The JavaScript array pop method called is one of several array methods that can be used to manipulate data in arrays. Web developers use it in times when they need to do something either with the shortened array or with the popped element. Let’s learn how to use it:
Syntax
The syntax for this method is fairly straightforward. By definition, the pop method removes the last element in an array and then returns it:
let frozen = [ "Anna", "Elsa", "Olaf", "Kristoff", "Sven" ]; let popped = frozen.pop();
If you were to type this code into the web browser console (*see side note below if you don’t know how to do that), and console.log
(frozen) and console.log
(popped), what would happen?
Frozen would come back as an array with one less element and popped would be the element that was removed from the array:
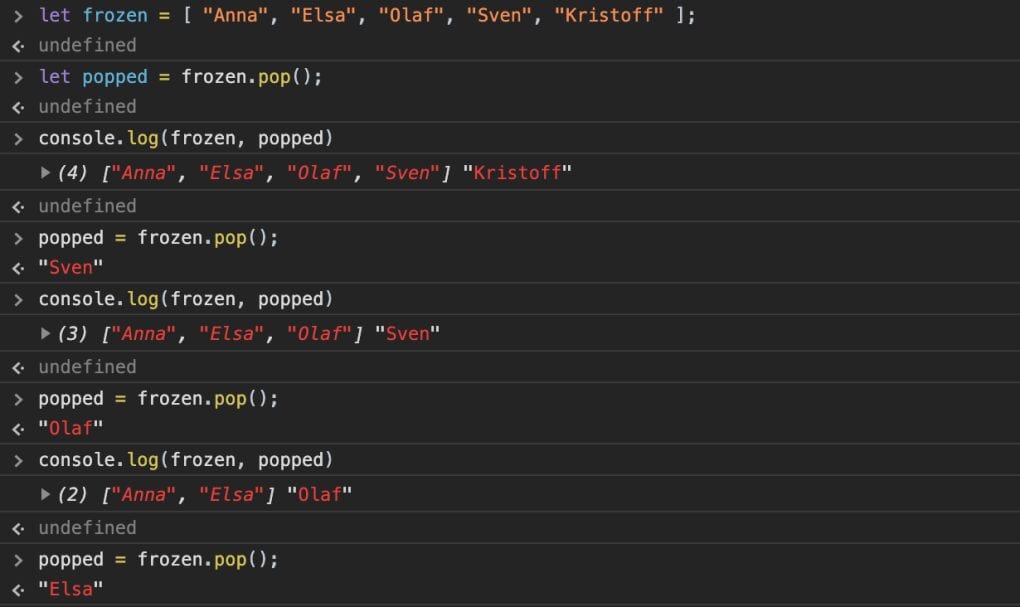
* Side Note: How to Access JavaScript Console in Chrome/Firefox/Safari:
Chrome/Firefox: In Chrome, right click on this page, select ‘Inspect’. You’ll see the Chrome Developer Tools pop open. At the top there should be a set of navigable tabs. Firefox is similar, but you will select ‘Inspect Element’. Click on Console and your workspace will be there.
Safari: If you use Safari, you will need to enable the ‘Developer’ menu. To do so, open the Safari browser, click on Safari and then go to ‘Preferences’. Click on ‘Advanced’. Check the ‘Show Develop menu in menu bar’ checkbox. Close the ‘Preferences’ and then click on ‘Develop’ in the menu bar. Select ‘Show JavaScript Console’.
You should now be able to type in the information in the example above (or come up with your own example!). Once both frozen and popped variables have been created, console.log both to see the effects the code has on the elements.
The pop method changes the length of the array. You can use it for as long as the length of the array exists. When there is an empty array, the method can still be performed (as in it won’t throw an error or anything), but the popped element will return ‘undefined’.
Conclusion
The pop array method opens up opportunities to use it in logic where we need to remove the last item from an array and then do something with it. This is the opposite of the push method, which adds something to the end of the array and is comparable to the shift method, which removes an element from the front of the array and returns that element.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.