Bootstrap Cards
The Bootstrap card is one of its most popular components, and with good reason. They serve as a very flexible media container, and have some nice preset styling and formatting.
Card content can be anything from text, images, links, buttons, lists, and can also have a header and footer.
Bootstrap Card Basics
Bootstrap cards are made with flexbox, and expand to the width of their containing element by default. If we want to specify a different width, we can either use Bootstrap’s width utility class on the card element itself, or we can wrap the card in a containing element, and size it with Bootstrap’s column classes.
Let’s take a look at a few examples:
<div class="container-fluid bg-light"> <div class="card p-4 m-4">card 1</div> <div class="card p-4 m-4">card 2</div> <div class="card p-4 m-4">card 3</div> <div class="card-group m-4"> <div class="card p-4">card 4</div> <div class="card p-4">card 5</div> <div class="card p-4">card 6</div> </div> <div class="card-deck m-4"> <div class="card p-4 m-4">card 7</div> <div class="card p-4 m-4">card 8</div> <div class="card p-4 m-4">card 9</div> </div> </div>
Bootstrap’s rule of thumb is to wrap everything that’s inside the <body> in a CONTAINER element, which makes everything inside of it responsive.
That means that it will shift size and layouts according to the current screen size.
In our example, we used CONTAINER-FLUID, which just makes the element full-width, as opposed to just CONTAINER, which adds horizontal margin and centers it on the page.
Next, we have our first set of three cards. They have no parent element other than the container so they have the default card behavior and are arranged top to bottom as we see in the screenshot:
The p-4 and m-4 you see in the code snippet represent a padding on all sides of 1.5 rem, and a margin on all sides of 1.5 rem respectively.
Bootstrap sizing utility values:
0 = 0 rem;
1 = 0.25 rem;
2 = 0.5 rem;
3 = 1 rem;
4 = 1.5 rem;
5 = 3 rem;
Then we have cards 4, 5, and 6, which are wrapped in a <div> with the CARD-GROUP class. They behave exactly like the BTN-GROUP class. They are joined together in a horizontal flexbox layout, and a rounded border is added to the whole group.
Lastly, cards 7, 8, and 9 are wrapped in a <div> with the CARD-DECK class, which joins them in a horizontal flexbox, but leaves them as separate elements visually.
Manually sizing cards
Before we do some manually sized cards, let’s learn a bit about Bootstrap’s layout and breakpoint system.
Bootstrap Columns
Bootstrap divides the page in 12 columns for easy layout development.
Here’s a quick demo to visualize it:
<div class="row"> <div class="col border p-5">column 1</div> <div class="col border p-5">column 2</div> <div class="col border p-5">column 3</div> <div class="col border p-5">column 4</div> <div class="col border p-5">column 5</div> <div class="col border p-5">column 6</div> <div class="col border p-5">column 7</div> <div class="col border p-5">column 8</div> <div class="col border p-5">column 9</div> <div class="col border p-5">column 10</div> <div class="col border p-5">column 11</div> <div class="col border p-5">column 12</div> </div>
First we have a wrapper for the columns called a ROW, which is just a flexbox container for them. We declare an element’s sizing by writing COL-SCREEN SIZE BREAKPOINT-NUMBER OF COLUMNS TO OCCUPY.
In our above example, we didn’t provide the breakpoint as we want this sizing no matter the size of the screen, and no number of columns because omitting it defaults to COL-1 so we get our natural 12 columns. Here’s what it looks like:
Bootstrap Breakpoints:
Bootstrap is a mobile-first framework, meaning that when developing with Bootstrap you prioritize mobile layouts and work your way upwards. All of it’s classes affect the element from the breakpoint provided and upwards.
Extra small is the default so omitting the break point completely, like P-4 for example, is the same as writing P-XS-4 which would apply it on XS screen size and all of the larger ones.
In other words, if you want a rule that’s effective on all screens just omit the breakpoint.
If we wanted to reduce the padding from the example to 0.5 rem from the medium screen size and above, we’d add the P-MD-2 class on the same element.
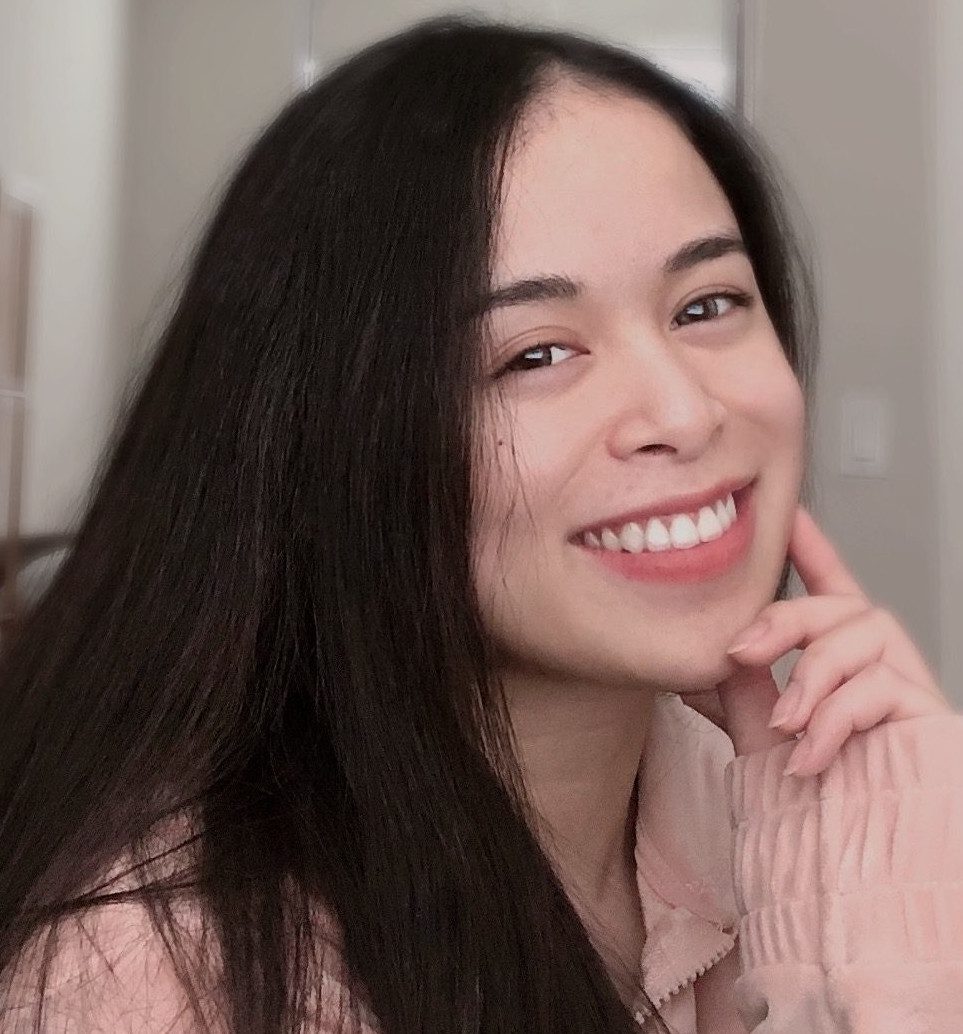
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Breakpoint pixel values:
- xs – screen size below 576 pixels. This is the default.
- sm – screen size from 576 pixels and above.
- md – screen size from 768 pixels and above.
- lg – screen size from 992 pixels and above.
- xl – screen size from 1200 pixels and above.
Now let’s use this to make our layout change depending on the screen size:
<div class="row"> <div class="col-5 col-md-7"> <div class="card p-4">left column card</div> <div class="card p-4">left column card</div> <div class="card p-4">left column card</div> <div class="card p-4">left column card</div> </div> <div class="col-7 col-md-5"> <div class="card p-4">right column card</div> <div class="card p-4">right column card</div> <div class="card p-4">right column card</div> <div class="card p-4">right column card</div> </div> </div>
Again, we have our ROW wrapper, and then a <div> set to COL-5 COL-MD-7, which means it will occupy 5 columns from the smallest screen size and upwards, but as soon as it reaches the medium screen size, it will change to 7 columns. After it we have a <div> with the reverse, COL-7 COL-MD-5, they’re both filled with a few cards. I grabbed the screen size in the screenshot so you can see how it changes:
767 pixels width, our left <div> is 5 columns wide and the right is 7.
We go up 1 pixel to 768 and they change to the opposite:
Card Subcomponents
Let’s now utilize the full power of cards by trying out all of their subcomponents.
Card with Header and Footer
<div class="card"> <div class="card-header text-center p-4">I'm a header</div> <div class="card-body"> <p class="card-text"> first paragraph </p> <p class="card-text"> second paragraph </p> <a href="#" class="card-link">Link 1</a> <a href="#" class="card-link">Link 2</a> <a href="#" class="card-link">Link 3</a> </div> <div class="card-footer"> Footer of a Bootstrap card </div> </div>
We created a card with a centered card header, which has a border and a light gray background, and a card body below it, which gives it some padding.
In the body, we have a few paragraphs with CARD-TEXT for some alignment, and a few links with CARD-LINK, so they’re colored blue and laid out next to each other.
At the end, we made a card footer which has the same styling as the header, but we didn’t center the text this time.
Card With an Image Cap
This time, we’ll add an image cap to the card, a title and subtitle to the card’s body, and a list group with links as list items:
<div class="card"> <img src="https://via.placeholder.com/100" alt="" class="card-img-top" /> <div class="card-body"> <h3 class="card-title">card title in the card's body</h3> <h4 class="card-subtitle mb-4">card subtitle</h4> <p>list group with links inside:</p> <ul class="list-group"> <li class="list-group-item"> <a href="#" class="card-link">List link 1</a> </li> <li class="list-group-item"> <a href="#" class="card-link">List link 2</a> </li> <li class="list-group-item"> <a href="#" class="card-link">List link 3</a> </li> </ul> </div> </div>
I’m using a very handy tool for the images conveniently called placeholder. The number at the end is the pixel width x height of the image, but that gets ignored here as the CARD-IMG-TOP stretches (or shrinks) the image into the full width of the card. The title and subtitle get some nice formatting, and we’ve used one of Bootstrap’s orientation utilities for padding and margin. The MB-4 you see on the subtitle stands for margin bottom.
Bootstrap padding and margin orientation:
- pt/mt – padding/margin top
- pb/mb – padding/margin bottom
- pl/ml – padding/margin left
- pr/mr – padding/margin right
- py/my – padding/margin y axis (top and bottom)
- px/mx – padding/margin x axis (left and right)
Then we have a list group which again encapsulates the list and it’s individual elements with a light, rounded border. Here’s our result:
Bottom Image Cap
There’s no CARD-IMG-BOTTOM in bootstrap, but we can easily “fake” this by making a div at the bottom of the card and placing an <img class=”card-img-top” inside of it.
<div class="card"> <div class="card-body"> <p class="card-text"> Lorem, ipsum dolor sit amet consectetur adipisicing elit. Quas unde corporis sed nam ad eius, pariatur, consectetur modi asperiores dolorem id quaerat eos quod nesciunt repudiandae aut temporibus rerum possimus. </p> </div> <img src="https://via.placeholder.com/100" alt="" class="card-img-top" /> </div>
I also added the good old lorem ipsum for good measure.
Side Image Cap
We can also make it horizontal by adding a ROW wrapper inside of our card to lay it out horizontally, and then using COL to define the size of the image and the rest of the card:
<div class="card"> <div class="row"> <img src="https://via.placeholder.com/100" alt="" class="card-img-top col-6" /> <div class="card-body col-6"> <h3 class="card-title">card title in the card's body</h3> <h4 class="card-subtitle mb-4">card subtitle</h4> <p>list group with links inside:</p> <ul class="list-group"> <li class="list-group-item"> <a href="#" class="card-link">List link 1</a> </li> <li class="list-group-item"> <a href="#" class="card-link">List link 2</a> </li> <li class="list-group-item"> <a href="#" class="card-link">List link 3</a> </li> </ul> </div> </div> </div>
We just wrapped all the contents of the card in a <div class=“row”>
and added COL-6 to both the image and the card body to make the card 50-50.
Card Image as a Background
We can also stretch an image over the whole card and have the text, lists, links etc, layed over it. We do it by simply giving the <img> a class of CARD-IMG and then placing everything else in a <div> with class CARD-IMG-OVERLAY. Inside of it, we configure the elements however we want.
<div class="card"> <img src="https://via.placeholder.com/100" alt="" class="card-img" /> <div class="card-img-overlay"> <h4 class="card-title">Overlay Title</h4> <p class="card-text"> Image text overlay </p> <p>second paragraph</p> <p>third paragraph</p> <p>fourth paragraph</p> <div class="btn-group w-100"> <button class="btn btn-primary">blue</button> <button class="btn btn-info">teal</button> <button class="btn btn-danger">red</button> <button class="btn btn-dark">black</button> <button class="btn btn-success">green</button> </div> </div> </div>
Here we used the width utility for the first time. The W-100 on the BTN-GROUP.
Bootstrap Width and Height Utilities:
w/h-25/50/75/100 – width/height 25%/50%/75%/100%
In our case it’s 100% minus the same padding that CARD-BODY applies, that’s applied here by CARD-IMG-OVERLAY.
Card Columns
There is also this option to lay out the cards in columns so that they go top to bottom first, and only when the column is filled they start on a new one.
<div class="card-columns"> <div class="card"> <h3 class="card-title text-center p-2">Title of card 1</h3> <div class="card-body"> <p class="card-text"> Lorem ipsum dolor sit amet consectetur adipisicing elit. Aliquam, rerum excepturi voluptate... </p> </div> </div> <div class="card"> <h3 class="card-title text-center p-2">Title of card 2</h3> <div class="card-body"> <p class="card-text"> Lorem ipsum dolor sit amet consectetur adipisicing elit. Aliquam, rerum excepturi voluptate... </p> </div> </div> <div class="card"> <h3 class="card-title text-center p-2">Title of card 3</h3> <div class="card-body"> <p class="card-text"> Lorem ipsum dolor sit amet consectetur adipisicing elit. Aliquam, rerum excepturi voluptate... </p> </div> </div> <div class="card"> <h3 class="card-title text-center p-2">Title of card 4</h3> <div class="card-body"> <p class="card-text"> Lorem ipsum dolor sit amet consectetur adipisicing elit. Aliquam, rerum excepturi voluptate... </p> </div> </div> </div>
We simply wrap all of our cards in a <div class=“card-columns”> and we get the following:
Styling Bootstrap Cards
When it comes to styling bootstrap cards, we can change their background and text color, as well as the color and look of their borders.
The styles can be applied to the whole card, it’s separate subcomponents, or both. Here’s a few styled cards to check out:
<div class="card bg-primary"> <div class="card-header"><h3>Header 1</h3></div> <div class="card-body"> <p>Body 1</p> </div> <div class="card-footer"><p>Footer 1</p></div> </div> <div class="card bg-success text-white"> <div class="card-header"><h3>Header 2</h3></div> <div class="card-body"> <p>Body 2</p> </div> <div class="card-footer text-danger"><p>Footer 2</p></div> </div> <div class="card bg-info text-dark border-danger"> <div class="card-header"><h3>Header 3</h3></div> <div class="card-body"> <p>Body 3</p> </div> <div class="card-footer"><p>Footer 3</p></div> </div> <div class="card border-primary bg-dark text-white"> <div class="card-header border-success"><h3>Header 4</h3></div> <div class="card-body text-danger"> <p>Body 4</p> </div> <div class="card-footer bg-warning text-info"><p>Footer 4</p></div> </div>
The first card has a blue background (BG-PRIMARY) on the card itself, styling the whole card.
The second card has a green background (BG-SUCCESS) and white text (TEXT-WHITE) on the whole card, but red text (TEXT-DANGER) in the footer, overriding the parent element’s TEXT-WHITE.
The third card has a dark blue background (BG-INFO), black text (TEXT-DARK) and a red border (BORDER-DANGER).
Finally, the fourth card has a blue border (BORDER-PRIMARY), a black background (BG-DARK), and white text (TEXT-WHITE) on the whole card.
A green border (BORDER-SUCCESS) on the header, red text (TEXT-DANGER) in the body, and a yellow background (BG-WARNING) and dark blue text (TEXT-INFO) in the footer.
Here’s what our rainbow cards look like:
To recap
The Bootstrap card is quite a powerful component with lots of options. They can be grouped into a card-deck for a separated, cohesive whole, or into a card-group to fuse them into a single entity. They can be spread out in a row, or in card-columns for a different look.
They can host images, lists and links, all in various layouts.
They can also have their own header and footer, all with preset styles and formatting, but open for us to customize.
They are incredibly flexible, and probably one of the best examples of how much you can accomplish with Bootstrap, with so little previous setup, in so little time.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.