No matter if you’re making a simple one page portfolio website, a monolithic app for a huge company, a blog, or a forum, chances are you’re going to need a dropdown menu.
A dropdown menu is especially useful when you have lots of links or actions, but not enough space on the page to display them all at once.
Usually, dropdowns require a bit of JavaScript.
If you haven’t started learning it yet or just want a quick solution, Bootstrap is the perfect fit.
What is Bootstrap?
You’ve probably heard the word thrown around lots of times, but what is Bootstrap?
It’s a front-end CSS (with a bit of JavaScript) framework. This means that it’s a kind of scaffolding made beforehand to speed up development considerably. Programmers commit a certain amount of time to develop a standard to which to hold up to, and include all the prerequisite setup necessary into the framework itself, so we don’t have to do it each time.
A standardized styling is applied to certain components in Bootstrap that are often built, like button elements, button groups, and navigation bars. This means that we can apply all those styles using a few predefined keywords instead of writing numerous lines of code repeatedly.
This helps a lot with consistency as a team of multiple developers working on larger applications don’t need to worry about applying the same styles. All styles are defined in the framework.
Setting up Bootstrap
To use Bootstrap, we need to add a few lines of code to our HTML structure.
We’re going to use the Bootstrap CDN for the fastest and simplest setup.
First of all, we need a proper HTML5 template, which is easily done in modern code editors. In VSCode, simply type ! and press enter or tab to get the HTML5 document structure which looks like this:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> </body> </html>
Now, inside of the <head> tag, paste the following lines above any other stylesheets:
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" integrity="sha384-9aIt2nRpC12Uk9gS9baDl411NQApFmC26EwAOH8WgZl5MYYxFfc+NcPb1dKGj7Sk" crossorigin="anonymous" />
Bootstrap depends on some JavaScript libraries under the hood, so we also need to include the following <script> tags right before the closing </body> tag:
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous" ></script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js" integrity="sha384-Q6E9RHvbIyZFJoft+2mJbHaEWldlvI9IOYy5n3zV9zzTtmI3UksdQRVvoxMfooAo" crossorigin="anonymous" ></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/js/bootstrap.min.js" integrity="sha384-OgVRvuATP1z7JjHLkuOU7Xw704+h835Lr+6QL9UvYjZE3Ipu6Tp75j7Bh/kR0JKI" crossorigin="anonymous" ></script>
That’s all the setup we need. We’re now ready to bootstrap our project.
Utilising Bootstrap
The power of Bootstrap is utilised by using it’s keywords as classes of HTML tags. By using it, you can style your page completely without even making a CSS file!
For instance, here’s a simple button group:
<div class="btn-group"> <button class="btn btn-primary">button 1</button> <button class="btn btn-secondary">button 2</button> <button class="btn btn-info">button 3</button> <button class="btn btn-success">button 4</button> <button class="btn btn-danger">button 5</button> <button class="btn btn-light">button 6</button> <button class="btn btn-dark">button 7</button> <button class="btn btn-white">button 8</button> <button class="btn btn-warning">button 9</button> </div>
And the outcome:
We’ve made a button group with all of Bootstrap’s preset colors (muted excluded as it’s reserved for text).
Now let’s get on to dropdowns.
Bootstrap Dropdowns, Various Ways
In older versions of Bootstrap, we had to use <a> tags exclusively for dropdowns. Since version 4 <button> tags are accepted as well.
A note on links and buttons:
Links should be used for navigation purposes only. Whether within the current page, or to send the user to a different page, but a link’s only function should be navigation.
On the other hand, any kind of action like log in, sign up, submit form, hide section, change color theme etc. should be handled with buttons.
Any sort of JavaScript click-related actions should be done with buttons.
Also, links can only be accessed with the enter key, whereas buttons can be accessed with enter or space which is important to take into account for accessibility purposes.
In any case, the data-toggle=”dropdown”
attribute is necessary in order for it to work.
Dropdown with Buttons
<div class="dropdown"> <button class="btn dropdown-toggle" data-toggle="dropdown"> Button menu </button> <div class="dropdown-menu"> <a href="#" class="dropdown-item">This dropdown</a> <a href="#" class="dropdown-item">Uses</a> <a href="#" class="dropdown-item">Buttons</a> </div> </div>
We wrap the whole thing in an element (most usually a <div>) with class=“dropdown”, and make a button with class=“btn dropdown-toggle” and data-toggle=“dropdown” as the first element. This will act as the toggle for the dropdown menu.
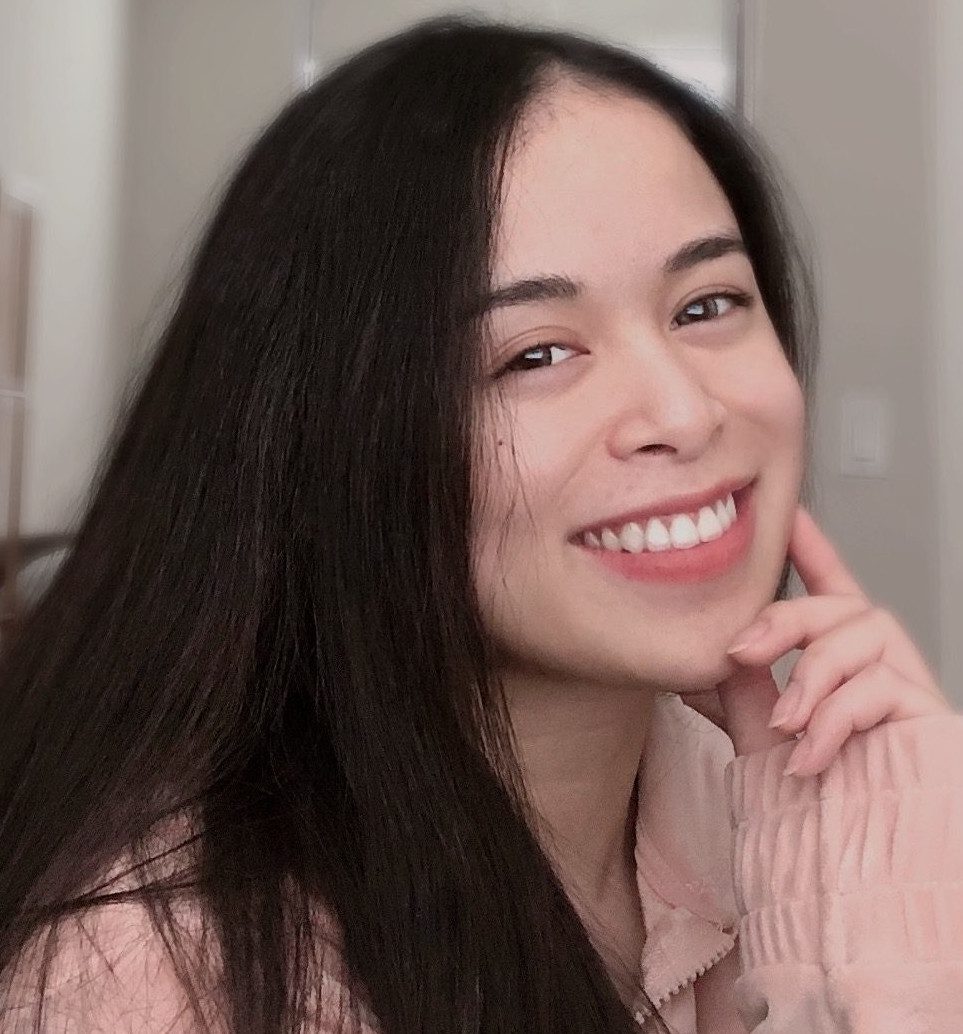
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Then, we make a container for the actual dropdown, again most usually a <div> or <ul> is used, with class=“dropdown-menu”.
Lastly, we make all the items that we want inside of the menu with class=“dropdown-item”. These are usually links to other pages or sections on the current page.
The result:
Hooray, you’ve made your first dropdown menu with Bootstrap!
Dropdown with Links
<div class="dropdown"> <a class="btn dropdown-toggle" data-toggle="dropdown"> Link menu </a> <ul class="dropdown-menu"> <a class="dropdown-item" href="#">This dropdown</a> <a class="dropdown-item" href="#">Uses</a> <a class="dropdown-item" href="#">Links</a> </ul> </div>
The exact same thing can be done using <a> tags. We also used <ul> this time for the menu, and the results are the same.
Styling a Menu and It’s Items
Bootstrap styles can be added to the menu and items. Let’s add some colors to our menu. We can do this by prefixing one of Bootstrap’s colors with bg- which stands for background:
<div class="dropdown"> <a class="btn btn-danger dropdown-toggle" data-toggle="dropdown"> Styled menu </a> <div class="dropdown-menu"> <a href="#" class="dropdown-item bg-primary">This is a</a> <a href="#" class="dropdown-item bg-warning">Styled</a> <a href="#" class="dropdown-item bg-success">Dropdown</a> </div> </div>
Which results in:
Split Button Dropdown
Another interesting option is to have a split button. With this we get double functionality. A button to serve one purpose, and the caret to access the dropdown. A potential use case is to have the button open a page containing all the items from the menu, and the individual links leading to their own separate pages:
<div class="dropdown"> <div class="btn-group"> <a href="#" class="btn btn-danger">All Menu Items</a> <a class="btn btn-danger dropdown-toggle dropdown-toggle-split" data-toggle="dropdown" ></a> <div class="dropdown-menu"> <a href="#" class="dropdown-item bg-primary">Item #1</a> <a href="#" class="dropdown-item bg-warning">Item #2</a> <a href="#" class="dropdown-item bg-success">Item #3</a> </div> </div> </div>
In this case, we need to make a container with class=“btn-group” to wrap the button and the toggle, and add dropdown-toggle-split to the toggle button.
Form inside a Dropdown
We can also place an HTML form inside a dropdown menu:
<div class="dropdown" data-toggle="dropdown"> <button class="dropdown-toggle btn-danger btn">Dropdown Form</button> <div class="dropdown-menu"> <form> <div class="form-group"> <label for="dropdown-form-name">Your name</label> <input type="text" class="form-control" id="dropdown-form-name" /> </div> <div class="form-group"> <label for="dropdown-form-password">Your password</label> <input type="password" class="form-control" id="dropdown-form-password" /> </div> <input type="button" class="btn" value="Press Me!" /> <span class="sr-only">Submit Form</span> <button type="submit" class="btn btn-success">Submit</button> </form> </div> </div>
We simply make a regular form within the dropdown-menu container.
The most common use case for this is a simple login form, just like in our example.
Note: Bootstrap included the sr-only class seen in the example, which stands for screen-readers only. The element is hidden on the page and only gets recognized by screen readers. This is important for accessibility purposes.
Dropup, Dropleft, Dropright
A few variants on the regular dropdown menu:
The Dropup Menu
We can use the dropup menu on a navigation bar that’s docked to the bottom of the page:
<div class="dropup"> <a class="btn btn-danger dropdown-toggle" data-toggle="dropdown"> Dropup menu </a> <div class="dropdown-menu"> <a href="#" class="dropdown-item">This is a</a> <a href="#" class="dropdown-item">Bootstrap</a> <div class="dropdown-divider"></div> <a href="#" class="dropdown-item">Dropup menu</a> </div> </div>
The Dropleft Menu
We can use the dropleft menu when the button that opens it is touching the right edge of the screen, so that our menu doesn’t go off screen.
<div class="dropleft"> <a class="btn btn-danger dropdown-toggle" data-toggle="dropdown"> Dropleft menu </a> <div class="dropdown-menu"> <a href="#" class="dropdown-item">This is a</a> <a href="#" class="dropdown-item">Bootstrap</a> <div class="dropdown-divider"></div> <a href="#" class="dropdown-item">Dropleft menu</a> </div> </div>
The Dropright Menu
And the dropright is the same as dropleft, only on the opposite side of the screen.
<div class="dropright"> <a class="btn btn-danger dropdown-toggle" data-toggle="dropdown"> Dropright menu </a> <div class="dropdown-menu"> <a href="#" class="dropdown-item">This is a</a> <a href="#" class="dropdown-item">Bootstrap</a> <div class="dropdown-divider"></div> <a href="#" class="dropdown-item">Dropright menu</a> </div> </div>
In each of these we’ve included another bootstrap feature, an empty div with a class of dropdown-divider which gives us a nice separation of sections within the menu.
Dropdown With Header
We can have header text in our dropdown menu by making an element with a class of dropdown-header like so:
<div class="dropdown m-4" data-toggle="dropdown"> <button class="dropdown-toggle btn-info btn">Entitled Dropdown</button> <div class="dropdown-menu"> <h2 class="dropdown-header">I'm a title</h2> <a class="dropdown-item" href="#">We are</a> <a class="dropdown-item" href="#">Items</a> </div> </div>
Why use a heading instead of just a paragraph, span, or other text tag?
A heading <h1-h6> plays a role in search engine optimization (SEO), so it’s worth placing them where appropriate.
If our dropdown hosts an important section of our website, it might make sense to give it some type of heading. Bootstrap also has some formatting applied to heading tags, which we can see in our example:
Right-Aligned dropdown
By default, Bootstrap’s dropdown menus are left-aligned with the their toggle button.
If a right-aligned menu would be a better fit for your design, or you simply prefer it that way, you can add a class of dropdown-menu-right to it:
<div class="dropdown"> <a class="btn btn-danger dropdown-toggle" data-toggle="dropdown"> Right-aligned </a> <div class="dropdown-menu dropdown-menu-right"> <a href="#" class="dropdown-item">This is a</a> <a href="#" class="dropdown-item">right-aligned</a> <div class="dropdown-divider"></div> <a href="#" class="dropdown-item">Dropdown menu</a> </div> </div>
Active And Disabled Items In Dropdown
You can make a dropdown item disabled or active simply adding the appropriate class:
<div class="dropdown m-4"> <button class="btn btn-secondary dropdown-toggle" data-toggle="dropdown" > Disabled button menu </button> <div class="dropdown-menu"> <a href="#" class="dropdown-item">This is a</a> <a href="#" class="dropdown-item disabled">Disabled</a> <a href="#" class="dropdown-item">Button</a> </div> </div>
When might you want a disabled item in your menu? Maybe the option is currently unavailable, like worldwide shipping, the item is out of stock, or similar.
<div class="dropdown m-4"> <button class="btn btn-success dropdown-toggle" data-toggle="dropdown"> Active button menu </button> <div class="dropdown-menu"> <a href="#" class="dropdown-item">This is</a> <a href="#" class="dropdown-item active">An active</a> <a href="#" class="dropdown-item">Button</a> </div> </div>
An active class on the other hand is made to grab the attention of the viewer, highlighting the current, or focused element. A potential use case for setting this class statically (without changing it or moving it from element to element) is for call-to-action (buy, register, subscribe etc.) buttons, or simply leading the users attention towards a certain element.
You’re ready for Bootstrap
Bootstrap is a very powerful framework built for rapid development, by abstracting away the setup necessary on each project, for each component.
Today we learned how we can make different types of dropdowns with it, with no JS involved (at least not on our part).
Now go and bootstrap a project!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.