How to Use “while” Loops in C++
In programming, you can automate repetitive tasks with loops. Loops are an important part of programming because they allow developers to reduce code repetition. This reduction helps make code more efficient and easier to read.
while
and do … while
loops are two of the three main types of loops in C++. while and do … while loops execute a block of code as long as a specific condition evaluates to true; otherwise, the loop will stop executing and the program will move past the while block.
This tutorial will discuss, with examples, the basics of loops in C++ and how to use while and do … while loops in your code. By the end of reading this guide, you’ll be an expert at using while and do … while loops in C++.
C++ Loops
Loops execute a specific block of code based on whether a condition is met. In C++, there are three types of loops:
- for loops
- while loops
- do … while loops
for
loops execute a block of code until a test expression evaluates to false. For instance, a for loop may print out every number in the range of 1 and 10 or calculate the power of every number between 19 and 27.
while and do … while loops, on the other hand, allow you to execute a block of code while a condition evaluates to true. This means you don’t need to know how many times the loop should run before you create the loop.
C++ while Loop
The C++ while loop executes as long as a specific statement evaluates to true. The syntax for a while loop is almost exactly the same as that of an if
statement; the only difference is that the while
keyword is used instead of if
.
Here’s the syntax for a while loop in C++:
while (expression) { // Run code while condition is true }
Here is how a while loop works in C++:
- The program evaluates the stated expression in the while loop.
- If the expression is equal to true, the program executes the code in the while loop body.
- The program then evaluates the expression again.
- If the expression is still equal to true, the program executes the code in the while loop body again.
- If the expression evaluates to false, the program terminates the while loop.
- This process continues until the expression evaluates to false and the program terminates the while loop.
Let’s walk through an example to demonstrate how the C++ while loop works in a program.
Suppose we are operating a local superstore and are looking to hire 10 seasonal workers to help us with the Christmas rush.
Every time our loop executes, we want to add a new employee to a counter that keeps track of how many seasonal workers we have on staff. Once we have 10 seasonal workers, the program should stop running the while loop.
Here’s the code we can use to accomplish this task:
#include <iostream> include namespace std; int main() { int workersToHire = 10, workersHired = 0; while (workersHired < workersToHire) { ++workersHired; int leftToHire = workersToHire - workersHired; cout << "There are " << workersToHire << " workers left to hire." << std:endl; } }
Our code returns:
There are 9 workers left to hire. There are 8 workers left to hire. There are 7 workers left to hire. There are 6 workers left to hire. There are 5 workers left to hire. There are 4 workers left to hire. There are 3 workers left to hire. There are 2 workers left to hire. There are 1 workers left to hire. There are 0 workers left to hire.
Let’s walk through our code. First, we declare a variable called workersToHire. This variable stores how many workers we want to hire. We also declare a variable called workersHired. This variable keeps track of how many seasonal workers we have already hired.
On the next line, we initialize a while loop that runs while the value of workersToHire is greater than workersHired. So, the loop will run until workersHired is equal to workersToHire.
In our while loop, we use the ++ increment operator to add 1 to the value of workersHired. We then declare a variable called leftToHire that calculates how many employees we can hire until we reach our capacity. Finally, we print out the message There are X workers left to hire. to the console, where X is equal to the value stored in leftToHire.
C++ do … while Loop
So far, we have discussed how the while loop is used. while loops execute a block of statements as long as a condition is checked and evaluates to true.
do … while loops are a form of the while loop but with one difference: do … while loops execute once, after which the program evaluates a specified condition. Here’s the syntax for a C++ do while loop:
do { // Run code } while (expression);
Above you can see that the do statement is first. The while statement, which accepts a test expression, follows the do statement. Here is how a do … while loop works:
- The program executes the code within the do block.
- The program evaluates the expression within the while statement.
- If the specified expression evaluates to true, the loop executes again.
- If the specified expression evaluates to false, the program terminates the loop.
Let’s walk through an example to explain in more depth how the do … while loop condition works.
Example of do … while
Suppose we are building a guessing game that allows a user to guess a number between 1 and 10. If the user guesses the right number, the program should print a message to the console congratulating them; otherwise, the user should be allowed to guess again.
If the user does not guess the right number in three guesses, the program should print a message to the console informing the user that they lost the game.
Here’s the code we can use to create our guessing game:
#include <iostream> include namespace std; int main() { int numberToGuess = 7, guessesLeft = 3, guessed = 0; bool correct = false; do { cout<<"Enter a number between 1 and 10: "; cin>>guessed; guessesLeft = guessesLeft - 1; if (guessed == numberToGuess) { correct = true; break; } } while (guessesLeft > 0); if (correct == true) { cout<<"Congrats! You guessed the right number."; } else { cout<<"You lost! You didn't guess the right number in three guesses."; } }
This is the result of our code after guessing three numbers incorrectly:
Enter a number between 1 and 10: 1 Enter a number between 1 and 10: 9 Enter a number between 1 and 10: 2 You lost! You didn't guess the right number in three guesses.
When we guess the right number, the program returns the following response:
Enter a number between 1 and 10: 7 Congrats! You guessed the right number.
Let’s break down our code. First we declare four variables that we need to build our guessing game:
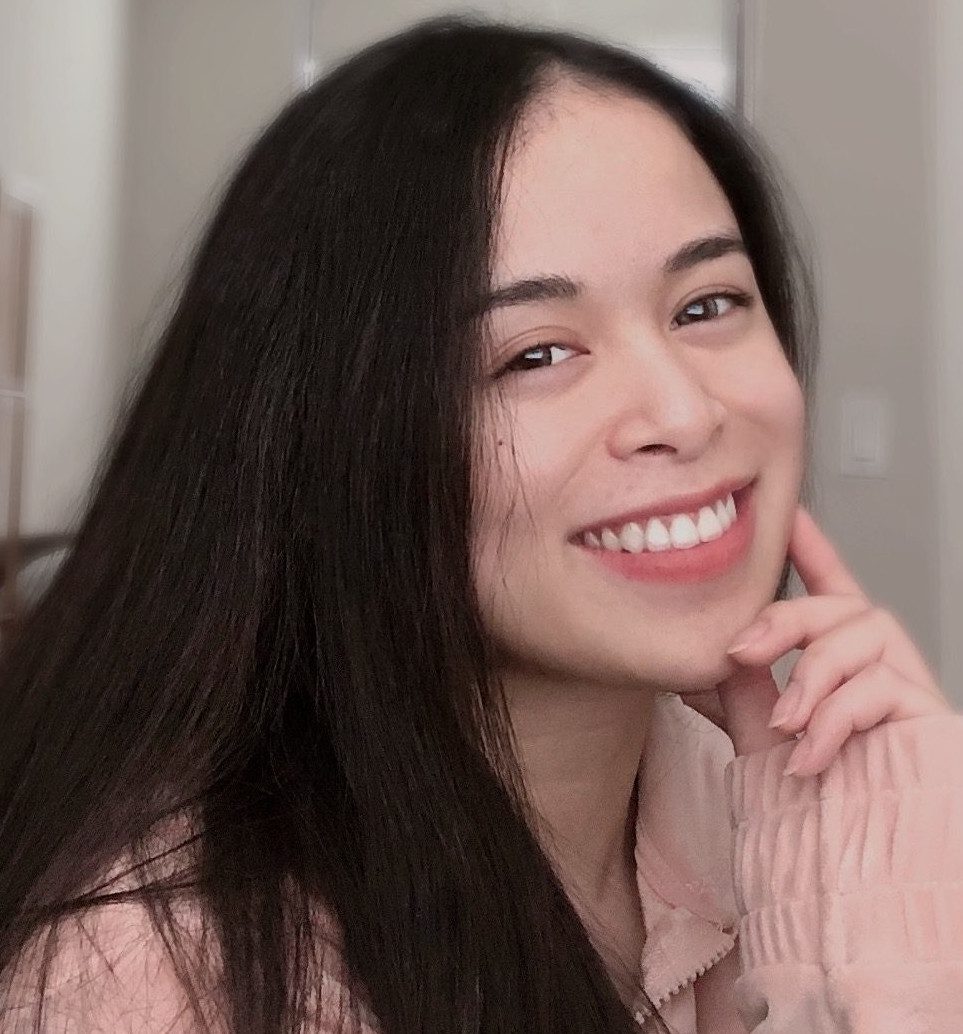
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
- numberToGuess is the number the player needs to guess. In this case, numberToGuess is equal to 7.
- guessesLeft is the number of guesses the player has remaining. This is set to 3 in our code.
- guessed is the number the user has guessed. By default, we set this to 0.
- correct keeps track of whether the user has guessed the right number. This is set to false by default and will only change to true if the user guesses the right number;
Then we use a do … while loop to create our guessing game mechanism. The program executes the contents of the do statement once, before it evaluates any condition. Our do statement asks a user to enter a number, assigns the number the user guesses to the variable guessed, and reduces the number of guesses a user has left by 1.
If the number the user enters is equal to numberToGuess, the variable correct is set to true and a break statement stops the do … while loop from executing again.
Then, our program evaluates whether guessesLeft is less than 0 in the while statement. If the user has more than 0 guesses left, the program will execute the contents of the do statement again; otherwise, the program will stop executing the do loop and will continue running the main program.
We also specified an if statement that checks whether the user guessed the correct number. If the user guessed the correct number, the program prints the message Congrats! You guessed the right number. to the console; otherwise, the program prints the message You lost! You didn’t guess the right number in three guesses. to the console, after the user has had three unsuccessful guesses.
Using a do … while loop is effective in this example because we want to give the user a chance to guess once before we check how many guesses they have left. The do … while loop allows our user to guess once, after which the loop checks whether they have any more guesses left.
Conclusion
In C++, while loops execute as long as a specific condition evaluates to true. do … while loops execute once first, after which the program determines whether a specific condition evaluates to true. If the condition evaluates to true, the loop runs again; otherwise, the do … while loop terminates.
This tutorial explored, with examples, the basics of loops in C++ and how you can use while and do … while loops in your code. Now you’re equipped with the knowledge you need to start using while and do … while loops like a C++ pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.