How to Use C++ Enum: A Guide
An enumerated type is a user-defined data type which can be assigned one value from a range of values.
Enums are often used in programming when a variable should only be able to store one value out of a specific set of values. For example, if you wanted a variable to only store days of the week, you may want to use an enumeration.
This tutorial will explore, with reference to examples, the basics of enumerations in C++, how to define an enumeration, and how to use an enumeration in your code. By the end of reading this tutorial, you’ll be an expert at using enums in C++.
C++ Enum
The enumerated type, also known as the enum type, is used to create a custom data type that has a fixed range of possible values. Enumeration is a user-defined data type.
To define an enum in C++, the enum keyword is used. Here’s the syntax for defining an enum in C++:
enum name { firstValue, secondValue, thirdValue, fourthValue };
Let’s break down this syntax into its main components:
- enum instructs our code to create an enumeration.
- name is the name assigned to our enumeration.
- firstValue, secondValue, thirdValue, and fourthValue are the values assigned to our enumeration. These values are enclosed within a set of curly brackets ({}).
In addition, you can also change the default value of an enum class. So, if you wanted a value to have its own name, you could do so by assigning its entry in the enumeration a new default value. Here’s the code we could use to accomplish this task:
enum name { firstValue = 0, secondValue = 1 }
In this syntax, our enum contains two values. The firstValue value has the value 0, and the secondValue value has the value 1.
Enums are useful because they allow you to more clearly define the potential values a variable can hold. For instance, if you only want a variable to store days of the week, declaring an enum makes it clear in your code that the variable should only be able to store days of the week.
Declare an Enum
Suppose we are creating an application which allows a cashier at a donut shop to record each order that has been placed and calculate the cost of the donut.
Our shop only sells a limited range of donuts, and so we only want the cashier to be able to record orders for the donuts in our inventory. The donuts we want our program to process are: Raspberry, Strawberry, Powdered, Chocolate, and Cinnamon.
This is a perfect example of where an enum could be useful. We want to limit our program to be able to process orders in the aforementioned flavors, and so using an enum is a good idea. Here’s the code we could use to declare a C++ enum:
enum flavors { Raspberry, Strawberry, Powdered, Chocolate, Cinnamon }
In our code, we declare an enum called flavors which has five potential values. So, when we try to assign a variable a value from this enum, the only values the variable will be able to store are those which are declared in our enum.
If we want to declare a variable that can only hold values from our flavors enum, we can use this syntax:
enum flavors orderFlavor.
The variable orderFlavor we have just declared can only store values in the flavors enum.
Using an Enum
Now that we have performed an enum declaration, we can use it in our code. Let’s return to the donut store example to illustrate how we can use an enum in a C++ program.
Suppose we are building a program that allows our donut store cashier to record each order. The cashier has just received an order for a raspberry donut, and we want to create an order for a raspberry donut in our program. We could do so using this code:
#include <iostream> using namespace std; enum flavors { Raspberry, Strawberry, Powdered, Chocolate, Cinnamon } int main() { enum flavors orderFlavor; orderFlavor = Raspberry; cout << "Donut ordered: 1x " << orderFlavor; }
Our code returns:
Donut ordered: 1x Raspberry
Let’s break down our code. First, we have declared an enum called flavors which stores the flavors sold at the donut shop.
Then, we declare a variable called orderFlavor which keeps track of the type of donut ordered by a customer. This variable can only store values in the flavors enum. On the next line of code, we assign the orderFlavor enum variable the value Raspberry.
Next, we print out Donut ordered: 1x
to the console, followed by the value stored in the orderFlavor variable.
In this example, you can see that using an enum makes our code easier to read. By using an enum, it is clear that the orderFlavor variable can only store values in the flavors enum. If we were working on a more complex program, the benefits of using enums would become even more clear, as there would be more variables for which we would need to account.
Changing Default Enum Values
In addition, we can also assign enums a particular default value. For instance, suppose we wanted to build a program that returns the price of a particular donut. Because there are a limited range of donuts a customer can order, we want to use an enum.
We could use the following code to create an enum that stores both our donut flavors and their prices:
#include <iostream> using namespace std; enum prices { Raspberry = 2.00, Strawberry = 2.00, Powdered = 1.75, Chocolate = 1.90, Cinnamon = 2.20 } int main() { enum prices orderPrice; orderPrice = Raspberry; cout << "Donut price: $" << orderPrice; }
Our code returns:
Donut price: $2.00
In this example, we have assigned each value in our enum a default value.
This means that, when we reference a value in our enum, its default value will appear instead of the name of the value. So, when we reference Chocolate, 1.90 will be returned; when we reference Cinnamon, 2.20 will be returned.
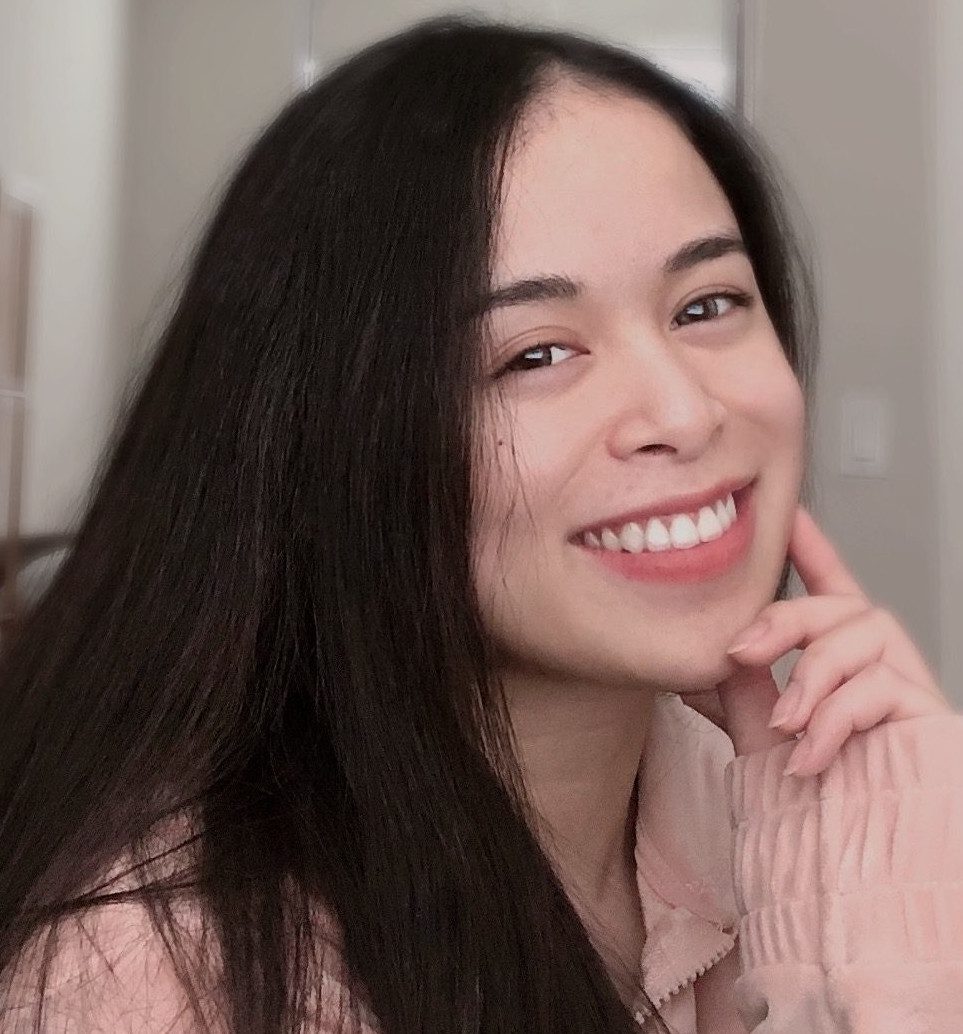
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
In our main program, we first declare a variable called orderPrice which uses the prices enum to restrict its possible values. Then we assign the orderPrice variable the value associated with Raspberry in our prices enum.
On the next line, we print the statement Donut price: $
to the console, followed by the price stored in the orderPrice variable. In this case, the customer ordered a raspberry donut, which costs $2.00, So, our code returned Donut price: $2.00
Conclusion
The enum data type is used to create a type of data that can only store a fixed set of values. For instance, an enumeration that stores seasons would only store spring, summer, autumn, and winter. Enums are useful when a variable should only be capable of storing one value that exists in a specific set of values.
This tutorial discussed, with reference to examples, how to create an enum in C++, how to use an enum, and how to change the default values stored by an enum. Now you have the know-how to start using enums in C++!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.