How to Write a Bash Function
A function is a block of code that performs a particular task. Once you have defined a function in a program, it can be reused as many times as you want.
Functions are useful because they allow you to reuse code. This enhances the readability of a program. It also means that when you need to make a change to your code, you only need to make that change in one place: where your function is defined.
In this guide, we’re going to talk about what functions are, how they work, and how you can define a function in bash. Without further ado, let’s begin!
Defining a Bash Function
Let’s start by creating a function that prints “I like scones!” to the console.
Open up a new bash file and call it functions.sh. The first step is to define a function. We can do this by using the function
keyword, followed by the name of the function, then followed by a set of curly braces ({}).
Here is our simple bash function:
function print_scones { };
This function currently does nothing. To make our function work, we’ve got to add a statement into its body. The body refers to all of the comments inside a function. Here’s our revised function:
function print_scones { echo "I like scones!"; }
This code only defines our function. This means that if we run our program, nothing will happen. To see our function in action, we’ve got to call our function by specifying its name:
#!/bin/bash function print_scones { echo "I like scones!"; } print_scones
Now, let’s run our script by executing bash functions.sh
:
I like scones!
Our code returns the message that we stated inside our functions. First, our code defines a function called print_scones
. Then, our code calls that function.
Passing Arguments to a Function
Our first function did not accept any arguments. This means that we couldn’t send any values from our main program into our function.
You can send data into a function by specifying a set of arguments when you call a function. Consider the following example:
#!/bin/bash function like_to_eat { echo "I like to eat $1." } like_to_eat scones
In this code, we have declared a function called like_to_eat. This function is capable of accepting arguments. In bash, the arguments passed to a function are assigned the values $1, $2, $3, and so on, depending on how many arguments you specify.
We have specified one argument, which contains the value of the food that we like to eat. To give this argument a value, we need to state it when we call our function:
like_to_eat scones
Let’s run our program:
I like to eat scones.
Our program has substituted $1 for the argument value that we specified, “scones”.
Returning a Value
Bash functions cannot return values to the main program. This is unlike most other programming languages where a return statement sends a value back to the main program.
Bash does have a return
statement, but it is used to retrieve the status of a function.
Consider this example:
#!/bin/bash function like_to_eat { echo "I like to eat $1." return 1 } like_to_eat scones echo $?
Our code returns:
I like to eat scones. 1
We have specified a return statement inside our function. This value is returned only if our function succeeds. Then, in our main program, we use the variable $? to retrieve the return status of the last function or command that was executed. This prints out 1 to the console, which is the return status from our function.
In bash, a return value of 0 typically means that everything is fine. Values between 1 and 255 are used to indicate an error.
There is a work-around if you want to return a value from a function. You can declare a global variable in your function whose value changes when the function executes:
#!/bin/bash function like_to_eat { message="I like to eat $1." } like_to_eat scones echo $message
We have assigned a value to the “message” variable within our function. This value will only be assigned when our function is executed. In our main program, we print out the value of that message. Let’s run our program and see what happens:
I like to eat scones.
Our function works! Technically we’re not returning any values in this example; we cannot return a text value with a return statement. Instead, we are setting a variable inside our function and referencing it inside our main program.
Local and Global Scope
When you’re working with functions, variables can have two scopes: local and global.
A global variable is a variable that is visible anywhere in a program. By default, variables are global. Within a function, you can declare a local variable. This is a variable that is only accessible inside our function.
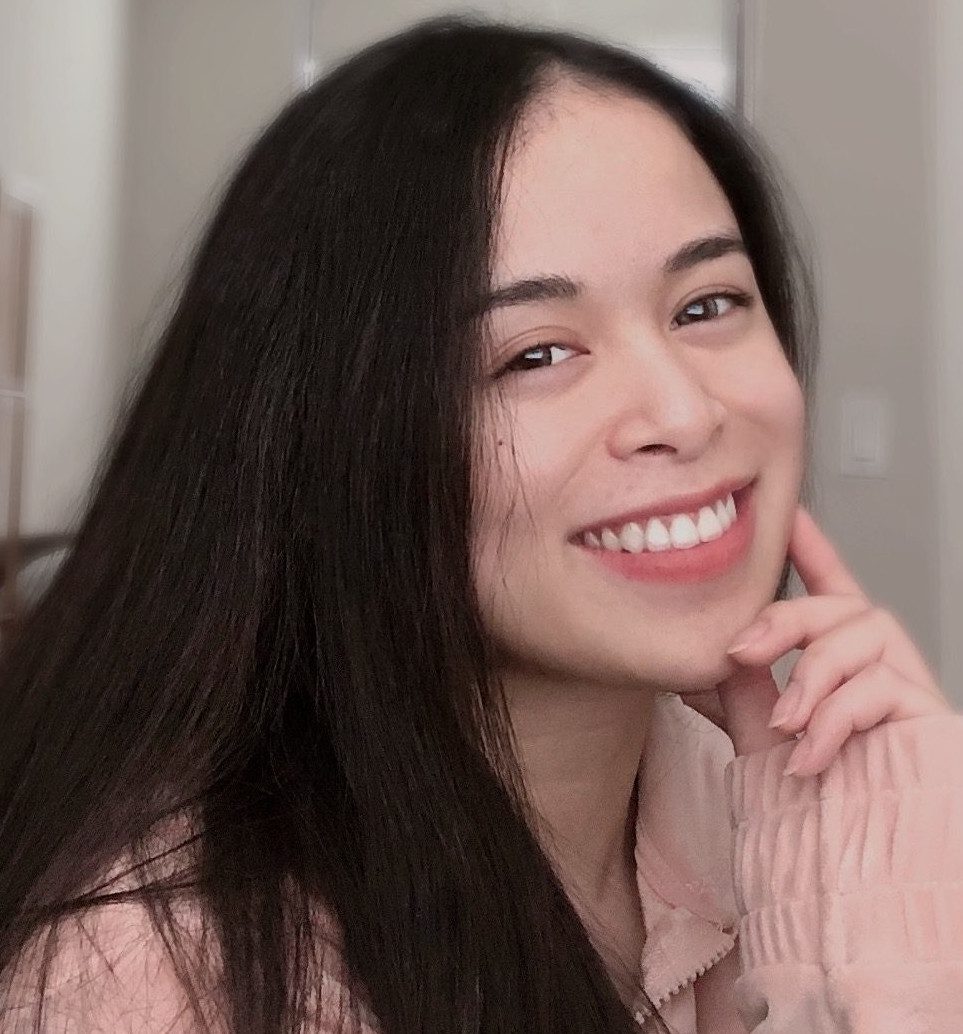
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
To define a local variable, we need to use the local keyword:
function print_scones { local message="I like scones." |
This code assigns the value “I like scones.” to the variable “message”. This variable is only accessible within the print_scones
function.
Create a file called scope.sh and paste in the following code:
#!/bin/bash function like_to_eat { local food=$1 echo "I like to eat $1 (inside function)" } food="scones" echo "I like to eat $food (before function)" like_to_eat pineapple echo "I like to eat $food (after function)"
Our code returns:
I like to eat scones (before function) I like to eat pineapple (inside function) I like to eat scones (after function)
We have used the variable “food” to represent the food that we are printing to the console. In our main program, we set the value of “food” to be equal to “scones”. We then print this food to the console:
I like to eat scones (before function)
Next, we call the like_to_eat function. This function sets the value of “food” to be whatever value we specify as an argument. In this case, we have specified “pineapple” as an argument. The following is then printed to the console:
I like to eat pineapple (inside function)
The value of “food” will only change inside the like_to_eat function. That is because it is a local variable.
Finally, we print out another message stating the value of “food” after our like_to_eat function has been called. The value of “food” is still “scones”. We did change its value inside our function, but we applied the change using a local variable. This means that the value that we changed “food” to is only accessible in our function.
The final statement in our code returns:
I like to eat scones (after function)
This statement is run after our function, but the value of “food” is still “scones”. That’s because we set the value of “food” to “scones” as a global variable.
You should always try to use local variables within functions. This will help improve the readability and maintainability of your code.
Conclusion
Functions are blocks of code that perform a particular action within a program. They are useful because they help reduce repetition because you can bundle your code into blocks that can be called multiple times.
Now you’re ready to start working with bash functions like an expert developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.