While loops. While loops. While loops. I could have written a bash program to write those sentences instead of writing while loops
three times over myself.
Loops are a foundational component of most programming languages. They allow you to automate and repeat similar tasks so that you can reduce repetition in your code.
In this guide, we’re going to talk about the while loop, how it works, and how you can use it in your bash scripts. We’ll walk through an example of a while loop so you can get started quickly.
Bash while Loop
While loops
are sort of like a repeating conditional statement. They run a block of code only when a condition evaluates to true. Otherwise, the loop does not execute.
While loops are conditionally based. This means that, unlike for loops, you don’t need to know how many times a block of code should repeat before the loop starts.
This is useful because it means you can keep your loop going until you change a variable. This may be more practical than having a loop that runs a specific amount of times in many scenarios.
Examples where a while loop could be useful include where you want a user to guess a number until they get it right or try to enter their password until they enter the correct one.
How to Write a while Loop
In bash, while loops are written like this:
while [condition] do [run commands] done
The syntax for the while loop reinforced a crucial part of bash’s syntax: it’s easy to read. Before we continue, take a moment to read the above syntax over in your head. It’s simple!
The run commands
will be executed until the condition we have specified is no longer true.
Let’s create a password-checking program to show how this works. In this program, we’re going to ask a user to insert their username and password. If their password is correct, the loop will print a message informing the user and then will stop; if the password is not correct, our loop will keep going.
Create a file called password.sh and paste in the following line of code:
found=false
We’ve just declared a variable. This variable will track whether the user has inserted the correct password. We’re setting its initial value to false
so that our while loop runs at least once.
Next, we’re going to add in this block of code to create our while loop:
while [[ $found == false ]] do echo "Insert your password." read password done
This code will ask our user for their password until the value of “found” is not equal to false. Now our user can insert a password, but we haven’t yet checked to see if this password is correct. Paste the following code directly after the read password
line from earlier:
if [[ $password == "test" ]]; then echo "You've entered the correct password." found=true else echo "Your password is incorrect." fi
We’ve declared a while loop which will keep running until the variable found
is no longer equal to false. When this loop executes, our user will be asked to insert their password, and the value the user enters is saved as the variable “password.”
Once a user has entered a password, an if
statement runs to check whether they’ve entered the correct password, which is test in this example. When a user inserts the correct password, “You’ve entered the correct password.
” is printed to the console. Our found variable is then set to true, which means that our while loop will not run again.
Here’s what happens when we insert the correct password:
Insert your password.
test
You’ve entered the correct password.
When a user inserts the wrong password, the else
statement in our if
statement will run. This will prompt the message “Your password is incorrect.” to the user. Then, our while loop will run again, until the user inserts the correct password:
Insert your password.
test123
Your password is incorrect.
Insert your password.
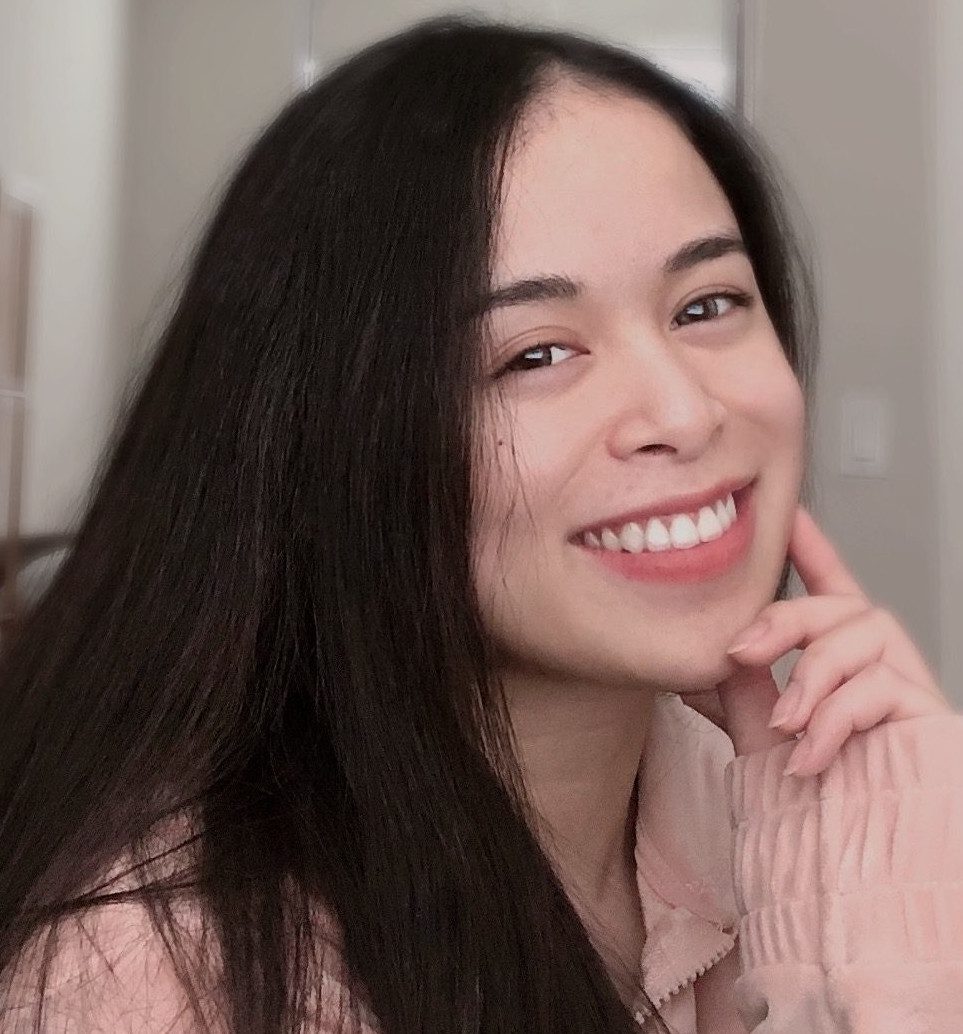
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
test
You’ve entered the correct password.
At first, we entered an incorrect password. This means that our program executes the else
statement in our if
statement, so we’re notified that our password is incorrect. On our next attempt, we successfully insert our password. This prompts us with a message informing us of such, then our program stops executing.
When you’re working with while loops, you may want to break out of them and halt your program. You can do this by pressing CTRL + C or Cmd + C, which will halt execution of your bash script.
Read a File Line By Line
There’s a lot you can do with a while loop – you could build a guessing game, for example – but one of the most common things you’ll find people do with a while loop is read a file line by line.
While loops are perfect for this case because they’ll run until a certain condition is met. That means you don’t need to know – or find out – how many lines are in a file. You can tell your program to print out each line while there are still lines to read.
Let’s say that we want to print out the file /etc/hosts
on our computer. Create a file called read_file.sh
and paste in this code:
file=/etc/hosts while read -r line; do echo $line done < $file
This example is slightly different from our last one. Instead of passing a condition with our while loop, we’re using input redirection (< $file
). This is used to tell our program from which file it should read. When the last file of our program is read, our program will halt execution.
Our code returns the contents of the standard /etc/hosts file, which are:
127.0.0.1 localhost
255.255.255.255 broadcasthost
Using Break and Continue Statements
While loops can be used alongside break and continue statements. These statements give you more control over the flow of your code:
- A break statement terminates the current loop.
- A continue statement exits the current iteration of a loop and allows the loop to continue iterating.
Break Statements
Let’s beef up our password evaluation program from earlier. In our last example, our user could try to insert their password an infinite number of times. Let’s impose a restriction which states that users cannot have any more than three attempts:
tries=0 found=false while [[ $found == false ]] do if [[ tries -eq 3 ]]; then echo "You have run out of tries." break fi echo "Insert your password." read password if [[ $password == "test" ]]; then echo "You've entered the correct password." found=true else ((tries++)) echo "Your password is incorrect" fi done
We’ve added a few pieces of code to our while loop. We’ve first declared a variable called “tries” which tracks how many times our user has tried to insert their password.
Within our while
loop we’ve added another if
statement. This checks if a user has tried to enter their password three times. If this is the case, the program will keep running. If a user has tried to enter their password three times, this if
statement will execute.
The message You have run out of tries.
will be printed to the console if the statement executes. Then, our loop will break, which means our program will stop running.
We’ve also added an increment counter ( ((tries++)) )
to the if.
statement that checks our user’s password. This increases the value of tries
by 1 every time a user incorrectly types in their password.
Continue Statement
The continue statement stops one iteration of a while
loop and goes onto the next one. To illustrate this, consider the following example:
count=0 while [[ $count -lt 3 ]] do ((count++)) if [[ $count -eq 1 ]]; then continue fi echo "Count: $count" done
In this program, we’ve created a loop which executes while the value of count
is less than 3. Each time the loop executes, the value of count
is increased by one.
Our program then checks if count is equal to 1. If this is the case, the current iteration of the loop will halt and the next one will begin. Otherwise, the loop will keep running and will print Count:
, followed by the value of “count”, to the console.
Conclusion
While loops allow you to execute the same block of code multiple times. Unlike for loops, you don’t need to instruct a while loop on how many times it should run. A while loop will run until a condition is no longer true.
Now you’re ready to start writing while loops in your bash scripts like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.