C++ is a powerful, general-purpose programming language that was developed by Danish computer scientist Bjarne Stroustrup. It is used for creating operating systems, web browsers, video games, and machine learning tools. Completing C++ exercises is a good way to begin learning this high-level programming language because you’ll get a strong understanding of the basics.
If you’re wondering, “Where can I practice C++?” the answer lies below. There are many C++ tutorials and quizzes you can check out, plus forums where you can get help with C++ from experienced coders.
C++ Exercises to Help You Learn C++
C++ exercises are an excellent tool for programming practice to build your skills and expand your knowledge of the language. Through exercises, you will learn C++ by exploring its syntax, variables, flow control, vectors, and classes. You will also familiarize yourself with other C++ tools like its text editor, linker, C++ compiler, and the comprehensive library that C++ offers.
10 C++ Exercises and Practice Problems (With Solutions)
1. Hello World
Hello World is an introductory C++ program designed to get beginners familiar with the basics of C++ syntax. The goal is to write code that will display the words “Hello World” on the computer screen.
Solution: The final answer should include the output “cout” to send the message “Hello World.” In C++, cout identifies the standard output device, in this case, the computer display screen.
2. Multiply Two Floating Point Numbers
Floating point numbers refer to positive or negative whole numbers with a decimal point, like 5.5 or 2.1. They often represent non-integer fractional numbers and are common in tech calculations. In this exercise, you will enter two numbers and store them in variables num1 and num2. Then, when the product is evaluated, the solution is also stored and displayed on the screen as a variable product.
Solution: For example, if the two floating numbers are A= 2.12 and B= 3.88, the product for A and B is 8.2256.
3. Insert Missing Special Characters
C++ comments are written using special characters to make the source codes readable and help in debugging specific code snippets. This exercise requires you to include symbols for a single-line comment and a multi-line comment.
Solution: In C++, single line comments uses // and multi-line comments use /* code */.
4. Display the Sum of Two Numbers Using Variables
Variables are an essential part of C++. You can use variables to store the values in a C++ program, then change the values during execution. This simple exercise instructs you to display the sum of two numbers using the variables X and Y to test your understanding of C++ characters and variables.
Solution: Your answers should follow some essential C++ variable rules. First, C++ variables are case-sensitive and use lowercase letters only. Second, they don’t include any spaces or special characters.
5. Reverse an Array
Reversing an array is a common exercise in programming. Sometimes, when you need to process arrays starting with the last element, you should reverse the array so that the first and last elements change positions. The same switch also applies to the elements in between the ends of the arrays. Keep in mind the array size and adjust accordingly if needed.
Solution: You can reverse an array using a for loop, reverse function, user-defined function, pointers, or a recursion function. After selecting your reversal method, you can enter all your elements in order and initialize the reversal.
6. Determining Eligibility of Admission
In this exercise, you will write a program that checks admission eligibility for an engineering course. The program accepts two integers and analyzes the patterns to determine whether the elements are equal. If the results of the integer range match, it means the engineering candidate fits the criteria.
Solution: The program will include the name and age of the candidate and the minimum age required. If the candidate is underage, the program will reject the application. It will then repeat the process until it finds the right candidate. If you’re running into issues, you can use any C++ compiler to debug your program.
7. Create a Bank Account
This exercise involves writing a bank account program using C++. Your program should display the depositor’s name, account number, account type, and balance. You must also feature functions that display the name, allow the withdrawal, assign the initial values, and deposit the requested amount.
Solution: The correct answer will include the right variables, classes, objects, clear comments, and relevant member functions so the bank program will run correctly.
8. Find the Length of a Number
Numbers are everywhere in programming, and finding the length of a number is crucial if you want to avoid inaccurate results. This exercise puts your skills to the test as you write a program that measures the length of a number in C++.
Solution: Start by declaring your variables, then input the number. Once you run the program, you should come up with the right result.
9. Convert Feet to Meters
One foot is equivalent to 0.3048 meters. In this exercise, you will create a program in C++ that converts feet to meters. This is a straightforward exercise for beginners that uses basic variables and functions.
Solution: Use the variable data type for the float, cout, and cin for your basic input and output, then run the conversion. Remember that using the most accurate figures possible in your version will work better than using approximations.
10. Find Total Number of Days in a Month
While coding, sometimes you will need to work with dates, so this exercise is good practice. It requires you to develop a program that will tell you how many days are in any given month.
Solution: This program uses function design, variables, and correct values. You will also input the month and year as the arguments and run your program to come up with the right number of days.
How to Get Help with C++
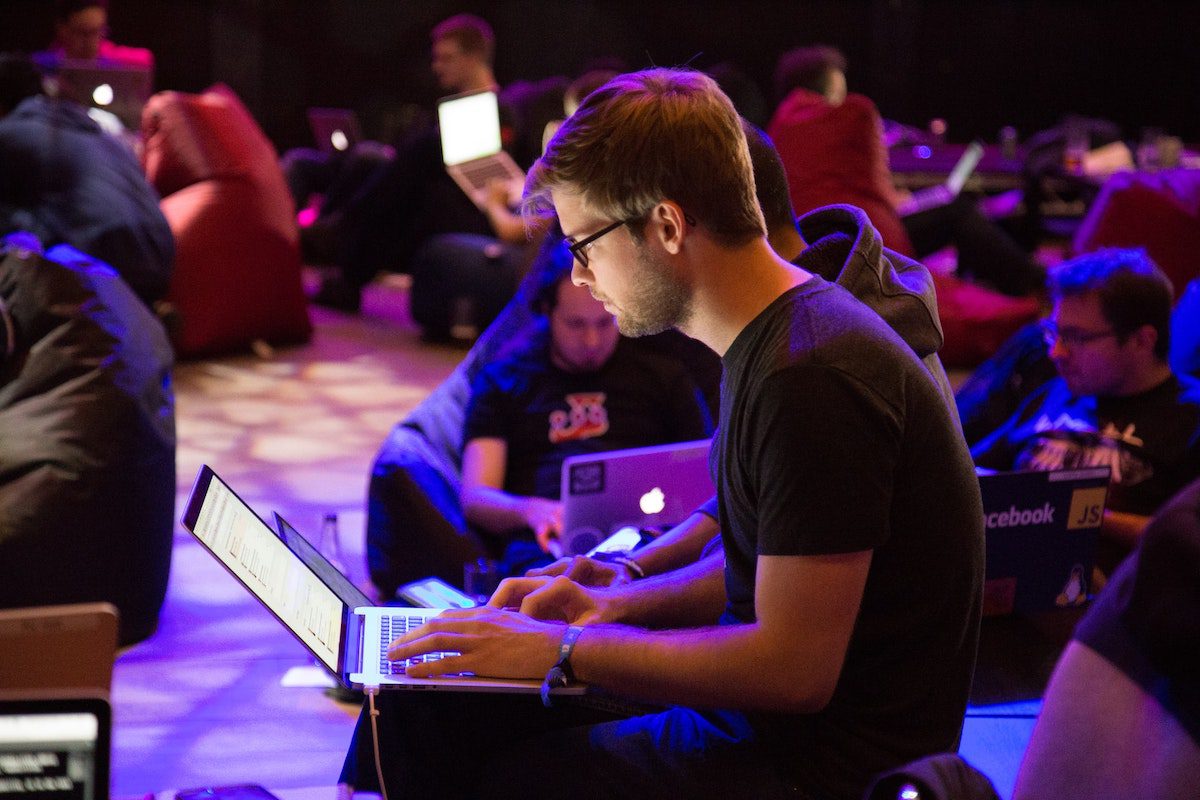
Whether you’re a beginner or seasoned professional, you will need help with at least some aspects of the C++ programming language. Below are the main resources you can turn to when you need help with C++.
C++ Exercises
C++ exercises are practical problems that you can solve using your C++ coding skills. These exercises explore C++ topics like control statements, objects, inheritance, interfaces, and strings. The exercises also come with a sample solution so you can make sure you came up with the right answer.
To find C++ coding exercises, you can look on popular C++ websites, a popular coding book, or on C++ YouTube channels. In addition, joining a C++ online community will give you even more access to code examples and exercises.
C++ Projects
C++ projects are more complex than exercises, requiring you to essentially solve a series of problems. Projects are important because they offer hands-on experience that you can apply directly to real-world projects in your job. Moreover, in projects, you will get to practice more broad skills such as database programming, software development, and system configuration along with your C++ skills.
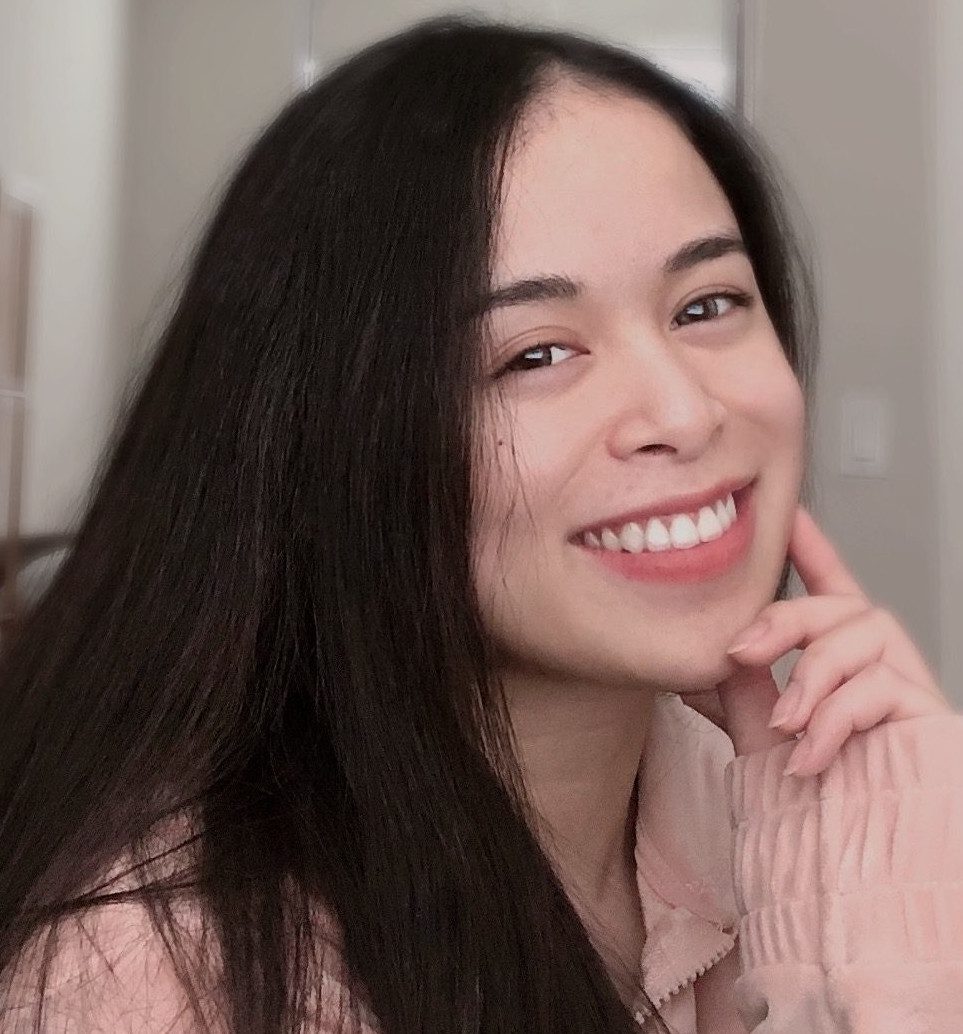
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Popular C++ project ideas include building a bank management system, a stopwatch, or a credit card validator. Once you’re comfortable with small projects, you can advance to projects like developing a Sudoku game, a car rental system, or a bookshop inventory system. You can find all of these projects on online C++ platforms or forums.
C++ Quizzes
C++ quizzes are usually multiple-choice questions that assess your knowledge of C++. The questions cover basic C++ topics then advance to more difficult topics as the quiz progresses. Topics include object oriented programming principles, data types in C++, writing a C++ program, class constructs, and C++ libraries.
C++ Forums and Blogs
C++ forums and blogs are communities where C++ enthusiasts share ideas and discuss the intricacies of the code. Forums are also a great place to network. You can even share your projects in a forum, allowing the community members to contribute to or review your work.
Reading C++ blog posts is a great way to compare your experiences with programming and gain insight from fellow developers. You may even want to start your own C++ blog so you can reflect on your learning process and receive feedback from readers. Popular C++ blogs and forums include C++ Tips of the Week, Learn C++ by Yilmaz Yorum, the C++ Forum on Reddit, and CodeGuru Forums.
Where Can I Practice C++?
You can practice C++ on forums and blogs, by watching video tutorials, or by visiting C++ exercise websites. However, you’ll need to beware of using bad programming resources that will give you incorrect information. Since every single digit in each arithmetical operation has to be precise, you need to make sure you’ve got your ducks in a row.
Community-sourced C++ exercises could be useful, but the complexity of code should be on par with your level of understanding until you’re ready to advance. Below are five of the best platforms that you can use to practice your C++ skills.
Websites to Practice C++
- Project Euler. Project Euler features a series of C++ problems. The website is home to over 600 problems covering different C++ topics. However, not all the problems have defined solutions, so you’ll have to come up with your own.
- W3resource. This website features several introductions to programming in C++ exercises with solutions. The exercises will make use of all your C++ knowledge. You’ll practice using C++ for math, loops, strings, and more.
- Edabit. Edabit is a fun platform for learning C++ from scratch. It features tutorials, challenges, and practice questions that range from very easy to expert-level.
- HackerRank. HackerRank is one of the leading technical assessment platforms for developers. On it, you will find several C++ practice exercises that gauge your knowledge of C++ strings, functions, variable-sized arrays, pointers, and operators.
- Exercism. Exercism offers C++ exercises, solutions, and personal mentoring to help you understand C++ fundamentals and how to apply the programming language.
What’s the Best Way to Learn C++?
Attending one of the best C++ coding bootcamps is the ideal way to learn this programming language. These rigorous bootcamp courses focus on C++, including applying it in a hands-on, practical way. You will work on real-world projects and gain skills that you’ll use in your career after graduation. Plus, since C++ is a complex language, you’ll be in high demand once you enter the job market.
C++ Exercises FAQ
Yes. C++ can be hard to learn for beginners. Getting familiar with programming languages like C, C#, or Java before you learn C++ can help. However, once you have mastered C++, you’ll be able to easily pick up other similar codes, like Go.
First, remember that you can’t rush the learning process for C++. It is a complex language and you must understand every step of its operations before you can use it. You should start by installing a C++ compiler, choosing a C++ online course or bootcamp to attend, then get to work on C++ fundamentals. Then, you can practice your C++ skills by completing projects and exercises.
It can take anywhere from three months to two years to learn C++, depending on your experience level and commitment. Your preferred learning path will also determine the time frame. For example, coding bootcamps offer intensive courses that take only a matter of months to complete, whereas computer programming bachelor’s degrees take years to complete.
Yes, learning C++ is with it because of its reliability, portability, speed, and efficient memory management. Moreover, once you’ve grasped C++ concepts, you can learn other advanced languages. Lastly, having C++ skills can help unlock lucrative careers, including as a quality assurance analyst, video game programmer, security solutions architect, or software engineer.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.