How to Use JavaScript Booleans
True or false values are everywhere in programming. We call these values Booleans. They can be used to compare two or more values and control what parts of a program should run.
In this guide, we’re going to talk about what Booleans are and how they work. We’ll walk through a few examples of how to use JavaScript Booleans to help you get started.
What is a JavaScript Boolean?
A Boolean is a value that can either be True or False.
Booleans are named after George Boole, a famous mathematician who is associated with advancing the study of mathematical logic. This means that Booleans are always capitalized.
Every object with a value in JavaScript is true. Everything without a value is false.
The following values all evaluate to true:
- 10
- “Hello”
- True
Any null, false, undefined, 0, NaN or empty string value is considered to be false.
Let’s discuss how Booleans can be used in JavaScript!
Using Booleans to Make Comparisons
There can only be two outcomes of a comparison: true or false. This means that we can use Booleans to evaluate the outcome of a comparison.
JavaScript uses comparison operators to compare two values. These are:
- ==: Equal to
- !=: Not equal to
- >: Greater than
- <: Less than
- <=: Less than or equal to
- >=: Greater than or equal to
When you use the last two operators in the above list, you need to be sure that you arrange the signs in the correct order. JavaScript will not understand your code if you flip the signs around.
We’re going to build a program that evaluates the ages of two students in a high school class. Let’s start by checking whether a student, Alex, is older than another student, Lisa:
var lisa = 15; var alex = 16; console.log(alex > lisa);
Our code returns: true.
Alex is 16 and Lisa is 15. This means that Alex is older than Lisa. We can use any comparison operator to evaluate how two variables compare. Let’s see whether Alex is the same age as another student, Paul:
var paul = 16; var alex = 16; console.log(alex == paul);
This code checks whether the value of “alex” is equal to the value of “paul”. Both of these students are 16 years old. Our code returns: true.
Strings can be evaluated using JavaScript Booleans.
Let’s check if the student Lisa is on the honor roll this month:
var honor_roll = "Alex"; var student = "Lisa"; console.log(honor_roll == student);
Our code returns: false. We are checking to see whether the value of “honor_roll” is equal to the value of “student”. The honor roll student is Alex. This means that when we compare our two strings, false is returned. If Lisa was on the honor roll, our code would return true.
We can assign the outcome of this comparison to a boolean variable:
var is_honor_roll_student = (honor_roll == student); console.log(is_honor_roll_student);
Our code returns: true. This code performs the same comparison. The value of the Boolean object comparison is stored in the variable “is_honor_roll_student”. We then print the value of that variable to the console.
Using Booleans with Logical Operators
Logical operators are usually used to evaluate two or more expressions. They allow you to check whether two or more statements are true, if one of two statements are true, or if one or more statements are false.
There are three logical operators that can be used with Boolean functions:
- &&: And
- ||: Or
- !: Not
Let’s create a program which checks whether you can buy a new computer game from an online store. We’ll first check whether a customer has a high enough gift card balance in your account, or whether a customer has a credit card which can be charged for the computer:
var gift_card_balance = 25.00; var cost = 30.00; var card = true; console.log((balance >= cost) or (card == true));
This code checks whether the customer has enough money in a gift card or if the customer has a card associated with your account. Our code returns: true.
The customer does not have enough money on their gift card balance. They have a card attached to their account. Because one of the two expressions we have specified is true, the expression evaluates to true.
The game you are buying is rated 18+. Let’s check whether a customer is over 18 and has enough money to purchase the game:
var age = 19; var enough_money = true; console.log((enough_money == true) && (age >= 18))
Our code returns: true. We have used the && operator to check whether both of our conditions are met. They are met, so our code returns true.
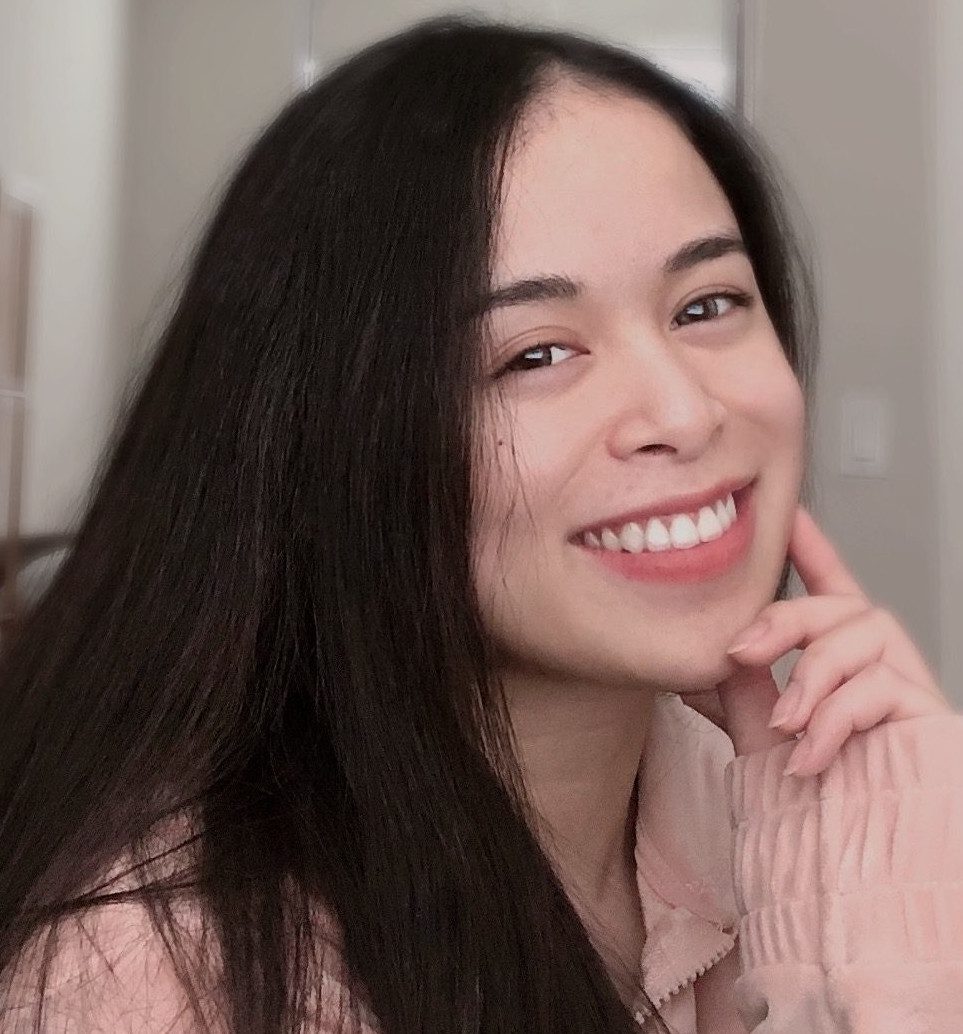
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
We can use the not operator to check if an expression is false. Let’s see whether our customer has purchased a game before:
var purchases = 1; var purchased_before = !(purchases == 0); console.log(purchased_before);
This code checks whether our customer has purchased a game before. We use the not (“!”) operator to invert the result of purchases == 0. This means that if a customer has purchased a game before, our expression will evaluate to true.
Using Booleans with an if Statement
Booleans are used to control the flow of a program. This means that you can use a Boolean to determine whether a certain block of code should be run.
This code snippet will print out a message to the console if a customer has a sufficient balance on a gift card to purchase a game:
var balance = 25.00; var cost = 30.00; if (balance >= cost) { console.log("This gift card has enough money."); } else { console.log("This gift card has an insufficient balance."); }
This code returns: This gift card has an insufficient balance.
Our code evaluates whether the customer’s balance is equal to or greater than the cost of the game. If the customer has enough money, the code inside our ”if” statement is run. Otherwise, the code inside our else
statement is run.
Let’s see what happens when we change the value of “balance” to $32.50:
var balance = 32.50; ...
Our code returns: This gift card has enough money.
Conclusion
A Boolean can store a true or false value. Booleans are commonly used to evaluate whether an expression is true or false. You can use a Boolean with an if
statement to run a block of code based on whether an expression evaluates to a certain Boolean value.
Now you’re ready to start using JavaScript booleans like an expert developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.