If...else
is a conditional statement in C++. The C++ if
statement runs a block of code if a condition is met. An if...else
statement functions the same way but runs a second block of code if the condition is not met. If
and if...else
statements can be nested.
Conditional statements are an essential part of every programming language. Conditional statements allow developers to run code based on whether a particular condition is met in a program.
In C++, if
and if … else
statements evaluate whether a statement is true or false and only run a block of code if the statement evaluates to true.
This tutorial will discuss, using examples, the basics of C++ conditional statements and how to write if
, if … else
, and else if
statements in C++. By the end of this tutorial, you’ll be an expert at using these statements in C++.
C++ Conditional Statements
Developers use conditional statements to define the condition(s) on which a block of code will run. Whether a block of code in a conditional statement will run depends on whether a certain condition or set of conditions are met. Here are a few examples where conditional statements may be used:
- If a shopper has enough money in their account, a payment should be processed; if the shopper has insufficient funds, the payment should be declined.
- If a customer is over the age of 17, they should be allowed to see a movie; if not, they should be denied entry to the movie.
- If a bakery has less than 15 bags of flour in stock, a new case of flour should be ordered; if not, nothing should happen.
In these cases, a certain action should only be performed if a condition is met. That’s where if
and if … else
statements come in.
C++ if Statement
The C++ if
statement evaluates whether an expression is true or false. If the expression evaluates to true, the program executes the code in the conditional statement; otherwise, the program does not execute the code in the conditional statement.
Here’s the syntax for an if
statement:
if (expression) { // Code here }
Let’s walk through an example to illustrate how if
statements work in C++. Suppose we are writing a program that calculates whether a bakery should order a new case of flour. If there are fewer than 15 bags of flour in inventory, a new case should be ordered.
We could use the following code to accomplish this task:
#include <iostream> include namespace std; int main() { int flourBagCount = 12; if (flourBagCount < 15) { cout << "There are not enough bags of flour in inventory." } }
When we run this code, the following is printed to the console:
There are not enough bags of flour in inventory.
Let’s break down this example. First we declare a variable called flourBagCount
that stores the number of bags of flour we have in inventory. Then we initialize an if
statement that checks whether there are fewer than 15 bags of flour in inventory.
If the variable flourBagCount
is less than 15, the message There are not enough bags in inventory.
is printed to the console; otherwise, nothing happens. In this case, flourBagCount
is equal to 12, which is less than 15, so the program executes the code contained within the if
statement.
C++ if … else Statement
When you’re working with if
statements, you may want your program to do something besides move on if the statement evaluates to false.
if … else
statements, like if
statements, check for a condition. If that condition is met, the program executes the contents of the if
portion of the statement. However, unlike if
statements, in if … else
statements, if the condition is not met, the contents of the else statement execute.
Here’s the syntax for an if … else
statement:
if (expression) { // Run code } else { // Run other code }
Let’s take our bakery example from above. In our example, if there are fewer than 15 bags in stock, the program prints a message to the console telling the baker that there are not enough bags of flour in inventory. However, if there are enough bags of flour in inventory, nothing happens.
Suppose that we want a message to appear notifying the baker that there are enough bags of flour in inventory if there are 15 or more bags in stock. That’s where we could use an if … else
statement. The following code would tell the program to print a certain message to the console if there are enough bags of flour in inventory:
#include <iostream> include namespace std; int main() { int flourBagCount = 16; if (flourBagCount < 15) { cout << "There are not enough bags of flour in inventory." } else { cout << "There are enough bags of flour in inventory." } }
Our code returns:
There are enough bags of flour in inventory.
In this example, we changed the value of flourBagCount
to 16. We also added an else
statement to our code.
Because flourBagCount
is not less than 15, our if
statement evaluates to false. This means that our program executes the contents of our else
statement instead. As a result, our program prints the text, There are enough bags of flour in inventory.
to the console.
C++ allows for nested if statements, which is simply an if
or if...else
statement inside of another statement. However, often a cleaner way to handle this need is often else if
statements.
C++ else if Statement
We have already discussed how to use an if
statement to code a programmed response if a condition evaluates to true, and how to use an else
block to run certain code if a preceding if
statement evaluates to false. But what if we want to check for multiple conditions and execute a block of statements if any of those conditions are true?
To check for multiple conditions in a program, we need to use a nested else if
statement. Here’s the syntax of a nested else if
statement in C++:
if (expressionOne) { // Code to run if condition is true } else if (expressionTwo) { // Code to run if condition is false and expressionTwo is true } else { // Code to run if all test expressions are false }
Let’s walk through an example to discuss how the else if
statement works.
Suppose we want to add a message to our bakery program from earlier that notifies our baker when the number of bags of flour in stock is equal to or greater than 15 and is equal to or less than 20. We want our program to do the following:
- If there are over 20 bags of flour in stock, the program should print out a message that says,
There are enough bags of flour in inventory.
- If there are between 15 and 20 bags of flour in stock, the program should print out a message that says,
A new order for flour should be placed soon.
- If there are fewer than 15 bags of flour in inventory, the program should print out a message that says,
There are not enough bags of flour in inventory.
We could use the following code for this program:
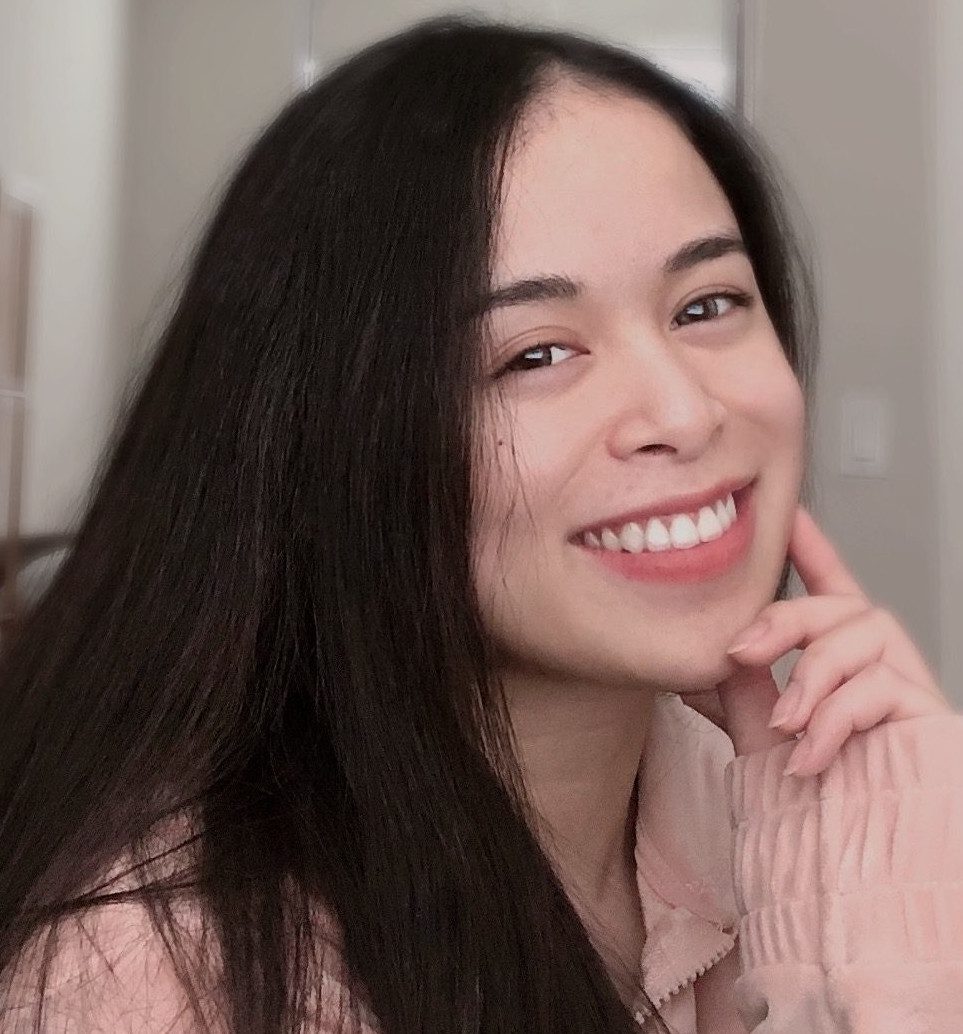
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
#include <iostream> include namespace std; int main() { int flourBagCount = 16; if (flourBagCount > 20) { cout << "There are enough bags of flour in inventory." } else if (flourBagCount <= 20 && flourBagCount >= 15) { cout << "A new order for flour should be placed soon." } else { cout << "There are not enough bags of flour in inventory." } }
Our code returns:
A new order for flour should be placed soon.
In this example, our program checks for two conditions and includes an else
statement that executes if those two conditions both evaluate to false.
In the first conditional statement in the above code, our program checks to see if the value of flourBagCount
is over 20. If it is, the message There are enough bags of flour in inventory.
is printed to the console.
If this statement evaluates to false, the else if
statement is evaluated. In this example, the else if
statement checks to see whether the value of flourBagCount
is equal to or less than 20 and equal to or greater than 15. If both these statements are true, the message A new order for flour should be placed soon.
is printed to the console. This was the case–and outcome–of the above example.
If both of the if
statements in our code (if
and else if
) evaluate to false, our program executes the code in the else
statement. If this is the case, our program prints out the message, There are not enough bags of flour in inventory.
Conclusion
You can use if
and if … else
statements to control the flow of a program in C++.
if
statements evaluate whether a condition is true and execute certain code if so. else if
statements evaluate whether a second condition is true and execute certain code if so. else
statements execute certain code if none of the specified conditions in an if
statement evaluate to true.
This tutorial discussed, with examples, how to use if
, if … else
, and else if
statements in C++. Now you’re ready to start using these C++ conditional statements like a professional developer.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.