JavaScript is integral to a better Internet experience as it makes responsive design smoother and easier. It’s commonly used in content management systems, phone apps, social media platforms, ecommerce platforms, and search engines. Developers use this programming language to add complexity and interaction to their applications.
If you want to pursue a career as a web developer or software engineer, it is important to learn JavaScript as well as its best practices and guidelines. This comprehensive article will highlight JavaScript best practices, guidelines, and resources for your web development career.
What Is JavaScript?
JavaScript is a programming language that is known for its lightweight capabilities. It is used for creating dynamic pages because it allows the client-side script to interact with the user side. This programming language also features object oriented capabilities aside from its client-side scripting capabilities and easy component testing.
JavaScript includes many different technical aspects, including intermediate variables, descriptive names, arrow functions, and class syntax. If you want to learn JavaScript, you’ll need to become more familiar with all of these concepts and more. You will also need to know how to find duplicate code and conduct code reviews.
Coding Concepts You Need to Understand for JavaScript Best Practices
To thrive as a developer, you need to understand some basic concepts in JavaScript. These basic practices guide all developers in their work. Some of them include closures, scope, hoisting, callbacks, and promises.
- Closures. Closures use inner functions to give you an idea of an outer function’s scope. Functions can include generic helper functions and asynchronous functions.
- Scope. The scope is a box with boundaries for functions, variables, and objects. The boundary restricts variables and decides if you can access variables or not. Also, it limits the availability or visibility of a variable. Developers need to understand this concept to be able to separate logic in a code and improve its readability.
- Hoisting. Another important concept for JavaScript is hoisting. It is a default behavior of moving declarations before code execution. It is useful because no matter where variables and functions are declared, they move to the top of the scope whether the scope is local or global.
- Callbacks. This concept ensures that functions do not run before the completion of the task. This is how developers create asynchronous JavaScript code. It also keeps them safe from errors and problems.
- Promises. This concept is used to execute more than two back-to-back operations in asynchronous JavaScript operations. With promises, a certain object may produce a single value in the future.
5 Common Challenges That JavaScript Guidelines Can Address
JavaScript has some impressive features that make it stand out among programming languages. Its guidelines address several challenges including web server creation, graphics, network apps, slow app responses, and support for external apps. Below are five things that JavaScript best practices can help improve.
Web Server Creation
JavaScript is useful for creating web servers. Since it is event-driven, it reacts to user interaction. Servers developed with JavaScript are quite fast. They don’t use buffering, and they make it easy to transfer large chunks of data. Also, JavaScript is single-threaded and features event looping.
Graphics
JavaScript is also useful in creating games. The Ease.js library offers seamless solutions for creating rich graphics. Its combination of HTML5 and JavaScript makes it popular for game development. JavaScript allows users to create a stage where they can render a display list to a target canvas.
Network Apps
JavaScript eases the process of building network apps. It is used to develop scalable and fast network applications because of its efficient, lightweight, and event-driven features. It’s also used to generate content and handle HTTP requests. If a user writes thick applications using JavaScript, it is possible to write the logic on the JavaScript server to allow cognitive leaps.
App Response Time
JavaScript is useful in making app responses faster. One example of this application is Google Maps, where clicking and dragging the mouse will give you a detailed view of the map. JavaScript makes this possible by interacting with the browser without having to send messages to the servers.
Support for External Apps
JavaScript is preferred by some because it supports external applications like running widgets, PDF documents, and flash applications. Also, JavaScript can load content into documents when the user needs it without having to reload the page. This standalone language is used to add special effects to web pages such as roll-outs, rollovers, and several other kinds of graphics.
Top 10 JavaScript Best Practices and Guidelines
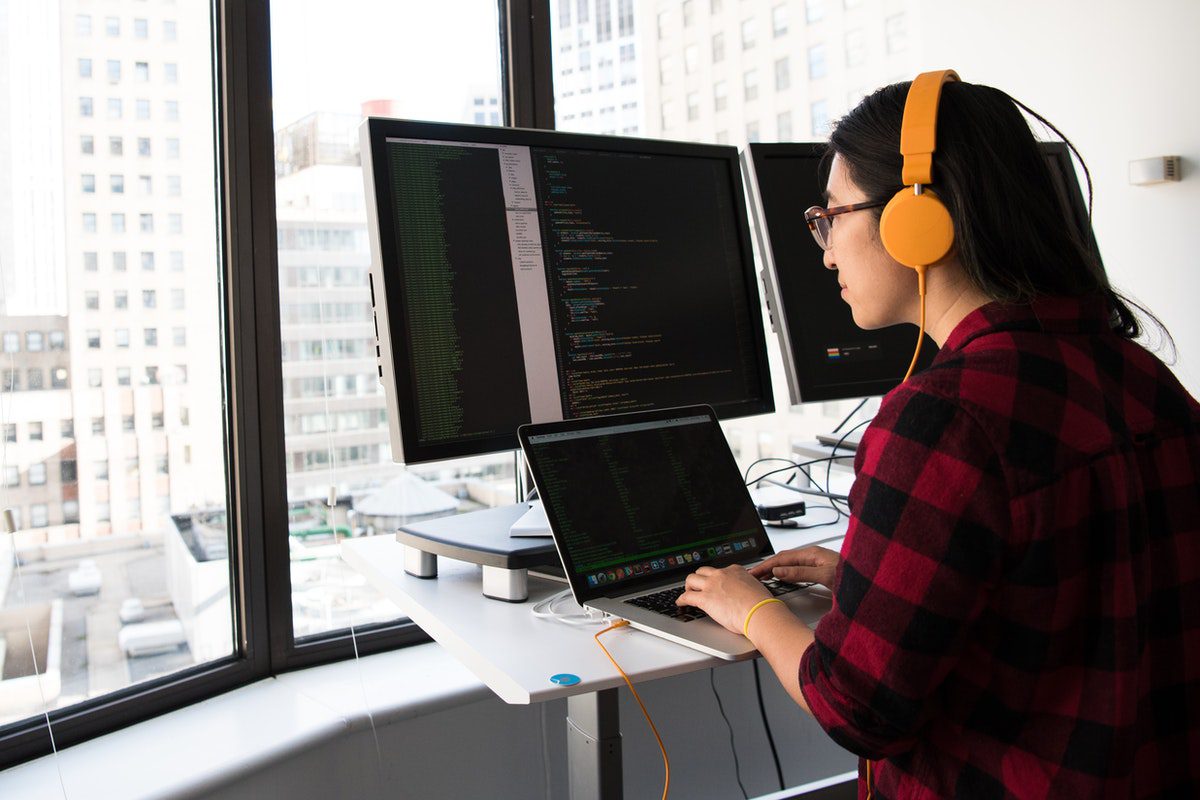
Best practices are often recommended by experts who have tried different methods and identified the common mistakes developers make in the field. Some of the most common JavaScript best practices and guidelines include using raw JavaScript over libraries, placing scripts below the page, organizing declarations, and choosing promises over callbacks.
Place Scripts Below the Page
The main goal here is to ensure that your page loads fast for the user. While loading a script, browsers cannot continue unless the entire file is loaded. This is why the user may have to wait before they can view an entire web page. Add the scripts at the bottom of the page before the body tag to help solve this issue.
Use Raw JavaScript
JavaScript has libraries that contain pre-written JavaScript codes, making it easier to develop applications. Some common JavaScript libraries like Lodash and jQuery can come in handy while coding as they save you time with AJAX operations. However, it is still faster to use raw JavaScript code than a library as long as you can code correctly.
Avoid Using “Undefined”
When something is tagged “undefined,” it means it lacks value. Some developers do this, but the problem is that JavaScript already adds “undefined” items to the code. This makes it difficult to determine if something is tagged “undefined” because of JavaScript or the programmer. Instead of using “undefined,” use the term “null.”
Organize and Initialize Declarations
It helps to maintain consistency when declaring things in JavaScript. Start by putting your declarations at the top from constants to variables. Ensure that the constants are added in uppercase to make it clear they are constants and other developers do not try to change them.
This is why it is not ideal to add “undefined” to declarations as a value. It is also not ideal to leave them without any value because they are already “undefined” automatically. To be safe, if you can avoid declarations, do not use them. When you have too many declarations, it may also give other developers a hint that you are not skilled in your profession.
Use Comments
Comments are used for several reasons, but the most important one is to explain something that is abnormal, uncommon, or requires some context for proper understanding. You can also use comments to indicate where something needs to be fixed in the future.
Comments can also be added to modules in a codebase explaining the intention or architecture behind the code. Even if it is a short three-letter name, use something with meaning to reduce the burden on other developers.
Opt for Promises Over Callbacks
Promises are JavaScript objects that link the consuming code and the production code together. The production code starts by taking time to produce the promised result. The promise then becomes available to the subscribed code when it is ready. Promises are preferred over callbacks for many reasons.
For one, promises are quite easy to use. Also, callbacks can be “promisified” and are mainly used to call when something is done. It doesn’t matter if it is synchronous or not. Since JavaScript is single-threaded, using promises is better because it increases code readability.
Avoid Chaining Loops and Unreadable Hacks
Chaining loops and chain iteration methods increase code complexity. This can present problems because it will slow things down when your data grows. In some cases, chaining loops may be required, but it is ideal to avoid the practice entirely. Be sure to assess your looping strategy because it helps you to determine if there are combined loops or unnecessary loops.
Unreadable hacks are also not ideal because they are abnormal and unconventional. Many developers add them, but it is better to follow the best practices of any tool you are using. Therefore, unless it is necessary, do not chain loops or use unreadable hacks.
Add Semicolons
Semicolons are essential in most programming languages, and this is also the case for JavaScript. The compiler reads semicolons to be able to distinguish between statements so that other statements will not leak into parts of the code where they are not needed.
In some cases, you may be able to avoid adding a semicolon in your JavaScript code. This is because the compiler adds semicolons. The problem with it is that some tools may misread your code easily and this could also lead to a bug making it to production. Use semicolons only when necessary.
Check Automatic Type Conversions
Always check automatic type conversions because you may end up with something you didn’t intend to create. In most cases, “falsy values” turn false while “truthy” values turn true. With numbers, “falsy values” turn to “zero” while “truthy” turns to “one.”
While working with data from an API or user you are not sure of, ensure that it is in the right format and type before starting to work on it. Check the conversions and reduce your overall workload.
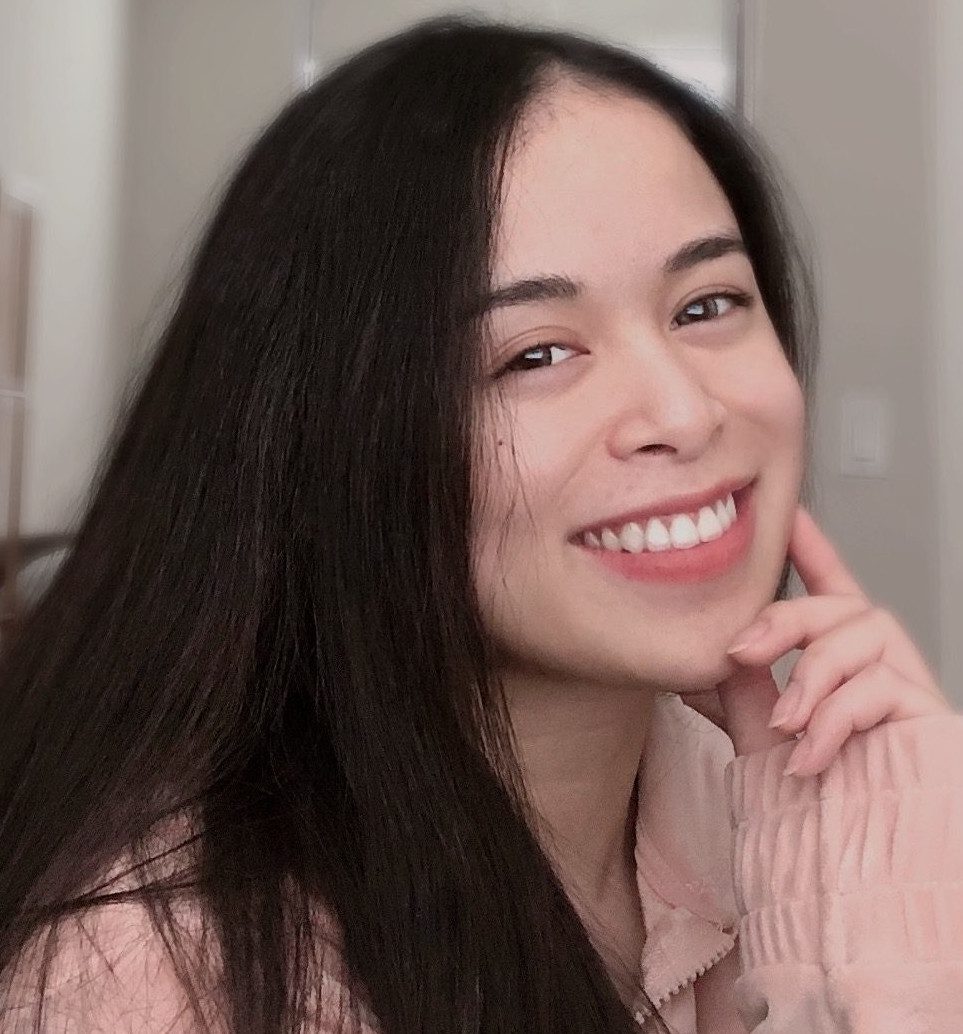
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Use IIFE
IIFE stands for Immediately Invoked Function Expression. This is simply a way to execute various functions immediately after they are created. They come in handy because they do not pollute the global object. Also, they make it easier to isolate variable declarations.
IIFE makes it easier to execute things quickly, and it can be used to plan your setup before the code is up and running. It also uses a simple API to initialize small libraries so developers can encapsulate complex logic in the code or expose objects that can be used to interact.
How to Learn JavaScript Best Practices
There are a lot of learning resources online to help you learn JavaScript best practices. They include online courses, video courses, or simple resources where you can find all the guidelines on your own. With the Internet, you have access to thousands of resources to work with. There is also an option of enrolling in one of the best JavaScript bootcamps.
Can a Bootcamp Help You Learn JavaScript Best Practices?
Yes, a bootcamp can help you to learn JavaScript best practices. Some bootcamps teach JavaScript alone, and some teach it as part of a software engineering program. The course you choose will give you intensive JavaScript training so you can apply for entry-level programming jobs. Bootcamps also give one-on-one support to students, despite remote learning.
These courses will teach coding practices to give students a clearer understanding of the bigger picture. Students use this knowledge in place of work experiences when they craft their portfolios. The programs are short, and within a few months, students can master all they need to enter the field.
Best Courses and Training Programs to Learn JavaScript Best Practices
Provider | Course | Price |
---|---|---|
App Academy | Software Engineering Immersive | $17,000 |
Digitalcrafts | Web Development Bootcamp | $14,950 |
General Assembly | JavaScript Course | $3,950 |
Ironhack | Web Development Bootcamp | $12,500 |
The Tech Academy | JavaScript Developer Bootcamp | $9,010 – $11,200 |
Should You Learn JavaScript Best Practices?
Yes, you should learn JavaScript best practices, especially if you want to start a career in tech. It is important to learn JavaScript’s best practices because they help save you time while coding. When you know what to avoid, it is easier to develop your application with minimal errors and cleaner code. You can learn best practices through online JavaScript courses.
Also, JavaScript is one of the most popular programming languages in the world today. As well, software engineers earn $53 per hour, according to the Bureau of Labor Statistics. Talented JavaScript developers can earn an even higher wage. If you want to have a successful career as a developer, you should learn JavaScript best practices.
JavaScript Best Practices and Guidelines FAQ
JavaScript is used to create interactive and dynamic experiences for users. The most notable applications and functions that make the worldwide web indispensable to us are coded in JavaScript. This is why it is considered the language of the web.
Some of the most prominent JavaScript best practices include adding semicolons, using IIFE, checking auto-type conversions, avoiding chaining loops, using promises instead of callbacks, organizing function declarations, and using the comment feature to describe things that are not clear or easily understood.
Yes, you can learn JavaScript best practices in coding bootcamps alongside several other JavaScript principles and concepts to prepare you for a career as a web developer. The programs are short but intensive, and they cover all you need within a few weeks.
Some of the important concepts you must learn before understanding JavaScript best practices include hoisting, promises, scope, closures, and callbacks. Each one is highly applicable to everyday development whether you are building an app or a website. Knowing these concepts can help you to avoid writing bad code or sloppy code.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.