The JavaScript appendChild() method adds an item to the end of a node. appendChild() is often used to add <li> items to a list. You must use multiple appendChild() statements if you want to add more than one item to a node.
How to Use JavaScript appendChild
Some lists contain text; other lists contain images; other lists contain custom web elements. Whatever is in a list, one thing is certain: web pages are full of lists.
When you’re creating a list, you will usually hardcode its values using HTML. This process can be sped up using a method called JavaScript appendChild. This method allows you to add an item to the end of a list or another web element, such as a blockquote.
In this guide, we’re going to talk about what appendChild() in JavaScript is and how it works. We’ll walk through an example to help you get started. Let’s begin!
What is JavaScript appendChild?
appendChild() adds a node to the end of a parent node. appendChild() is commonly used to add items to a HTML list. A node refers to any item in the HTML Document Object Model (DOM).
The syntax for appendChild() is like this:
parent.appendChild(child);
“parent” refers to the element to which you want to add a child. “child” is the element you want to append to the bottom of the parent.
You need to use a method like JavaScript getElementById() or another “getter” to retrieve an element. Or, you can create a HTML element in JavaScript and then use that element as the “parent” object with appendChild().
For instance, a list of scone recipes would contain a list of nodes. These nodes would probably be <li> tags because we would be creating a list.
A parent <div> which contains <img> tags would contain child <img> nodes. A common use of appendChild()is to add <li> tags to a list such as a <ul> or <ol>.
appendChild() JavaScript: Example
Let’s create an application that uses appendChild. We’re going to create a site which loads a list of the names of students in a tenth grade class. We’ll break this down into three steps:
- Setting up a front end
- Selecting and creating the elements
- Using appendChild
Setting Up a Front End
First, we’ll set up a basic front end that shows our list of student names. Open a file called index.html and paste in this code:
<!DOCTYPE html> <html> <head> <title>Tenth Grade Class Roster</title> <link rel="stylesheet" href="styles.css"> </head> <body> <h1>Tenth Grade Class Roster</h1> <ul> </ul> </body> <script src="scripts.js"></script> </html>
We’ve created a basic HTML document. This document contains a title and a list which have no child items. The list is represented with the <ul> tag.
Next, let’s add a few styles to our page in a file called styles.css to improve the aesthetics of our web page:
body { background-color: #f7f7f7; margin: auto; width: 50%; }
This CSS rule will set a light gray background color for our page. We do this using the CSS background-color property. It will also move the contents of the <body> tag into the center of the page. To learn more about how we structured the layout, check out our CSS box model guide.
Our page is not functional yet. We haven’t added any list elements. Here is what our page looks like when you open it up in a browser:
Clearly there is still work to do. Let’s add JavaScript into our web page.
Selecting and Creating the Elements
Before we can start adding items to our list, we need to select the elements with which we are going to work. Open up a file called scripts.js and add this code:
const students = document.querySelector("ul");
This line of code will select the item on our page with the tag <ul>. This represents our list. Next, we’re going to create an element to add to our list:
let li = document.createElement("li"); li.textContent = "Steven";
This code creates a text node with an <li> tag which contains the word “Steven”. Now all that’s left to do is add our newly created element to our list.
Using The appendChild() JavaScript Method
appendChild() allows us to add elements to a node. Add the following code to the bottom of your scripts.js file:
students.appendChild(li);
This will add the list item we created earlier to our list. Let’s open up our web page and see the results:
Our list now has one item. We can make our code more efficient by creating a function that adds items to our list. This will allow us to add multiple items without having to repeat our createElement code from earlier.
Change the createElement and textContent lines of code to this:
function createStudent(name) { let li = document.createElement("li"); li.textContent = name; return li; }
This function will create a new <li> element whenever it is called. Then, change the appendChild() statement to use this function:
students.appendChild(createStudent("Mark")); students.appendChild(createStudent("Chloe")); students.appendChild(createStudent("Louise"));
This will create three students: Mark, Chloe, and Louise. Let’s open up our index.html file again and see whether our changes have been made:
Our class roster now has three names.
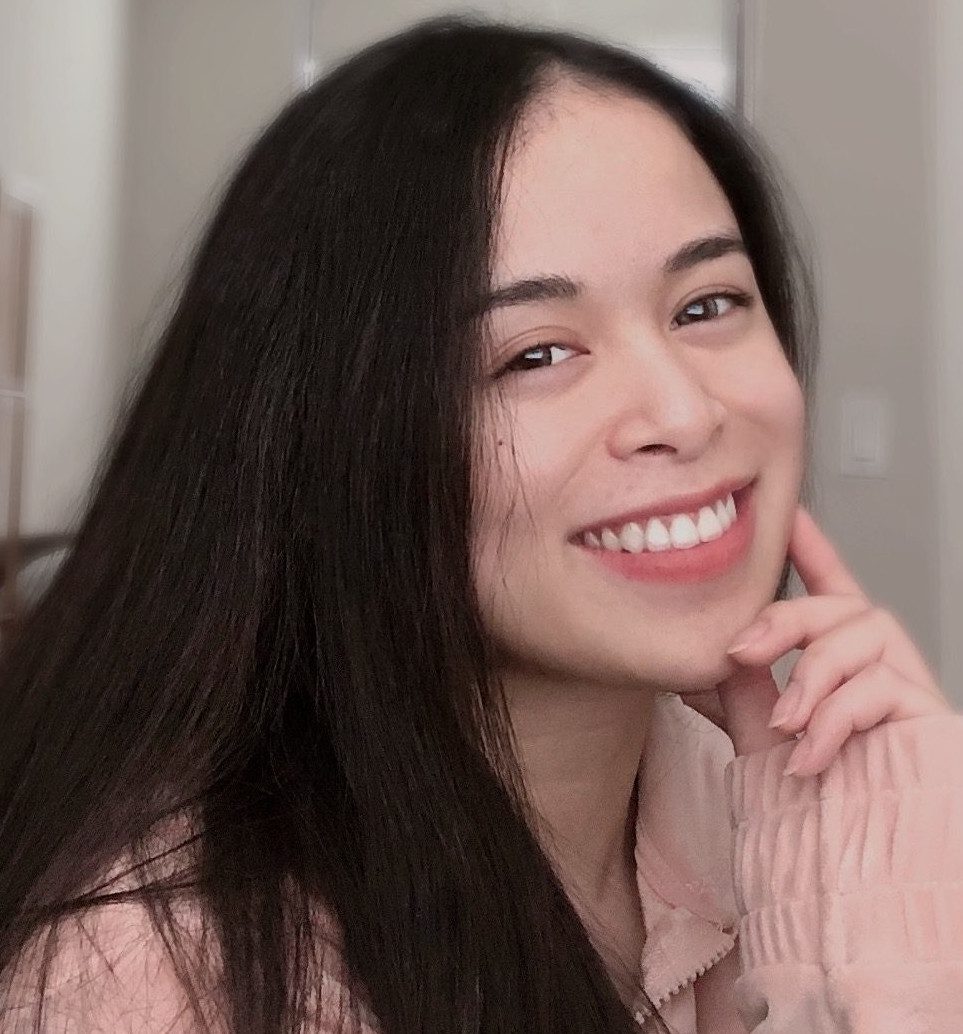
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Move Items to a Different List
You can move items to a different list using the appendChild() method. Let’s show this off by amending our example from above. First, let’s change our HTML page so that we have two lists:
… <body> <h1>Tenth Grade Class Roster</h1> <h3>Sat Test</h3> <ul id="sat_test"> </ul> <h3>Has Not Sat Test</h3> <ul id="has_not_sat_test"> </ul> </body> ...
We have created two lists: sat test and has not sat test. Now, let’s update our JavaScript file to select our two lists and create three students:
const sat_test = document.getElementById("sat_test"); const has_not_sat_test = document.getElementById("has_not_sat_test"); function createStudent(name) { let li = document.createElement("li"); li.textContent = name; return li; } has_not_sat_test.appendChild(createStudent("Mark")); has_not_sat_test.appendChild(createStudent("Chloe")); has_not_sat_test.appendChild(createStudent("Louise"));
We use the getElementById method to select our two lists.
This code adds Mark, Chloe, and Lucy to the “has not sat test” list. Louise has just sat her test, and so she can be moved into the other list. We can move her name using the appendChild() method and another method called lastChild:
var louise = has_not_sat_test.lastChild; sat_test.appendChild(louise);
This code selects the last DOM element in our “has not sat test” list. This item is retrieved using the lastChild method. Next, we have appended this item to our “sat test” list.
Let’s open up our HTML file:
We have two lists: sat test and has not sat test. Initially, we move all student names into the “has not sat test” list. Then, we use appendChild() to move Louise’s name into the “sat test” list.
Conclusion
The appendChild() method is used to create a node at the end of an element. You can use appendChild() to add any element to a list of elements. For instance, you could add a <div> element to a <section> tag.
As a challenge, try to see if you can use appendChild() JavaScript method to create a grid of images. When a page loads, it should render a list of four images using appendChild().
For guidance on top JavaScript online courses and learning resources, check out our How to Learn JavaScript guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.