Are you tired of having to declare the size of an array before you can use it? You’re not the only one, as it’s not always convenient to have to define how many values an array should store before you can start adding values to that array.
That’s where our helpful friend, the Java ArrayList, comes in.
In this guide, we’re going to talk about what ArrayLists are, how they work, and how they compare to traditional arrays. We’ll also walk through the most important methods that you need to know about in order to work effectively with the ArrayList class. Let’s begin!
What is an ArrayList?
An ArrayList is a special type of list that allows you to create resizable arrays. The ArrayList class implements the List interface, which is used to store ordered data.
When you’re working with an array in Java, you’ve got to declare the size of that array before you can store values within that array. It’s often the case that you don’t know how many values you’ll want to store in an array before you declare it, which presents a problem.
The ArrayList class allows you to define an array that doesn’t need to be told how many values it can store. ArrayLists, which are sometimes referred to as dynamic arrays
, can change their capacity when you add or remove elements.
How to Create an ArrayList
Let’s get started in the obvious place: declaring an ArrayList. Setting one up is quite different to how you’d declare an array, because it uses the Java List interface.
Open up a Java file and paste in the following code into your main class:
import java.util.ArrayList; ArrayList<String> songs = new ArrayList<>();
We’ve just created an array list called songs
. String
refers to the type of data that will be stored in our array and ArrayList refers to the type of object we want to create.
We’ve got to import ArrayList into our code because it comes from the Java util
library.
It’s worth noting that you cannot create array lists using primitive data types. If you want to declare an array of integers, for instance, you can’t use the primitive int
type; you’d need to use the Integer class.
Notice that we didn’t have to specify how many values our ArrayList needs to store. That’s because ArrayLists
are dynamic.
How to Add Elements to an ArrayList
The add() method lets you add a single element into your list. Let’s say that we want to add in two songs to our songs
array list: Love Me Do and Help! (which are both by The Beatles).
Open up a new Java file and write in the following code:
import java.util.ArrayList; class Main { public static void main(String[] args) { ArrayList<String> songs = new ArrayList<>(); songs.add("Love Me Do"); songs.add("Help!"); System.out.println("Songs: " + songs); } }
Our code returns: Songs: [Love Me Do, Help!]
Like arrays, items in an ArrayList
are given their own index numbers. This is because items in an ArrayList are ordered and the numbers tell our program in which order each item in our list should appear. If needed, you can specify the index position to which you want to add an item:
songs.add(0, “Love Me Do”);
This will add the entry “Love Me Do” at index position 0 to our songs
list.
Initialize an ArrayList
When you declare an array, you can initialize it directly by specifying the values that you want to assign to that array. ArrayLists
don’t work in this way. You’ve got to use a special method called asList() from the Arrays class to initialize an ArrayList. asList()
returns an array as a list that can be read by the ArrayList class.
You may want to initialize an ArrayList with values if you’ve already got a few values that you want to add to your list.
You could use a for loop to add each item individually. However, that approach is more complex to set up and less efficient than initializing the list with values using asList()
.
Let’s get started by importing the libraries we are going to use in our code:
import java.util.ArrayList; import java.util.Arrays;
We’ll then write a class which initialized our songs ArrayList with two values:
class Main { public static void main(String[] args) { ArrayList<String> songs = new ArrayList(Arrays.asList("Love Me Do", "Help!")); System.out.println("Songs: " + songs); } }
In this code, we’ve declared an ArrayList
that can store string values. We’ve used asList() to instruct our code to initialize our array list with two default values, which are the same Beatles songs that we added in our last example.
Retrieve an Item from an ArrayList
The ArrayList class comes with a handy method called get()
that allows you to access the elements of an array list. To use this method, you’ve got to specify the index position of the element that you want to access.
Consider this example:
import java.util.ArrayList; class Main { public static void main(String[] args) { ArrayList<String> songs = new ArrayList<>(); songs.add("Love Me Do"); songs.add("Help!"); System.out.println("Second song: " + songs.get(1)); } }
In this code, we’ve created an array list and added two values. We’ve then used songs.get(1)
to retrieve the song at the index position 1 in our list. Our code returns:
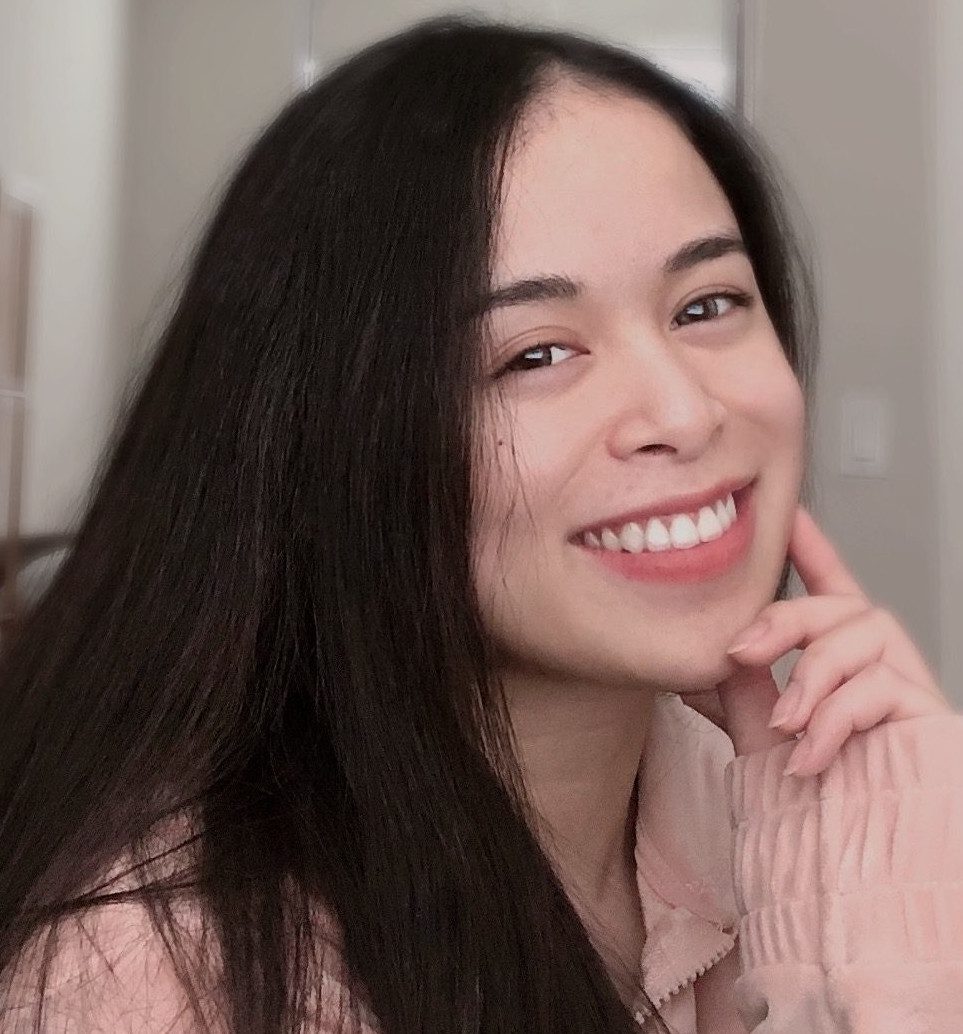
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Second song: Help!
Update an ArrayList
Updating an array list is as easy as adding elements to the list. There’s a built-in method called set()
that you can use to change the value stored in a list. Consider the following code:
import java.util.ArrayList; class Main { public static void main(String[] args) { ArrayList<String> songs = new ArrayList<>(); songs.add("Love Me Do"); songs.add("Help!"); System.out.println("Songs: " + songs); songs.set(1, "Come Together"); System.out.println("Songs: " + songs); } }
Our code returns:
Songs: [Love Me Do, Help!]
Songs: [Love Me Do, Come Together]
In our code, we’ve added two values – “Love Me Do” and “Help!” – to our list. We’ve then changed the item with the index position 1 to “Come Together”. This replaces “Help!” with “Come Together” in our list. When we print out our list at the end of the program, we can see that it has been revised.
Remove an Element from an ArrayList
Elements don’t have to stay in an ArrayList forever. You can remove an element using the remove()
method at any time. What an easy method name to remember!
Consider this example:
import java.util.ArrayList; class Main { public static void main(String[] args) { ArrayList<String> songs = new ArrayList<>(); songs.add("Love Me Do"); songs.add("Help!"); System.out.println("Songs: " + songs); String removed = songs.remove(0); System.out.println("Songs: " + songs); System.out.println(removed); } }
In this example, we add two items to our array list. We then remove the item with the index position 0. Our code returns:
Songs: [Love Me Do, Help!]
Songs: [Help!]
Love Me Do
We’ve just removed Love Me Do
from our array list. The remove()
method returns the name of the item that has been removed, which we assigned to the variable removed
. We printed out the value of this variable to the console at the end of our program.
Iterate Through an ArrayList
What if you want to loop through an ArrayList? You can do that using either a for loop or a for-each loop, depending on your preference.
Let’s say that we want to print out all the Beatles songs in our list to the console, with each value appearing on a new line. We could do so using the following code:
import java.util.ArrayList; class Main { public static void main(String[] args) { ArrayList<String> songs = new ArrayList<>(); songs.add("Love Me Do"); songs.add("Help!"); for (int i = 0; i < songs.size(); i++) { System.out.println(songs.get(i)); } } }
In this program, we’ve used a for loop to iterate through every item in our array list. For each item in the array list, we print it out to the console on its own line. This loop continues until every item in our list has been printed to the console.
Notice that we’ve used a method called songs.size()
in this program. The size() method tells us how many values are stored in our array list.
Our code returns:
Love Me Do
Help!
Similarly, you can use a for-each loop to iterate through an array list. This is because for-each loops support iterating through any iterable object. Consider the following example:
import java.util.ArrayList; class Main { public static void main(String[] args) { ArrayList<String> songs = new ArrayList<>(); songs.add("Love Me Do"); songs.add("Help!"); for (String song : songs) { System.out.println(song); } } }
This program is very similar in both structure and purpose; it prints out all the values in our songs
array list to the console. The difference is that we’ve used a for-each loop to iterate through each value.
You may opt to use a for-each
loop instead of a for
loop because it is easier to read a for-each loop, but both methods work effectively.
Conclusion
The Java ArrayList class allows you to define arrays which can store multiple values without being told how many values to store upfront.
We’ve only scratched the surface of using ArrayLists in Java. To learn more, you may want to view the following tutorials:
Now you no longer have to worry about what you’ll do if you don’t know how many values an array will need to store. You’ll be able to use ArrayList to solve this problem!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.