How to Use C++ Queues
In programming, queues are used to create data structures using the first-in, first-out (FIFO) approach.
When you’re programming in C++, you may decide that you want to store a particular set of values in a FIFO structure. For instance, if you’re creating an app that stores a list of orders at a coffee shop, you would want them to be stored in a FIFO order.
That’s where the C++ queue comes in. This tutorial will discuss the basics of queues, how to use queues in C++ to store data, and explore the main methods used to work with queues in C++. By the end of this tutorial, you’ll be an expert at using the C++ queue structure.
C++ Queues
Queues are a type of container adaptor in C++. They are used to implement a first-in, first-out (FIFO) data structure in programming. This structure means that the first item in a queue will be the first one on which an operation is performed.
Elements are inserted and stored in the FIFO order. For instance, if you want to remove an item from a queue, the first one to be removed will be the first one in the queue.
One example we could use to demonstrate the queue structure would be to think about shopping at a store. The first person who goes in line to check out will be the first one to be served; the second person in the queue will be served next, and so on.
To declare a queue in C++, we must use the queue package. This package allows us to use queues in our code. We can use the following code to include the queue package in our code:
#include <queue>
Now that we’ve imported queue into our code, we can start working with the queue data structure.
Declare a Queue in C++
Declaring a queue is another term used to describe the process of creating a queue. So, when you declare a C++ queue, you are telling your program that a queue should be created.
Here’s the syntax that is used to declare a queue in C++:
queue <dataType> queueName;
Let’s break this down. Here are the three main components in our queue:
- queue instructs our program to create a queue.
- dataType is the type of data that is to be stored in our queue.
- queueName is the name of our queue.
Suppose we are operating a coffee shop and we are building a program which keeps track of our orders. Our program should use a queue to store the names of customers who have ordered a coffee so our baristas know who to serve in what order.
We could use the following code to declare a queue to serve this purpose:
queue <string> pendingOrders;
In our code, we have declared a queue called pendingOrders
. This queue can store string values.
Add Item to a C++ Queue
C++ queues are used to store data, which means that there are a number of features we can use to manipulate the contents of a queue.
The push()
method is used to add an item to a C++ queue. push()
accepts one parameter, which is the value of the item you want to add to a specified queue.
Let’s return to the coffee shop example from earlier to demonstrate the push()
method in action. Suppose we have just opened our store and two customers have placed an order: Holly and Jim. Holly was first to place an order, then Jim placed his order.
We could use the following code to add Holly and Jim to our queue:
#include <iostream> #include <queue> #include <string> int main() { queue <string> pendingOrders; pendingOrders.push("Holly"); pendingOrders.push("Jim"); return 0; }
In this example, we have added Holly and Jim to our queue called pendingOrders
in that order. Our code returns 0, which tells us that our code has finished running. Let’s break down our code.
First, we import the queue and string libraries which are used to work with the queue data structure and string data type, respectively. We then use the queue method to create a queue called pendingOrders
. This queue is capable of storing string values.
Next we use the push()
method to add Holly’s name to our queue, and we use push()
to add Jim’s name to the queue on the next line. This means that Holly is first in our queue, and Jim is second in our queue.
Here’s what our queue looks like:
Item |
Holly |
Jim |
Remove Item from a C++ Queue
The pop()
method is used to remove an item from a queue in C++. The pop() function removes the item at the first position in the queues, because queues use the first-in, first-out data structure.
Suppose our barista has just processed Holly’s coffee order and wants to remove Holly’s name from the list of pending orders. We could use the following code to accomplish this task:
#include <iostream> #include <queue> #include <string> int main() { queue <string> pendingOrders; pendingOrders.push("Holly"); pendingOrders.push("Jim"); pendingOrders.pop(); return 0; }
In this example, we have added Holly and Jim to our queue called pendingOrders
. Then we used the pop()
method to remove the first item in our queue. Our code returns 0, to indicate that our program has finished running.
Here’s what our queue looks like now that we have removed Holly’s name from the queue:
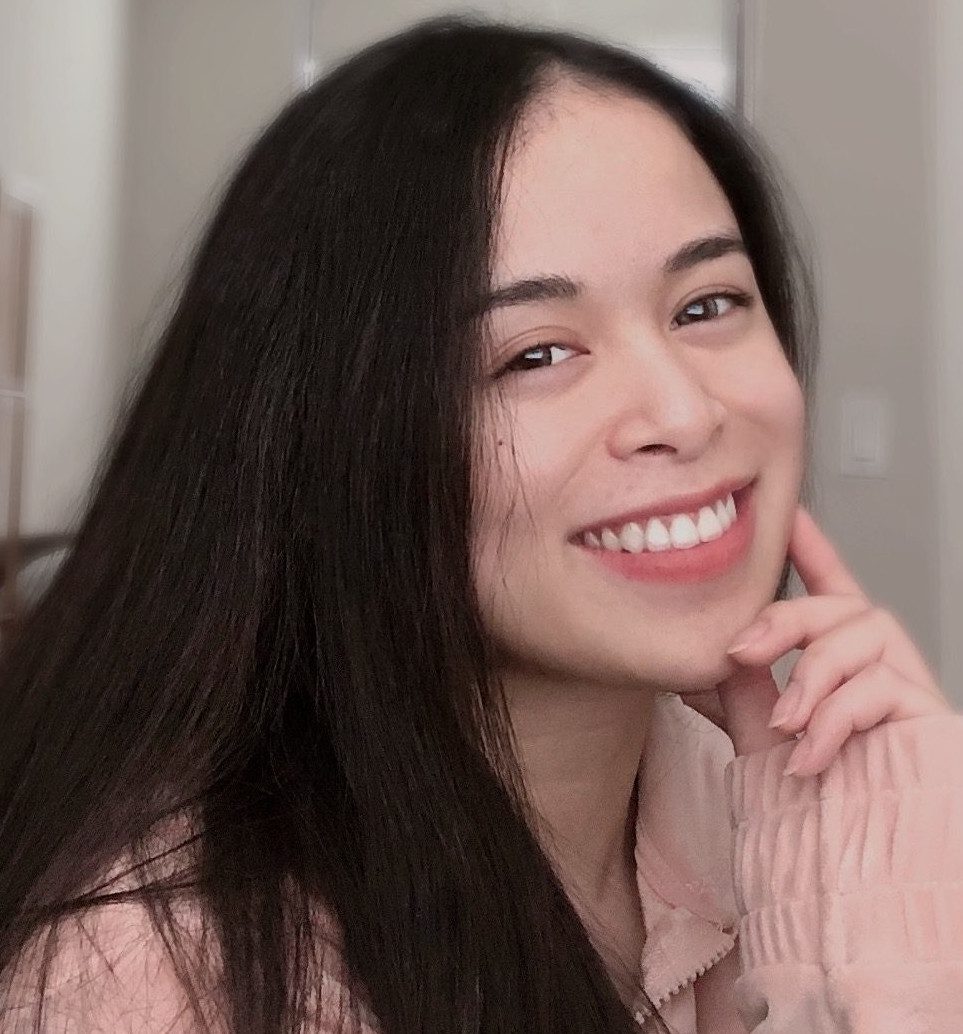
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Item |
Jim |
As you can see, our queue only has one item remaining, which is Jim’s name.
Retrieve Item from a C++ Queue
The front()
and back()
methods are used to retrieve the items at the front and the back of a queue in C++.
C++ Queue front
Suppose our barista wants to know the next order that is pending so they can start working on brewing the customer’s coffee. We could use the following code to find out the name of the next customer whose order is pending:
#include <iostream> #include <queue> #include <string> int main() { queue <string> pendingOrders; pendingOrders.push("Holly"); pendingOrders.push("Jim"); cout << pendingOrders.front() }
Our code returns: Holly
.
In our code, we first declare a queue called pendingOrders
and add the names Holly and Jim to the queue (in that order). We then use the front()
method to retrieve the item at the front of the queue, and we print it to the console using cout.
C++ Queue back
Let’s say that our barista wants to know the most recent order placed in our queue. This is the order at the back of the queue, because queues use the first-in, first-out structure. We could use the back()
method to retrieve the most recent order placed in the queue:
#include <iostream> #include <queue> #include <string> int main() { queue <string> pendingOrders; pendingOrders.push("Holly"); pendingOrders.push("Jim"); cout << pendingOrders.back() }
Our code returns: Jim
.
In this example, we have initialized a queue and assigned it the values Holly and Jim like we did in our example using the front()
method. But instead of using front()
to print the first item in our queue, we use back()
to print the last item in our queue. Because Jim was added last to our queue, his name was returned by the back()
method.
Print a C++ Queue
When you’re working with queues in C++, you may decide that you want to print the contents of a queue to the console. C++ does not include any specific method that is used to print a queue to the console, but we can use a while loop to print out the contents of a queue.
For instance, suppose we wanted to print out a list of our pending orders at the coffee shop so that our baristas know who to serve in what order. We could use the following code to accomplish this goal:
#include <iostream> #include <queue> #include <string> include namespace std; void showQueue(queue <string> pendingOrders) { queue <string> orders = pendingOrders; while (!orders.empty()) { cout << "\t" << orders.front(); orders.pop(); } cout << "\n"; } int main() { queue <string> pendingOrders; pendingOrders.push("Holly"); pendingOrders.push("Jim"); showQueue(pendingOrders); }
Our code returns:
Holly Jim
Let’s break down our code. In our example, we have declared a function called showQueue which accepts a queue called pendingOrders
as a parameter. This function creates a new queue called orders, then uses a while loop to print out every item in the queue.
The while loop uses the empty()
method to check if the orders queue is empty. !empty()
means that the while loop should run until the orders queue is empty. The while loop in our code executes the following steps:
- A new tab character (
\t
) is printed to the console, followed by the first item in the orders queue. This item is accessed using thefront()
method. - The first item in the orders queue is removed using
pop()
. This allows our while loop to retrieve the contents of the next item in our queue on its next iteration.
In our main program, we first create a queue called pendingOrders
, then we add Holly and Jim to our queue. Finally, we invoke the showQueue()
method which prints out the contents of the pendingOrders
queue that we specified as a parameter to showQueue()
.
Other Queue Methods
There are four additional C++ queue methods that you may want to use in your code. Here is a reference table with a brief description of each of these methods:
Method | Description |
size() | Returns the size of the queue. |
emplace() | Inserts new elements in the queue above the last element in the queue. |
swap() | Swaps the contents of two containers. |
empty() | Checks whether a queue is empty. Returns true if a queue is empty, and false if a queue contains one or more values. |
Conclusion
The queue structure is used in C++ to create queues. Queues leverage the first-in, first-out data structure, which means the first item in the queue is the first one which will leave the queue when an item is removed.
This tutorial discussed, with references to examples, the basics of queues in C++, and how to perform common operations on a C++ queue such as adding and removing array items using push and pop. Now you’re ready to start working with C++ queues like an expert developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.