After using the command line for a while, you will start to notice that there are a lot of commands that you repeat often.
Some of these commands, like “cd” and “ls” are just part-and-parcel of working with the Linux command line. However, some commands are longer and require more thought. If you’re looking to save time when typing commands, bash aliases are worth a look.
In this guide, we’re going to talk about what bash aliases are, why they are used, and how you can create your own bash alias. Let’s get started!
What is a Bash Alias?
A bash alias is a shortcut that executes a longer command.
One way to think about a bash alias is like it was a speed dial number. Speed dial numbers are shortened codes you can use to get in touch with your friends and family.
You don’t need to remember their entire phone numbers or search through your address book to call someone you call often: you just need to dial their speed dial number.
Bash aliases are useful because they help save time. Many Linux commands are already short (like how “cd” is short for “change directory”) but if you’ve got long commands to write, alises can be incredibly helpful.
Aliases can help you reduce the risk of error when you are working in the command line. All you need to do is define a command once and you can use it many times. This means that you’re less likely to make a typo which affects your command.
You should avoid using aliases for commands that you want to review before execution, such as commands that destroy files. This will give you a chance to think about whether you want to run a particular command before you execute it.
How to Create a Bash Alias
Let’s create an alias. For this article, we’re going to create an alias for the always-confusing tar command. We’re going to create an alias which shortens this command:
tar -xvf
While this command isn’t too long, it can be hard to remember what it means. If you extract files often, then having an alias to help you do it will save you a lot of time.
Our alias for this command is going to be tarx
. This stands for “tar extract”. When we use the tarx
alias, we’ll not need to write out tar -xvf
.
Let’s write an alias called tarx:
alias tarx=“tar -xvf”
This command declares our alias. This alias will last as long as our Linux session. Now, let’s type in tarx and specify a file to open:
tarx tutorials.tar.gz
This command opens up the tutorials.tar.gz
file.
Aliases do not persist after sessions. This means that if you find yourself using a command often across multiple different sessions, you’d have to run the “alias” command every time you open a session. This is impractical.
We can make aliases persistent by adding it to the ~/.bashrc file
. This file contains the commands which are run whenever an interactive bash shell is opened. Open up the ~/.bashrc
file and add the following contents:
# Aliases alias tarx="tar -xvf"
This alias is not yet available in our session. We’ve got to refresh our bash session. We can do this using the source command:
source ~/.bashrc
Now if we try to run our alias it will work. Every time we open a terminal session, we’ll be able to run this alias.
Using Functions with a Bash Alias
In our last example, our alias referred to a command that was one line long. This serves most use cases, but not all of them.
There are two main cases where using the syntax from the last example does not work. When you need to accept arguments into the middle of a command, you cannot use the “alias” syntax we discussed earlier. Similarly, if you want to run multiple commands using one alias, you cannot use a single “alias” keyword.
That’s where functions come in. We can declare a function which allows us to run multiple commands under one alias. Functions can accept arguments so we can pass data into the middle of our commands.
Let’s create a function that creates a file called .gitignore and adds *.pyc
to the file:
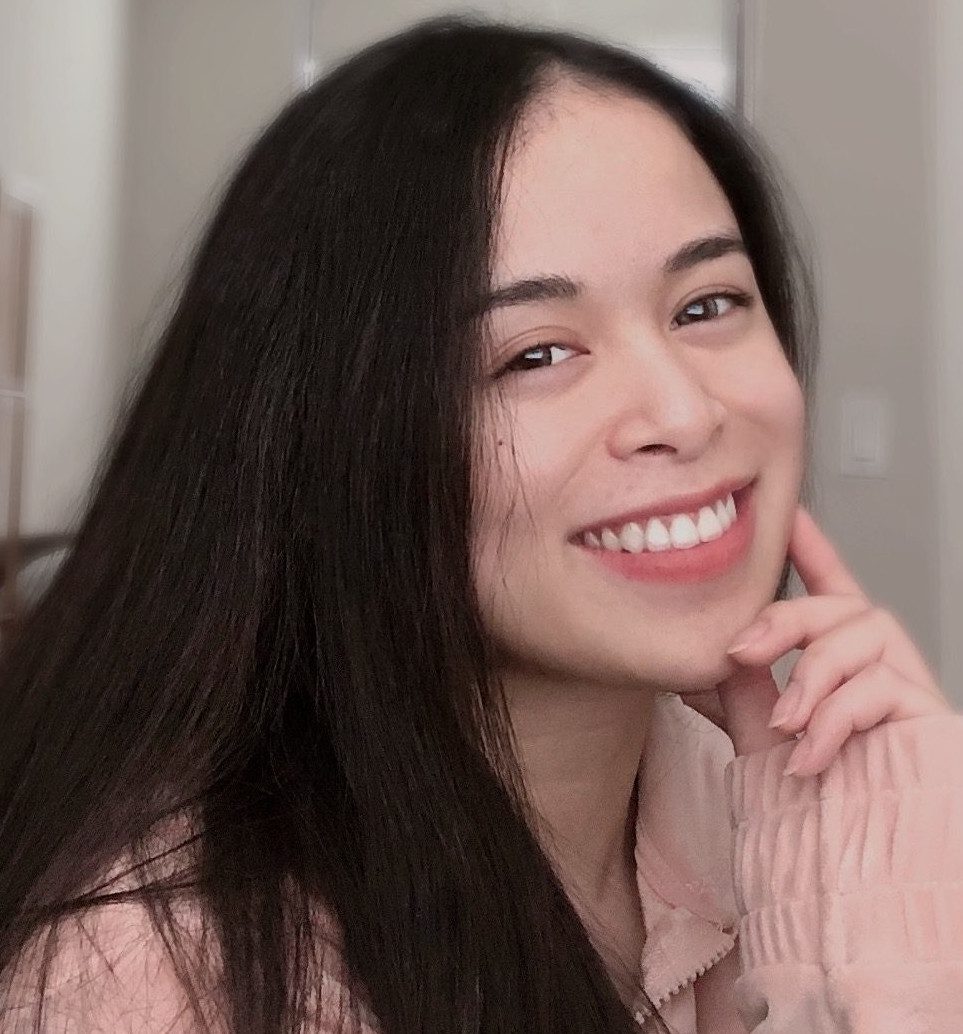
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
gip () { touch .gitignore echo "*.pyc" >> .gitignore }
We have created an alias called gip
, which is short for git ignore Python
. This alias sets up a gitignore
file for a Python project.
This alias executes two commands. Our alias first creates a file called .gitignore
in the user’s current working directory. It then adds the text *.pyc
to the end of the gitignore
file.
To make our function work as an alias, we need to add it to the ~/.bashrc
file:
# Aliases alias tarx="tar -xvf" gip () { touch .gitignore echo "*.pyc" >> .gitignore }
Our bash session now has two aliases: tarx and gip. We need to run source ~/.bashrc
to reload the file so the alias will be accessible in our current session. Now our commands are ready for use!
We haven’t accepted any arguments into our function just yet. Let’s say that we want our gip
command to accept one argument: the directory in which the .gitignore
file should be created. Right now, the .gitignore
file is created in the user’s working directory.
We could accept an argument using the $ parameter keywords. These keywords correspond to the position of a parameter after a function name. $1 gets the first parameter, $2 gets the second parameter, and so on.
Let’s create the code that accepts our parameter:
gip () { touch $1/.gitignore echo "*.pyc" >> $1/.gitignore }
When we run gip, the first argument we specify will correspond to the directory in which we want our .gitignore file to appear. Consider this command:
gip /home/career_karma/python_tutorials/
This will create a .gitignore
file in the /home/career_karma/python_tutorials/
directory.
The trouble with our alias as it is is that if we do not specify a directory in which to place our file, $1 will not have a value. We could add an if
statement into our alias which checks if $1 is present. If $1 is present, a file called .gitignore
is added to that folder; otherwise, .gitignore
is added to the user’s current working directory.
Add the following code to your gip alias:
gip () { if [ -n $1 ]; then file = $1 else file = "." fi touch $file/.gitignore echo "*.pyc" >> $file/.gitignore }
This code checks if $1 is present using -n $1. If it is, the value of file
is set to the value that the user has specified; otherwise, file
is set to “.”, or the user’s current working directory.
We use the $file
variable to specify the directory to which the .gitignore
file should be added.
Let’s try out our command without any parameters:
gip
This command adds a .gitignore
to our current working directory.
Conclusion
Aliases can help you make better use of your time in the shell. Aliases allow you to write shorter versions of commands that you use often. This means that you don’t have to write the long-form versions of a command every time you use it. Once an alias is declared, you can use it as many times as you like in your bash shell.
Now you’re ready to start working with bash aliases like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.