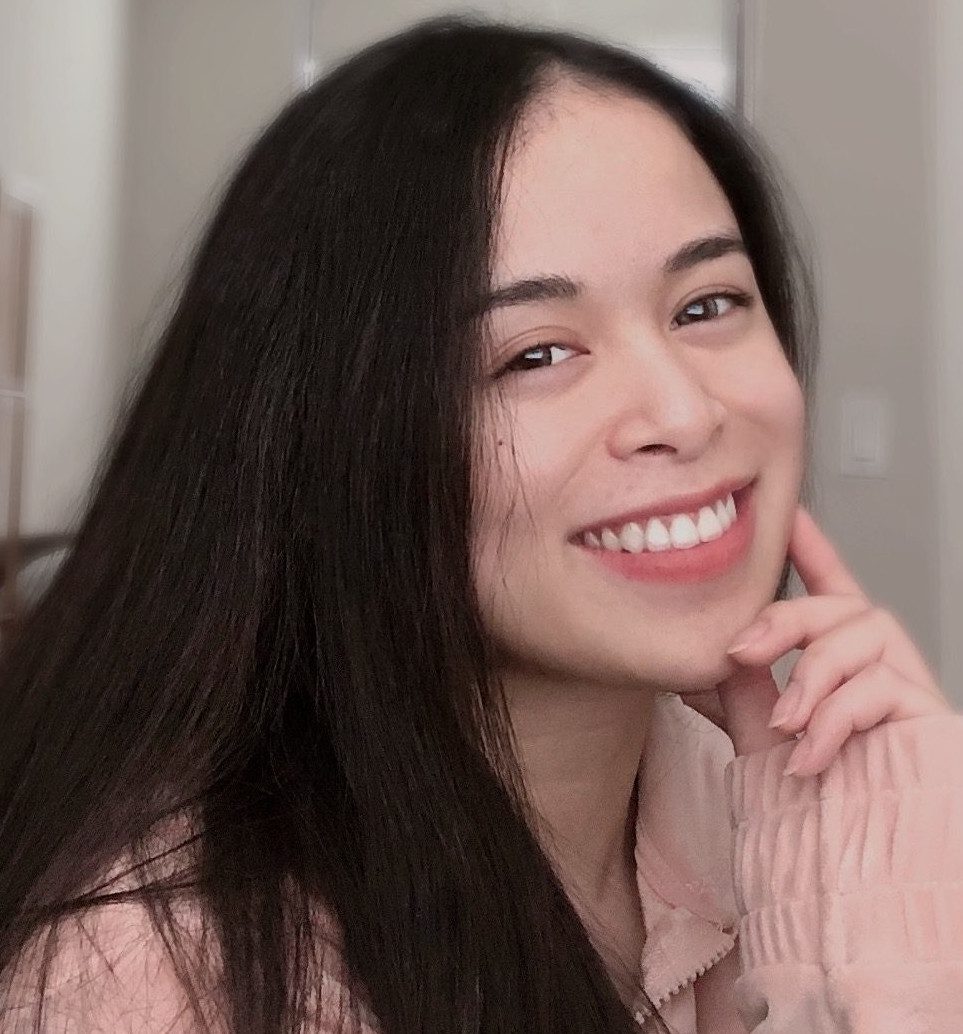
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
#include <iostream> #include <stack> #include <string> using namespace std; int main() { stack <string> tests; tests.push("Graham"); tests.push("Indra"); tests.push("Sophia"); tests.pop(); cout << tests.top(); }
Our code returns: Indra
.
This example is similar to the one that we used to add items from a list, but we have added in a new line of code after we use push()
to add all student names to the stack. This line of code uses pop()
to remove the test at the top of the stack.
When pop()
is executed, Sophia is removed from the stack—her name is at the top of the stack—and every other item in the list shuffles up one position. So, after running pop()
, Indra’s name moves to the top of the stack.
Next, we use top()
to retrieve the name at the top of the tests stack, and we print out that name to the console. Because we removed Sophia’s name using pop()
, Indra’s name is returned, which was the next one in the stack.
Retrieve an Item from a Stack
The top()
method is used to retrieve the element at the top of a stack.
Let’s return to the elementary school test example. Suppose we have just finished marking Indra’s test and we want to know which one is next on the list to mark. We could use the following code to retrieve the name of the student whose test should be marked next:
#include <iostream> #include <stack> #include <string> using namespace std; int main() { stack <string> tests; tests.push("Graham"); tests.push("Indra"); tests.push("Sophia"); tests.pop(); tests.pop(); cout << tests.top(); }
Our code returns: Indra
.
This code is similar to our example that we used to remove an element of the stack. In this example, we declare a stack called tests, then we add the names Graham, Indra, and Sophia to the stack. In this example, though, we use pop()
twice, and so we remove the top two names from our stack. In this case, the names Sophia and Indra are removed.
Finally, we use top()
to retrieve the item at the top of our stack. Because we removed Sophia and Indra’s names, Graham’s name has ascended to the top of the stack. So, when we use top()
to retrieve the name at the top of the stack, Graham’s name is returned.
Print a Stack
In C++, there is no method that can be used to print the contents of a stack. However, we can use a for loop to iterate through the contents of a stack in C++, which when used with other methods, allows us to print out the contents of a stack.
Suppose we have added three names to our stack of student tests: Graham, Indra, and Sophia. We want to print out a list of these names to the console, so we know all the students who have handed in a test to be marked. We could use the following code to accomplish this task:
#include <iostream> #include <stack> #include <string> using namespace std; int main() { stack <string> tests; tests.push("Graham"); tests.push("Indra"); tests.push("Sophia"); stack <string> new_tests = tests; cout << "Tests handed in:\n"; while (!new_tests.empty()) { cout << new_tests.top() << "\n"; new_tests.pop(); } }
Our code returns:
Tests handed in: Sophia Indra Graham
Let’s break down our code. First, we declare a stack called tests and we use push()
to add Graham, Indra, and Sophia to the stack.
We then declare a new stack called new_tests and assign it the same values that we have stored in the tests stack. This is an important step because, in order to access every item in our stack later in our code, we need to remove the top item. So, to avoid updating our initial stack, we have declared a new one.
On the next line, we print out a message stating Tests handed in:
to the console, followed by a new line character (\n
) which adds a blank line to the console.
We then initialize a while loop which runs until the expression !new_tests.empty()
evaluates to false. This expression evaluates whether or not new_tests is empty. Our while loop, when executed, performs the following steps:
- The student name at the top of the new_tests stack is retrieved using
top()
and is printed to the console, followed by a new line character. - The student name at the top of the new_tests stack is removed using
pop()
. This means that, when the while loop runs again, we can get the next item in the stack.
Using this code, you can print out the contents of a C++ stack.
Other Stack Methods
There are two additional methods used with stacks in C++ which you may encounter. These are contained in the reference table below:
Method | Description |
size() | Returns the size of the stack. |
empty() | Checks whether the stack is empty. This method returns true if the stack is empty and false if the stack is not empty. |
Conclusion
Stacks are used to store data in last-in, first-out order. Stacks are useful when you want to store data in an order where the most recent item added to the stack is the first one that should be removed from the stack.
This tutorial discussed, with reference to examples, the purpose of stacks in C++, how to create a stack, and how to add, remove, and retrieve items from a stack. Now you’re ready to start working with C++ stacks like a professional developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.