Are you considering building a command line interface? That’s a great project idea! In this guide, we discuss how to build a command line interface in Ruby.
Before we get started, you should have Ruby installed on your computer, and you should be using a terminal that allows you to run Unix commands. If you’re using a Linux or macOS-based computer, you’ll already have a Unix-compatible terminal built-in. Once you’ve met these requirements, you’re ready to get started!
What are Command Line Interfaces?
As you get started in your coding career, you soon learn about the terminal. The terminal window allows you to interact with command line interfaces (CLIs), which you can use to run and interact with programs using text commands.
Using the terminal window, you can give instructions to your computer without a mouse or a visual application. This saves you time when you code because you can just focus on writing instructions. You don’t need to worry about learning a new user interface for each tool you use!
The CLIs you have interacted with so far have probably been developed by other people. For example, the Python CLI allows you to run Python from the terminal, while the Ruby CLI allows you to do the same with Ruby applications. Fortunately, you can build your own too!
How to Build a CLI in Ruby
To get started, open a terminal window on your machine. Then, use the mkdir
command to create a folder for your code. Here’s an example of this command in action:
mkdir ruby-cli
This command creates a folder called “ruby-cli” on our computer. We can now use cd
to go into this folder and create our CLI:
cd ruby-cli
Great! Now we’re in our new “ruby-cli” folder. That’s all the setup we need to do for this article—the next step is to start writing our command line interface.
The first feature we’re going to add to our command line interface is to print out the term “This is an example CLI” to our terminal. We can do so by creating a new file called “home.rb” and inserting code into the file.
To create a new file, we can use the following command:
nano home.rb
This will open up a nano
text editor where we can put the code for our program. You can use a different text editor, such as vim or emacs, if you want.
In our nano text editor, we paste the following code:
#!/usr/bin/env ruby puts "This is an example CLI"
Now, let’s save by pressing Control-X on Mac, Y and then Enter. This creates a new file called “home.rb” in our “ruby-cli” folder.
In our code, the first line tells the computer to use the Ruby interpreter to run our code. This ensures that our code is run in Ruby. The second line instructs our program to print “This is an example CLI” to the console.
Now, we can run our file using the ruby
command:
ruby home.rb
Our code returns:
This is an example CLI.
As you can see, we’ve just created a simple CLI. When we run our “home.rb” file, the term ‘This is an example CLI” is printed to the console.
Accepting User Input Using Flags
Our CLI is pretty simple so far—it only prints out a message to the console. What if you wanted to accept user input? We can do so using the optparse
library. This allows us to parse options provided from the command line which can be used in our program.
We’re using the optparse
library for a few reasons. First, the optparse
library allows us to generate a help menu for each command. Second, optparse
is built-in to Ruby, so you don’t need to install anything.
Let’s add in a feature that accepts a user’s email address as an input and then returns it to the console.
Open up the “home.rb” file in your text editor and replace its contents with this code:
#!/usr/bin/env ruby require 'optparse' options = {} OptionParser.new do |op| op.on("-e", "--email", "Your email address") do |value| options[:name] = value end end.parse! puts 'Your email address is: ' + options[:name]
In this code, we use the optparse
library to parse data that is sent through a command. We then assign the value the user has specified to a variable, and print it out to the console. In this case, we print “Your email address is: “, followed by the email the user has specified.
We can use this command to try out our code:
ruby home.rb -e jack@jones.com
Our code returns:
Your email address is: jack@jones.com
The cool thing about the optparse
library is that it auto-generates a help menu. We can see this help menu by using the following code:
ruby home.rb --help
Our code returns:
Usage: ruby [options] -e, --email Your email address
When you’re running your command, you can use either the -e
or the --email
flag.
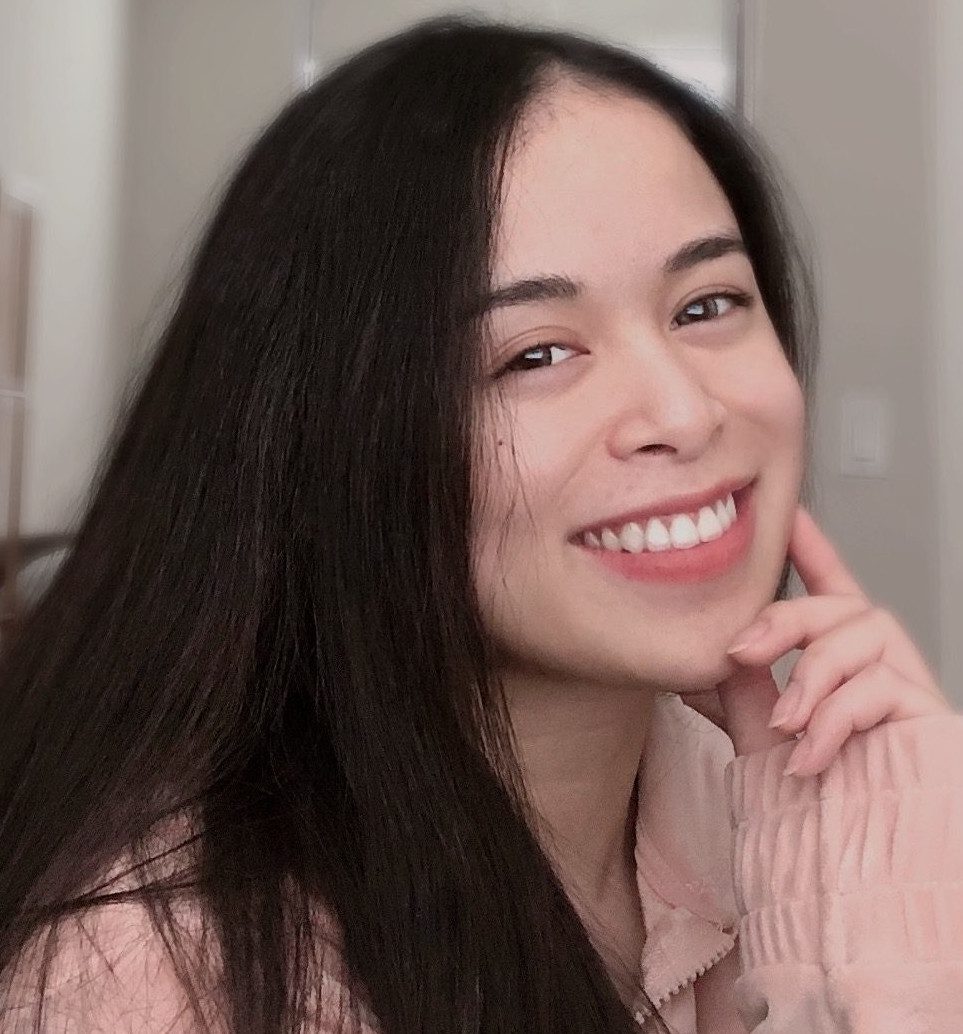
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Accepting User Input in Your Program
In the previous example, we accepted user input using flags. However, flags can be impractical if you want to accept multiple pieces of information.
The beauty of creating a CLI in Ruby is that any Ruby program can become a CLI. So, you don’t need to learn any new conventions to get started.
Suppose we want to build an application that retrieves a user’s name and email, and checks whether they are over the age of 16.
Let’s start by running the following command to enter into our nano
text editor:
nano age_checker.rb
In your newly-created file, paste the following code:
puts "What is your name?" name = gets puts "What is your age?" age = gets if age.to_i >= 16 puts "#{name}! You are 16 or older!" else puts "#{name}! You are not over the age of 16!" end
After saving your file, you can run your new CLI using this command:
ruby age_checker.rb
Our code returns:
What is your name? James What is your age? 17 James! You are 16 or older!
If we insert the value “14” in response to the question “What is your age?”, the following is returned:
James! You are not over the age of 16.
You can write any Ruby code you want into your CLI. The only limit is your imagination.
Conclusion
A CLI allows you to interact with a program using a terminal. It’s an alternative to a user interface for a program (like what you see when you open a text editor like Atom or Notepad).
In this guide, we’ve shown you how to build a simple CLI using Ruby. Now you’re ready to start building your own CLIs like a Ruby expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.