How to Concatenate NumPy Arrays
NumPy is an excellent library for working with arrays in Python. It covers everything from creating to manipulating arrays of all sizes. It’s no surprise then that NumPy comes with a utility that you can use to concatenate arrays.
The numpy.concatenate()
method joins two or more arrays into a single array.
In this guide, we’re going to talk about what NumPy arrays are and how you can concatenate them. We’ll walk through a few examples to help you get started. Let’s begin!
The Structure of an Array
A NumPy array is a type of array that works with the NumPy library. It looks like any other array but it is stored inside an ndarray object:
array([1, 2, 3])
To work with a NumPy array, we need to import the numpy library:
import numpy as np
We’re going to concatenate two arrays. One array will contain all the numbers between 1 and 9 (inclusive). The second array will contain all the numbers between 10 and 18 (inclusive).
Let’s create these arrays using the arange()
method:
first_array = np.arange(1, 10).reshape(3, 3) second_array = np.arange(10, 19).reshape(3, 3) print(first_array) print(second_array)
This code creates two arrays. Each array is a 2d array. We have made these arrays 2d by using the reshape()
method which creates a 3×3 grid for each array:
[[1 2 3] [4 5 6] [7 8 9]] [[10 11 12] [13 14 15] [16 17 18]]
We now have two arrays with which we can work! Let’s concatenate them using concatenate()
.
NumPy Concatenation
You can concatenate an array on two axes: by row or by column. This is accomplished using the concatenate()
method. The concatenate method can merge 1d, 2d, 3d, 4d, and arrays with a higher number of dimensions.
Concatenate by Row
We’ll start by concatenating our two arrays by row. This will align the items in our two arrays by row and merge them into one array:
final_array = np.concatenate((first_array, second_array)) print(final_array)
We have specified a tuple inside the concatenate()
function. This tuple contains the list of arrays that we want to concatenate. Without any parameters, concatenate()
concatenates by row.
Our code returns:
[[ 1 2 3] [ 4 5 6] [ 7 8 9] [10 11 12] [13 14 15] [16 17 18]]
Our arrays are concatenated by rows.
Concatenate by Column
You can use the concatenate method to merge two arrays by column.
To do this, we need to introduce a new parameter: axis. By default, this value is 0. This corresponds to the x axis, or the row axis. We can override this value and set it to 1. This will merge our two arrays by column.
Let’s concatenate our two arrays by column:
final_array = np.concatenate((first_array, second_array), axis=1) print(final_array)
Our code is almost the same as our last example. The difference is that we have specified the axis=1 parameter. Let’s see what happens when we run our code:
[[ 1 2 3 10 11 12] [ 4 5 6 13 14 15] [ 7 8 9 16 17 18]]
Our arrays are merged by column.
Concatenating More Than Two Arrays
The concatenate()
method can concatenate any number of arrays. Let’s try to concatenate three arrays on a horizontal axis. The arrays with which we will be working contain all numbers in the range of:
- 1 to 9 (inclusive).
- 10 to 18 (inclusive).
- 19 to 27 (inclusive).
Let’s define our arrays in Python:
first_array = np.arange(1, 10).reshape(3, 3) second_array = np.arange(10, 19).reshape(3, 3) third_array = np.arange(19, 28).reshape(3, 3)
Next, we can use concatenate to merge them together:
final_array = np.concatenate((first_array, second_array, third_array), axis=1) print(final_array)
We have specified our three arrays as a tuple in the concatenate()
method. When combined, these arrays correspond to all the numbers between 1 and 27.
Let’s run our program:
[[ 1 2 3 10 11 12 19 20 21] [ 4 5 6 13 14 15 22 23 24] [ 7 8 9 16 17 18 25 26 27]]
Our three arrays are now assigned to the variable “final_array”. We have printed this variable to the console to show that our arrays have been successfully merged.
Conclusion
The NumPy concatenate()
method joins two or more NumPy arrays. Arrays are joined on the vertical axis by default. You can join arrays on the horizontal access using the axis=1 flag.
You can concatenate two or more 1d arrays using the vstack and hstack methods. concatenate()
is more efficient than these methods. concatenate()
also supports concatenating 2d, 3d, and higher dimension arrays.
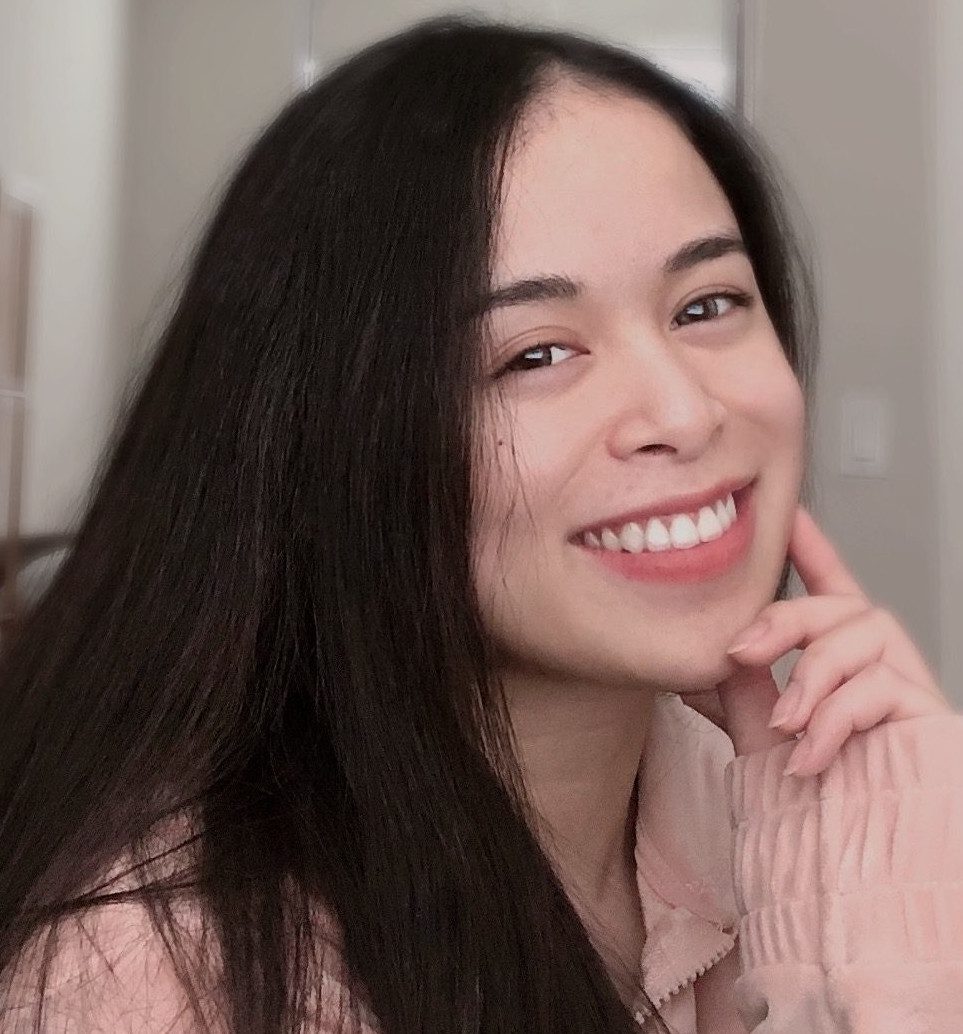
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Now you’re ready to start concatenating NumPy arrays like a Python expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.