When you’re building a web application, there may come a time when you want to perform a HTTP request to access an external resource. For instance, suppose you are making a blog. You may want to call an API to retrieve a list of comments to show on each blog post.
Axios is a popular JavaScript library you can use to make web requests. In this guide, we’ll explain how to use axios to make a GET request. We’ll walk through a few examples to showcase how axios works and how you can use it in your code.
Why Axios?
Axios is a promise-based library that makes it easy to make web requests.
You may be wondering: why should I use axios over one of the many other web request libraries out there? It’s true that there are other libraries like fetch that you can use to make GET requests, but axios has a number of advantages these libraries don’t have.
Axios supports older browsers, which will allow you to create a more accessible user experience. Axios also comes with built-in CSRF protection to prevent vulnerabilities. It also works in Node.js, which makes it great if you’re developing both front-end or back-end web applications.
How to Install Axios
Before you make a GET request using axios, you’ll have to install the library. You can do so using the following command:
npm install axios --save
This command will install axios and save it to your local package.json file. Now you’re ready to start using the axios library.
How to Make a Request Using Axios
Getting started with axios is simple. To make a web request, all you need to do is specify the URL from which you want to request data and the method you want to use.
Suppose you want to retrieve a list of random facts about cats from the cat-facts API. We could do so using this code:
axios ({ url: "https://cat-fact.herokuapp.com/facts", method: "GET" )}
This code returns a promise which represents a request that hasn’t been completed yet. In order to retrieve data from this HTTP request, you would use an async/await function like so:
const axios = require("axios"); async function getCatFacts() { const response = await axios ({ url: "https://cat-fact.herokuapp.com/facts", method: "GET" }) console.log(response.data) } getCatFacts()
The server responded with a list of cat facts.
When we call this function, an HTTP GET request is made to the cat-facts API. We use an async/await function so our program does not continue until the web request has been completed. This is because axios returns a promise first. It returns the data from the request it makes after that request has been completed.
The axios library comes with a few shorthand commands you can use to make web requests:
- axios.get()
- axios.post()
- axios.delete()
- axios.put()
- axios.patch()
- axios.options()
In this tutorial, we’re going to focus on the axios.get()
and axios.post()
methods, which use the same basic syntax as all the other shorthand methods.
Making GET Requests Using axios
In our last example, we used axios to make a GET request. But, there is a simpler way to make a GET request with axios: using axios.get()
.
Suppose you want to retrieve a list of cat facts, then count how many have been returned. You could do so using the following code:
const axios = require("axios"); async function getCatFacts() { const response = await axios.get("https://cat-fact.herokuapp.com/facts") console.log(`{response.data.all.length} cat facts were returned.`) } getCatFacts()
The code generated this response: 225 cat facts were returned.
Let’s break down our code. We have first declared an async function called getCatFacts()
in which we make a web request.
We then use axios.get()
to retrieve a list of cat facts from the cat-facts API; “response.data” holds the response objects and request body from our request.
Finally, we use the .length attribute to calculate how many cat facts have been returned from our request. We then add this number into the string “cat facts were returned”.
Sending Headers Using axios
When you’re making a GET request, you may need to send a custom header to the web resource to which you are making the request. Suppose you are retrieving data from an API that requires authentication. You may need to specify an authentication header.
To specify a header with an axios request, you can use the following code:
axios.get("https://urlhere.com", { headers: { "header-name": "header-value" } })
This code would send the header “header-name” with the value “header-value” to the URL we have specified.
Sending Parameters Using axios
Many APIs allow you to send parameters in a GET request. For instance, an API may allow you to limit how many responses are returned using a limit parameter.
Specifying a parameter to send with a web request is easy using axios. You can either include the parameter as a query string, or use the params property. Here’s an example of axios making a web request using query strings to specify a parameter:
axios;get("https://urlhere.com/?date=2020-05-15")
Alternatively, you could specify a params property in the axios options using this code:
axios;get("https://urlhere.com/?date=2020-05-15", { params: { date: "2020-05-15" } })
Both of these examples send a parameter with the name “date” and the value “2020-05-15” to the specified URL.
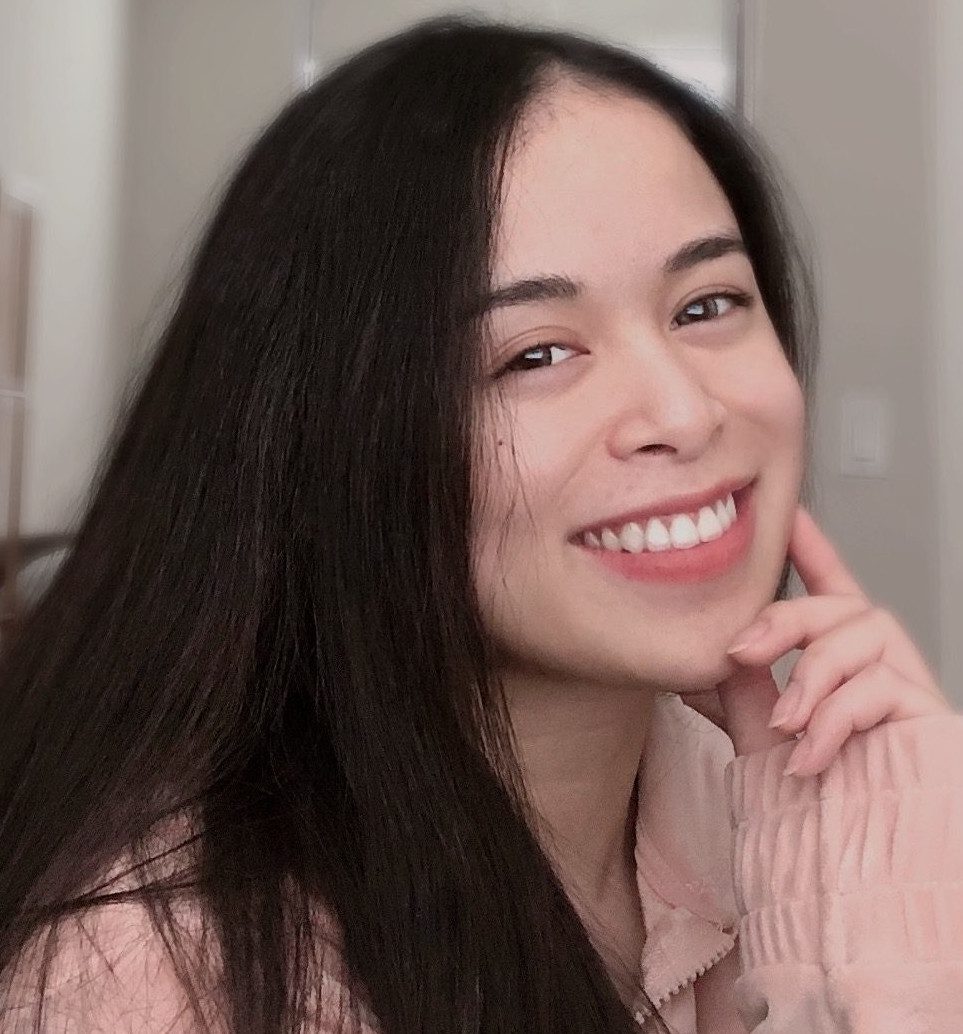
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
How to Make a POST Request Using axios
The syntax for making a POST request is the same as making a GET request. The difference is you should use the axios.post()
method instead of axios.get()
.
Suppose you want to make a post request to an API. You could do so using this code:
axios.post("https://urlhere.com")
You’re able to specify headers and parameters in the same way as you would to make a GET request. Let’s say you want to send the header “Name” with the value “James” with your POST request. You could do so using this code:
axios.post("https://urlhere.com", { headers: { "Name": "James" } })
Conclusion
The axios library is used to make web requests in JavaScript. It can be used on both the front-end using JavaScript or on the back-end using a platform like Node.js. Unlike other web request libraries, axios has built-in CSRF protection, supports older browsers and uses a promise structure. It’s perfect for making web requests.
Now you’re ready to start making GET and POST requests using axios like a professional web developer.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.