A modal is a window that is separate from the main window of a web page. It’s main purpose is to disable the main window from user interaction and to share information or confirm something with the user. Modal windows are popular to use as log-in components, confirmation of user input, or a myriad of other uses.
Bootstrap is a framework that can help us create components super quickly. In this article, we review how to get Bootstrap set up, learn why we might need a modal, and take a look at some examples of modals in action using the Bootstrap framework.
Getting Started
To make sure we can view a modal in our web page, make sure we have the proper dependencies. For this project, we need Bootstrap, Popper.JS and jQuery. Navigate to Bootstrap’s Quick Start page for assistance with getting all your dependencies.
It’s up to you how to link the packages we need, but the easiest, most beginner-friendly way is to use the content delivery network – the CDN – for jQuery, Popper.js, and Bootstrap. Be careful of the order of your <script> tags – order matters here.
When to Use a Modal
Modals are great to use for web-based applications that need to get a user’s attention once in a while.
When logging in to your bank’s website, for instance, then navigate to another tab, and then go back to the first website you might see a modal that has popped up, indicating you are to be logged out due to inactivity. Detecting inactivity is a prime example of when a modal is needed. The bank’s setup doesn’t allow further interaction on the site until the user has made the decision to continue to stay logged in or log out.
From a development perspective, modals help to keep the website from refreshing to a new page when it’s not necessarily needed. Any number of shopping retailers use this as a way to provide a “Quick View” for their users so the user can not only navigate their site faster, but complete their shopping faster. Quick Views don’t require an entire page reload to get an item’s information.
If a user needs to access main screen content while interacting with a modal, reach for another UI element to use instead. The point of a modal is to take the interaction with the main window away, giving control to the modal.
Use Bootstrap to Create a Modal
Modals are traditionally built with HTML, CSS and JavaScript. All of these languages are important to the creation of the modal, but JavaScript is imperative to get modals to work. JavaScript the switch that will turn the modal on or off.
When using Bootstrap, it’s good to have the documentation at hand so you can properly copy and paste the modal code. As you are learning to use Bootstrap, and how these components work, I recommend copying/pasting and then commenting the code so you can know or figure out what each line of code is doing.
Since we are not reinventing the wheel here by using Bootstrap’s modal code, it’s crucial to know how it works so you can explain it to another person. It’s not worth it to copy and paste something if you’re not able to explain why it works.
Launching the Modal With a Button
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#exampleModal" >Launch demo modal </button>
This code comes directly from Bootstrap’s documentation on how to create a modal using their framework. The rest of the article is spent on going through the code so we can be sure of what the markup means. We cover mainly attributes in this section.
class="btn btn-primary"
We know what class means as it relates to CSS. Here it is no different. The classes listed correspond to the Bootstrap stylesheet that we referenced in the head of the HTML document.
The “btn” class controls most of the styling of the button; the color is controlled by the second class name. Try changing “btn-primary” to another common button color (i.e. btn-secondary or btn-danger) to see what happens.
data-toggle="modal"
This attribute corresponds to the data-dismiss attribute that’s part of the button inside the modal.
data-target="#exampleModal"
The data-target attribute targets the code block that has an id of exampleModal. The exampleModal is our actual modal content. It grabs the information from the identified block.
This is especially crucial if we have multiple modals on the same page. You want to change the data-target attribute and the id so that only one modal will open at a time.
Event handlers are all managed by Bootstrap using jQuery and Popper.js. No need to worry about what to do when a user clicks as it’s already handled! To make sure it continues to work, do not change any of the original class names as this is how they are selected in our CSS.
Main Modal Element
<div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true" > ... Modal stuff here ... </div>
As with above, this code comes directly from Bootstrap’s documentation. The purpose of this section is to go over the markup to be certain what it means.
class="modal fade"
The main modal element is launched by using a button on the main web page. The class names here indicate we have two separate selectors for our modal. The first indicates the styles for the modal itself. The second indicates that this element transitions using a fade-in and fade-out technique.
id="exampleModal"
We have seen the id attribute before. It is how we target an element for changing the style. In this instance, it is also the target of the launch button on the main page. It matches the “data-target” attribute.
tabindex="-1"
The tabindex usually indicates that the element is reachable by keyboard. This is not the case when the number is negative. It’ll be focused by using JavaScript and/or a click event.
aria-labelledby="exampleModalLabel" aria-hidden="true"
The aria attributes are straightforward and refers to accessibility. The labelledby aria attribute is usually associated with that modal-title id. The hidden attribute’s true value indicates it is not seen by screen readers.
Creating Your Own Modal Element With Bootstrap Starter
You can put your own spin on Bootstrap’s modal by changing up the styles. The cascading nature of CSS still reigns supreme so overwriting the CSS can be done with creating a new class and attaching it to the class name separated by a space, or by overwriting a class name using the important keyword. The latter should only be used in extreme cases. Most often, we will see changes done with a custom class name.
<!DOCTYPE html> <!--[if lt IE 7]> <html class="no-js lt-ie9 lt-ie8 lt-ie7"> <![endif]--> <!--[if IE 7]> <html class="no-js lt-ie9 lt-ie8"> <![endif]--> <!--[if IE 8]> <html class="no-js lt-ie9"> <![endif]--> <!--[if gt IE 8]><!--> <html class="no-js"> <!--<![endif]--> <head> <meta charset="utf-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <title>Bootstrap Modal</title> <meta name="description" content="" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <!-- Link to Bootstrap Stylesheet --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.5.0/css/bootstrap.min.css" /> <style> body { max-width: 1400px; width: 100%; padding: 20px; margin: 20px; } .btn-ck { background-color: goldenrod; border: 1px solid black; color: black; } .btn-ck:hover { background-color: rgb(231, 203, 131); border: 1px solid lightgray; } .custom-modal { background: rgb(250, 249, 243); height: 400px; width: 500px; position: fixed; top: center; left: center; } </style> </head> <body> <!--[if lt IE 7]> <p class="browsehappy"> You are using an <strong>outdated</strong> browser. Please <a href="#">upgrade your browser</a> to improve your experience. </p> <![endif]--> <!-- Button triggers modal --> <h1>Modal Example</h1> <button type="button" class="btn btn-primary btn-ck" data-toggle="modal" data-target="#exampleModal" > Launch demo modal </button> <!-- Modal --> <div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true" > <div class="modal-dialog"> <div class="modal-content custom-modal"> <div class="modal-header"> <h5 class="modal-title" id="exampleModalLabel"> CareerKarma Demo Modal </h5> <button type="button" class="close" data-dismiss="modal" aria-label="Close" > <span aria-hidden="true">×</span> </button> </div> <div class="modal-body"> You are reading a modal with a bit of custom styling... </div> <div class="modal-footer"> <button type="button" class="btn btn-secondary" data-dismiss="modal" > Close </button> <button type="button" class="btn btn-primary btn-ck"> Save changes </button> </div> </div> </div> </div> <!-- The following CDN's go right before the closing body tag. --> <!-- jquery CDN first --> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.5.1/jquery.min.js" async defer ></script> <!-- popper.js CDN second --> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/2.4.4/umd/popper.min.js" async defer ></script> <!-- bootstrap CDN third --> <script src="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.5.2/js/bootstrap.min.js" async defer ></script> </body> </html>
In the code editor above, we’ve used CSS to change the style of the buttons and the background of the modal. In this example, I’ve added a custom class name instead of trying to overwrite any of the CSS that Bootstrap already provides using the !important keyword.
Conclusion
The main hurdle when creating a modal using Bootstrap is making sure documentation is ready correctly. Quite often if there is an error, it’s most likely due to a copy/paste error, an error in position of the CDN or stylesheet in the head/body of your HTML or that your CSS is not targeting the correct element.
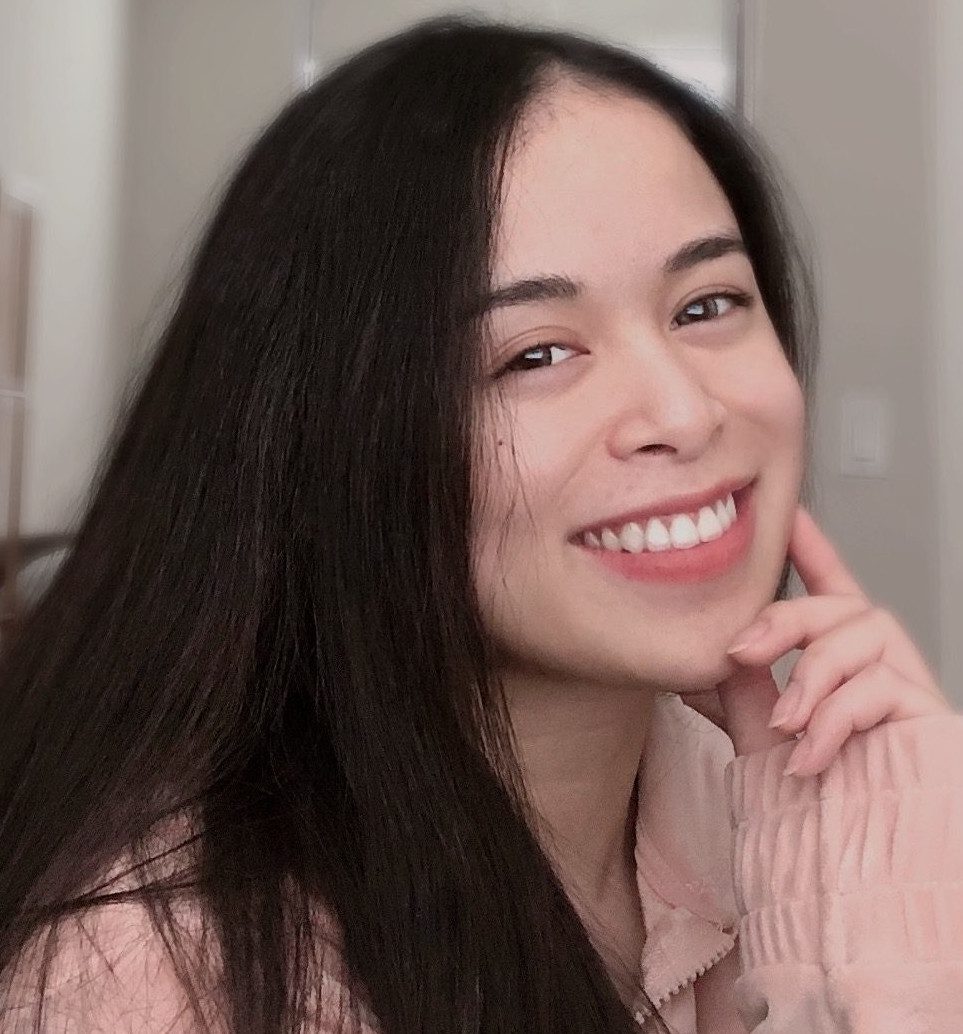
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Some of this custom styling will come with trial and error. What’s great is that Bootstrap’s documentation is very thorough – if you’ve not learned another framework or package prior to this one, you’re lucky in that the documentation is pretty great and fairly simple to understand.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.