Programmers like to reduce repetition in their code. The more you repeat your code, the less maintainable it is, and the slower your programs will run. That’s the reason why there are various methods in JavaScript to iterate through datasets.
One of these methods is called the map()
method. This method loops through an existing array and performs a specific function on all items in that array.
In this guide, we’re going to talk about how to use the JavaScript map()
function. We’ll walk through three common examples of the map()
function in action to help you get started.
What Is the map Function?
The JavaScript map()
method calls a specific function on each item in an array. The result of this function is then moved into its own array.
For instance, suppose you want to multiply every item in an array by two. You could do so by creating a function that multiplies every array item by two, and moving that function into a map()
method.
The syntax of the map()
function is:
const newArray = oldArray.map(function, thisValue);
function
is the callback function that will be run on each element in the array. To learn more about functions, you can read our ultimate guide to JavaScript functions. thisValue
is the default value that will be stored in the this
variable in the function. By default, this is undefined.
The map method creates a new array based on the results of the callback function.
The map()
method has a number of uses. The most common is to call a function on a list of array elements. An example of this would be multiplying every number in a list of numbers, or finding the length of each string in a list of strings.
You’ll also find the function used to render lists in JavaScript libraries such as React or Vue.js.
Calling a Function with Map
The map()
method allows you to perform a repetitive task on every item in a list, which makes it useful in a number of cases.
Let’s say that you own a cookie store and you are going to raise the prices of each cookie by 5%. Rather than calculating all of the new prices individually, you could use the map()
method.
Here’s the code you would use:
const cookiePrices = [1.50, 1.75, 1.60, 2.00, 2.05, 1.45]; const newCookiePrices = cookiePrices.map(cookie => { var price = cookie * 1.05; return price.toFixed(2); }); console.log(newCookiePrices);
Our code returns:
["1.58", "1.84", "1.68", "2.10", "2.15", "1.52"]
In this example, we’ve increased the price of every cookie by 5%. First, we declared a list of cookie prices in the variable “cookiePrices”. Then, we used the map()
method with a function that calculates a 5% price increase for each cookie.
“cookie * 1.05” calculated the percentage increase, then we returned that increase, rounded to the nearest two decimal places. Finally, we printed the new array to the console using the console.log()
method.
We could also move our function into its own function to make our code more readable:
function calculateIncrease(cookie) { var price = cookie * 1.05; return price.toFixed(2); } const cookiePrices = [1.50, 1.75, 1.60, 2.00, 2.05, 1.45]; const newCookiePrices = cookiePrices.map(calculateIncrease); console.log(newCookiePrices);
Our code returns the same values from earlier, but is more readable:
["1.58", "1.84", "1.68", "2.10", "2.15", "1.52"]
Change Items in an Array
The map()
method is often used to change the items in an array. Let’s suppose that our cookie shop is building a loyalty program for its customers. Every cookie you purchase earns you 10 points, and if you earn 100 points, you will earn a free cookie.
The following program would allow us to accomplish this task:
var customers = [ { name: "Hannah Geoffrey", cookiesPurchased: 2 }, { name: "Peter Clarkson", cookiesPurchased: 3 }, { name: "Steven Hanson", cookiesPurchased: 7 } ] function calculateLoyaltyPoints(customer) { var newCustomer = { name: customer.name, cookiesPurchased: customer.cookiesPurchased, points: customer.cookiesPurchased * 10 } return newCustomer; } var customersWithPoints = customers.map(calculateLoyaltyPoints); console.log(customersWithPoints);
Our code returns:
[{ cookiesPurchased: 2, name: "Hannah Geoffrey", points: 20 }, { cookiesPurchased: 3, name: "Peter Clarkson", points: 30 }, { cookiesPurchased: 7, name: "Steven Hanson", points: 70 }]
In this example, we’ve calculated the total number of points each customer should be awarded based on how many cookies they have purchased.
First, we declared an array of objects called “customers”. This list stores the names of our customers and how many cookies they have purchased using key:value pairs.
Then, we declared a function called calculateLoyaltyPoints()
. This function adds a new item to each customer item called “points” which is calculated by multiplying the number of cookies each customer has purchased by 10.
We use the map()
method to iterate through our list of customers and apply our calculateLoyaltyPoints()
function. Finally, we print out the revised list of customers. This list now reflects our “points” value we added to each customer’s entry.
Render a List Using a Library
The map()
method is commonly used in JavaScript libraries like React. The purpose of this method is to render the items in a list. Let’s walk through an example of map()
in React.
Suppose we want to show a list of the cookies our store sells on our website. We could do so using this code:
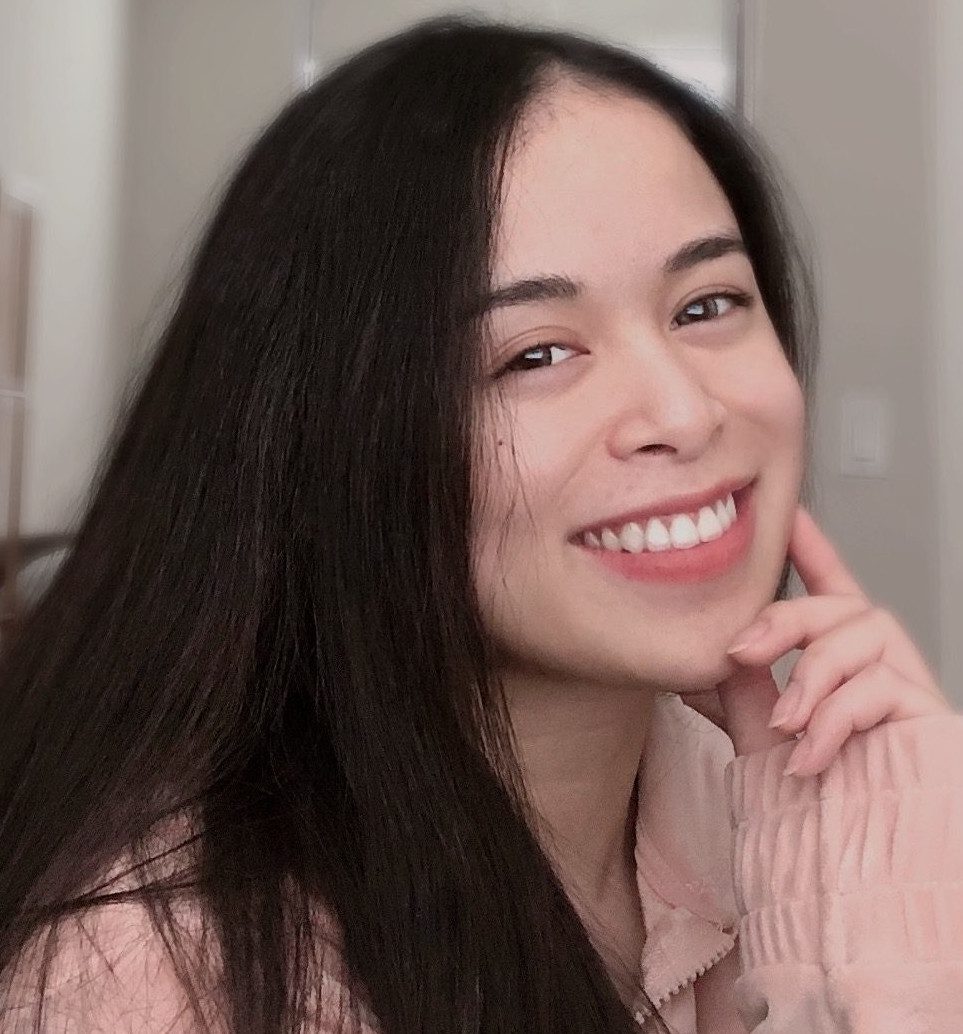
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
import React from "react"; import ReactDOM from "react-dom"; var cookies = ["Raspberry Chocolate Chip", "White Chocolate Chip", "Oat", "Milk Chocolate Chip"]; const CookieList = () => ( <div> <ul>{cookies.map((cookie, i) => <li key={i}>{cookie}</li> )} </ul> </div> ); const root = document.getElementById("root"); ReactDOM.render(<CookieList />, root);
This code creates a component in React which renders a list. Each list item is contained within a <li> tag which is rendered using map()
. map()
creates an individual <li> tag for each item in the list, and the “i” variable assigns each list item a unique key.
Map vs. Iterator Methods
Map is an example of an iterator method in JavaScript. These methods allow you to loop through all the items in a list and perform some action.
When you’re deciding to use the map()
function, it’s good practice to first ask whether another iterator method would be better. This will ensure that you choose the right tool for the job.
Here are the other iterator methods that exist in JavaScript:
- reduce(): The reduce method allows you to reduce an array to a single value. This method is best if you want to do something like adding up all the items in an array.
- filter(): Filter allows you to remove items from a list that do not meet particular criteria.
- forEach(): forEach() runs a function on every item in a list. forEach() is similar to a for loop because it allows you to loop over an array and perform a task on each item.
You may be thinking to yourself, “map sounds really similar to the forEach()
method. Are they the same?” That’s a good question. There is a subtle difference between these two methods.
The map()
function iterates over a list, changes each item in the list and returns a new list. The forEach()
function, on the other hand, iterates over a list and, as a side effect, applies some operation to the list.
The map()
function is best used if you need to call a function on every item in a list, declare a list component in a framework like React, or change the content of a list.
Conclusion
The map()
method is useful for performing repetitive tasks multiple times. It’s also useful for declaring components in React such as lists.
In this tutorial, we analyzed three main uses of the map()
method. Now you’re ready to start using the JavaScript map()
method like an expert developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.