Dennis Ritchie, the father of C, famously said that the only way to learn a new programming language is to practice it. He’s not wrong, practice does make perfect. This is why we’ve compiled a list of the best C exercises with solutions you can rely on to get help with C.
If you’re wondering where you can practice C, put your knowledge to the test and try out any of the exercises we’ve listed below. We’ve also made a list of some of the best websites where you can practice C assignments that come with solutions. You’ll hopefully gain a deeper understanding of C and build the skills you need to become a strong C developer.
C Exercises to Help You Learn C
According to edX, C is perfect for learning the basics of computers and software development. The following are C programming exercises with solutions that can guide you to learning C. They include basic, yet very technical programming exercises. Take some time with them and follow the solutions carefully until you have gained mastery of this language.
10 C Exercises and Practice Problems (With Solutions)
1. Hello World
The Hello World program is the simplest program exercise in C. All you need to do is write a program that displays the words “Hello World!” on your screen. You’ll need to know how to use C functions to complete it.
Solution: Use the print function to display a request for the basic salary and the scan function to record that input. Then repeat the same process for the HRA and DA. Finally, find their sum and display the result.
2. Adding Two Numbers
Write a program to input two numbers, sum them together, and display the result. To complete this exercise, you’ll need to know how to use different data types, especially integer data types, and programming operators in C.
Solution: Create and name two variables to store your integers. Use the addition operator to add them together and store the result in another variable called sum. Lastly, use the print function to display the result.
3. Find the Gross Salary of an Employee
Write a program that inputs the basic salary of an employee and returns their gross salary as output. This exercise tests your proficiency in receiving input, displaying output, and using programming operators.
Solution: Use the print function to display a request for the basic salary and the scan function to record that input. Repeat the same process for the HRA and DA. Find their sum and display the result.
4. Check Leap Year
Write a program that checks if the input term is a leap year. This exercise tests your control statement knowledge, specifically on using a conditional statement.
Solution: Since leap years are divisible by 400, it is a leap year if the input data is divisible by 400. It is a common year if the input data is not divisible by 400 but is divisible by 100. If the input data is not divisible by 100 but is divisible by four, print it as a leap year. In all other cases, print that they are common years.
5. C Program to Check Whether a Character is a Vowel or Not
Write a program to receive input data and determine whether it is a character vowel or consonant. This exercise tests your knowledge of basic data types, the if/else function, and programming operators.
Solution: Using the if/else function and the equal-to operator, check if the input term is a vowel. C is a case-sensitive language, so pay close attention to the character form and check for both uppercase and lowercase letters.
6. C Program to Find the Largest Element of an Array
An array is a variable that can store several similar elements and assign each an array position. Write a program to input the array elements, determine the largest, and display it. To complete this exercise, you’ll need to have an understanding of array elements and the use of “For loops.”
Solution: Let the largest element (we’ll call it max) be the first element of the array. Create a For loop and compare max with the remaining elements of the array. If any element is larger than max, switch them and continue the process. At the end of the process, max will be the largest element in the array.
7. Calculate Simple Interest
Write a program to input loan information about interest, principal, and time, then calculate simple interest. This exercise tests your skill with data types and operators.
Solution: Create variables to input loan information. Create a variable P to input principle, I to input interest rate, and T to input time. The formula for simple interest is P×R×T/100. Express it using operators and print the result.
8. Reverse a Sentence Using Recursion
Write a program that can accept a sentence as its basic input and return it reversed as output. To complete this exercise, you’ll need to create user-defined functions and use the recursion function.
Solution: Create a user-defined function called “reverse” to store the input sentence. Apply the recursion function and call back the reverse function. This function will continue to run until the user hits enter. When they do, the reverse function will print the characters starting with the last.
9. Find the Frequency of Characters in a String
Write a program to analyze an input string and determine the frequency with which a particular character appears in it. You’ll need to understand character strings and arrays to complete this exercise.
Solution: Record an input of the key character and store it in a variable. Use the “For loop” to run over each character in the string. Every time it encounters the key character, it increases the frequency count by one.
10. Print the Smallest Value in an Array
Write a program that will accept input values from the user, store it in an array, and identify the smallest value in that array. You’ll need to know arrays and the “For loop” to execute this successfully.
Solution: Let the first address in your array be your minimum value. Then, use the “For loop” function to run over every element in the array. If you encounter an array value less than the one stored in the first address, replace it. The first address will hold the smallest array value when the loop ends.
How to Get Help with C
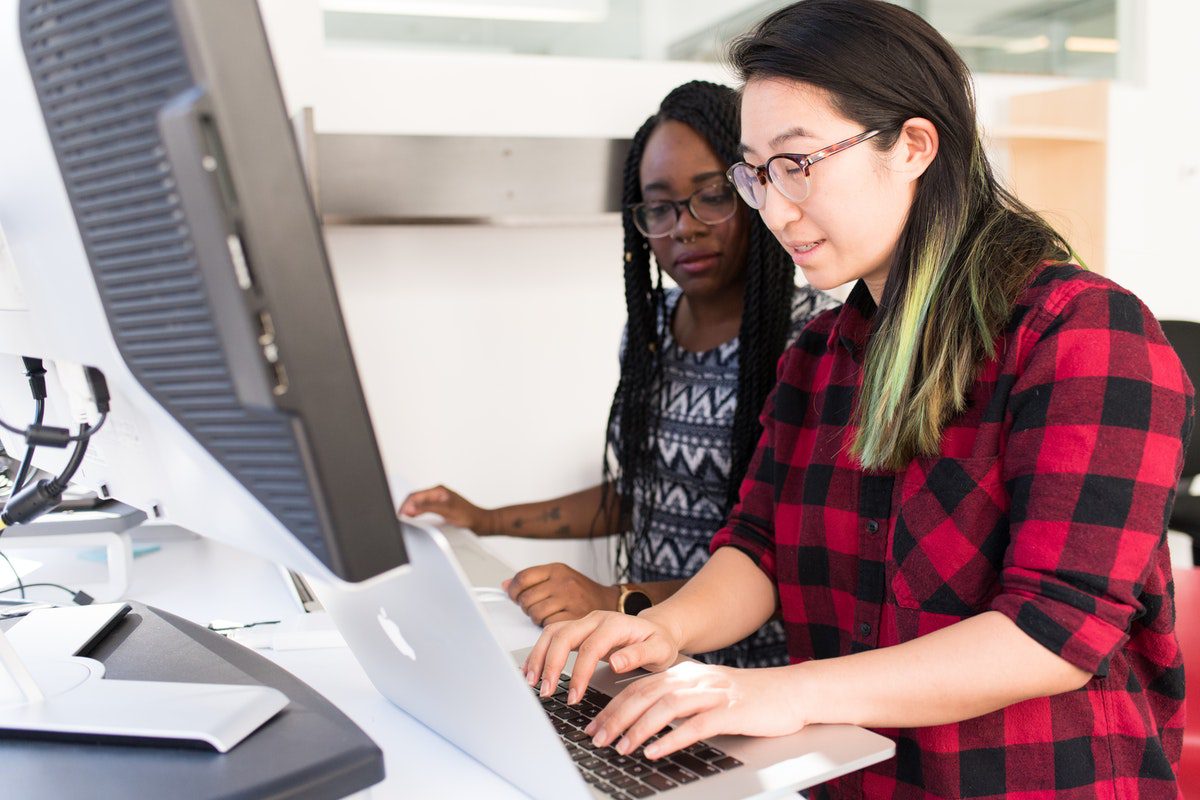
Learning a new language can be difficult, however, you don’t have to go about it alone. Whether you want to test your theoretical knowledge, practice code, or learn C best practices, you can rely on the resources we’ve listed below to find help with C problems and speed up your learning.
C Exercises
Exercises are the C equivalent of workbooks. They reduce abstract theories into puzzles and questions and require you to apply your theoretical knowledge to solve them. C exercises are a great way to gain experience and develop your problem-solving skills.
C Projects
C projects are significantly more ambitious undertakings than practice exercises. Instead of just being simple practice problems, projects involve creating practical programs that solve real-world problems. Working on projects teaches you how to combine the various elements of what you’ve learned.
C Quizzes
Quizzes are a great way to add a little fun to learning C. They use multiple-choice questions and cover a range of topics, from C function questions to interpreting lines of code. Your job is to choose the correct answer while keeping to time. Quizzes won’t help you write code, but they help sharpen your knowledge and prepare you for interviews.
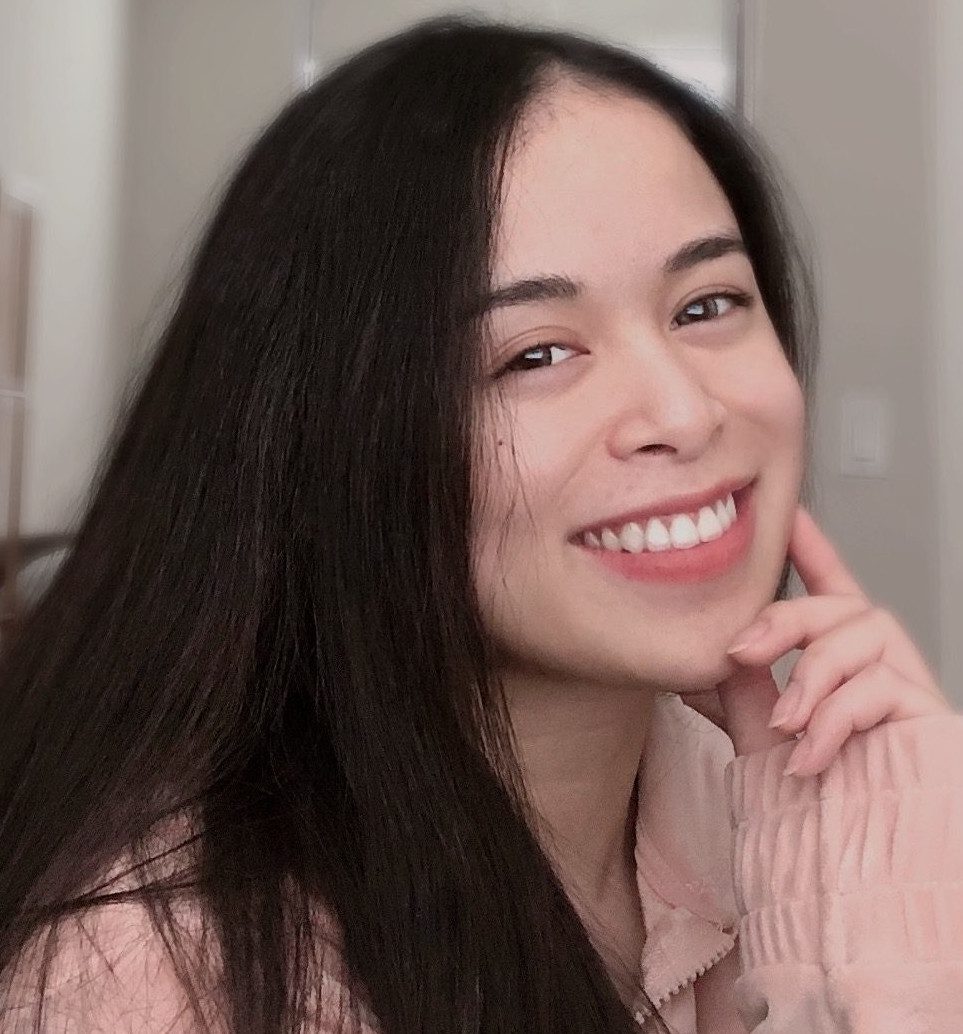
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
C Forums and Blogs
Blogs and forums are great places to get help with C from experts. It can sometimes be easier to understand a subject when it’s explained by another person rather than some technical C document. You can turn to informal blogs and forums and use simple language to get help learning C. DEV is a great community that is ready to welcome you and help you grow.
Where Can I Practice C?
If you’re ready to practice and improve your C skills, there are a variety of websites you can try out. We’ve listed the best websites to practice C, below.
Websites to Practice C
- HackerRank. HackerRank is a community of programmers where you can find C exercises with solutions for beginner, intermediate, and advanced learners. You can solve and form C programming exercises on HackerRank to earn Hackos, a form of currency you can only spend on HackerRank. It’s a fun way of learning C.
- HackerEarth. HackerEarth is a website with tons of practice problems with detailed solutions that you can use to practice C. Occasionally, this site organizes hackathons where you can test your skills against others.
- Topcoder. Topcoder organizes weekly calls for you to learn from experts and improve your skills in C. It also hosts a yearly tournament called the Topcoder Open, where developers compete for the chance to emerge as coding champions.
- Geeksforgeeks. Geeksforgeeks hosts a daily challenge where you can compete with other C developers to solve a programming exercise. Practicing every day is one of the best ways of getting better.
- CodeWars. Codewars has a unique practice style. This website lets you tackle small practice programs called “Kata”s and gives immediate feedback on your work, making it easy to learn from your mistakes.
What’s the Best Way to Learn C?
There’s no better way to learn to write programs in C than to actually write programs in C. If you’re thinking of getting started in C, you may want to think about attending bootcamps and taking online courses that can teach you how to write real programs in this language.
C Exercises FAQ
It can take you six months to a year to learn C. However, if you want to shorten that time, you should practice basic programming exercises daily and follow the above tips on how to get help with C.
You should start with keywords and identifiers. Every introductory C programming course starts with a lesson in keywords and identifiers. A keyword is a word with special meaning in C, while an identifier is a unique name that represents an entity.
When Daniel Ritchie developed C in 1972 at Bell Labs, he drew inspiration from B, an older programming language. Since C was to replace it, they named it the following letter in alphabetical sequence.
When it comes to functionality, C++ is better than C. It retains the original C features but includes new ones like object oriented programming, new data types, and better data security. However, there is still value in learning C. It is a lower-level programming language, so it runs faster than C++ and gives you more control over your code.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.