Web applications can get very complicated, very quickly. One thing I’ve learned in my time as a developer is that there’s a lot you can do with plain old JavaScript if you give it the chance.
The localStorage API is a powerful built-in function that removes the need to use databases for simple applications that don’t need a server. We can use this API to save pieces of data locally which can be accessed by our application.
In this guide, we’re going to talk about how to use the localStorage method in JavaScript. We’ll run through an example of how to use localStorage to save the contents of a form for later on a web page. Let’s get started!
What Is localStorage?
localStorage is part of the web storage API. This API allows you to store data inside a user’s browser which your application can access.
The storage API is useful because it means that you don’t need to send every piece of data about a user’s session to a database. If a user enables dark mode on your site, you can track that on the client side; if a user saves a form for later, you don’t need to store the half-completed form in your database.
There are two types of web storage. localStorage is data that will stay in a user’s browser window even after they close the browser tab. Unlike cookies, localStorage data is data with no expiration date. sessionStorage is data that lasts until a session expires, which means until the browser is closed.
In this post, we are going to focus on the localStorage object. However, you can substitute this for sessionStorage because they both use the same syntax.
How to Use localStorage
Before we delve into our example, let’s walk through the methods offered by localStorage.
To create a localStorage entry, we can use a method called setItem()
:
localStorage.setItem("name", "Linda Carlton"); console.log(localStorage);
Our code returns:
{ name: "Linda Carlton", length: 1 }
localStorage stores items in key:value pairs. In our example, we have created an item called “name”. This item has the value “Linda Carlton”.
We used a console.log()
statement to print out the contents of localStorage to make sure that our value has been added.
You can use this same code to update a value stored in localStorage.
To retrieve a particular value from localStorage, you can use the getItem()
method:
localStorage.getItem("name");
Our code returns:
Linda Carlton.
There are two methods you can use to remove data from localStorage:
- removeItem(): Removes a single item from localStorage
- clear(): Removes every item in localStorage()
If we want to remove our “name” item from local storage, we could use this code:
localStorage.removeItem(name); console.log(localStorage);
Our code returns:
{ length: 0 }
Notice that even though we cleared our localStorage there is still one item stored: length. This tells us how many items are stored in localStorage. Now that we’ve removed the “name” entry, there is nothing stored in localStorage.
It’s also important to note that localStorage can only store strings.
Building a Form Saving Feature Using localStorage
We’ve all been on some websites that ask us to fill out a long form. Unless something is really important, I like to be able to go back to that form later. That’s why so many sites have “save form” features that keep track of your form details for later.
While some of these probably track your data in a database, you can make a lightweight version using the localStorage method.
Create the Front End
We’re going to create a customer feedback form for a local tea house, The Little Tea House. Our first step is to create the front-end for our web application. Create a file called index.html and paste in the following code:
<!DOCTYPE html> <html> <head> <title>The Little Tea House Feedback</title> <link rel="stylesheet" href="./styles.css" /> </head> <body> <div> <h1>The Little Tea House: Customer Feedback</h1> <p>How was your experience at The Little Tea House? We'd love to learn more!</p> <form> <label for="name">Name: </label> <input id="name" type="text" /><br /><br /> <label for="email">Email: </label> <input id="email" type="email" /><br /><br /> <label for="feedback">Message: </label><br /><br /> <textarea id="feedback"></textarea><br /><br /> <button id="saveButton">Save form</button> <button id="retrieveButton">Retrieve form</button> </form> </div> <script src="./form.js"></script> </body> </html>
This code creates a basic HTML page with our form fields:
Our site now has everything it needs, but we’re going to add in a few styles to make it more aesthetically pleasing. Create a file called styles.css and paste in this code:
div { margin-left: 15vw; margin-right: 15vw; background-color: lightblue; padding: 20px; }
Now go back to the website and refresh the page:
As you can see, our form now appears in the center of our web page and has a blue background. It looks great! Now we’re ready to start writing the JavaScript for this webpage.
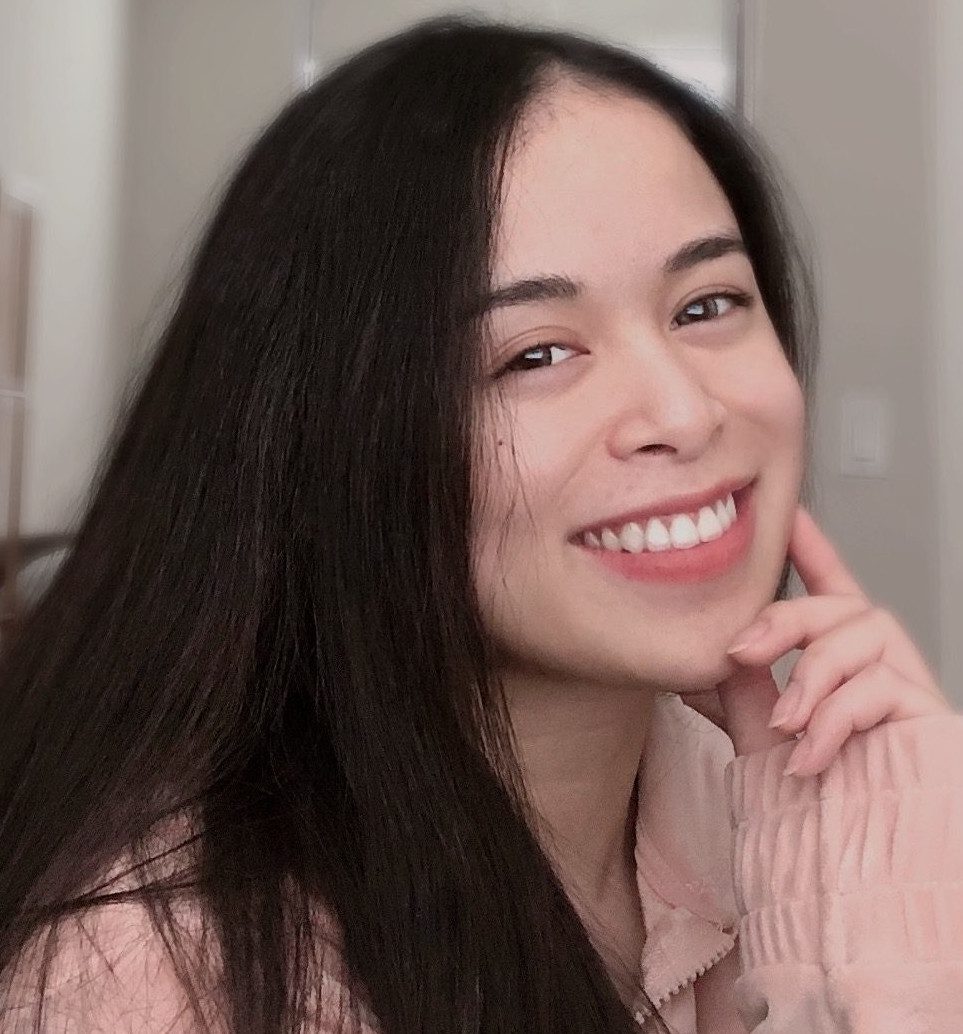
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Create a Form Saving Feature
Our next step is to collect the values stored in our form fields. From above, our form fields have the IDs:
- name (our Name field)
- email (our Email field)
- feedback (our Message field)
We can use these IDs to retrieve the values from each of our form fields. Create a file called form.js and paste in this code:
var nameField = document.getElementById("name"); var emailField = document.getElementById("email"); var feedbackField = document.getElementById("feedback");
We’re declaring these as “var” variables because we are going to change their values later on. We’re also going to retrieve our buttons so that we can make them clickable later in the tutorial:
var saveButton = document.getElementById("saveButton"); var retrieveButton = document.getElementById("retrieveButton");
Our next step is to create a function which saves these values into localStorage when our button is pressed. We’ll do this using the following code:
function saveResponses() { localStorage.setItem("name", nameField.value); localStorage.setItem("email", emailField.value); localStorage.setItem("feedback", feedbackField.value); }
This code will not run until our saveResponses()
function is invoked. So, how do we make this happen? We’ve got to link it up to our button. This code allows us to invoke our function when our button is clicked:
saveButton.addEventListener("click", saveResponses);
Now when we press our “Save form” button, our responses will be stored in localStorage.
But it’s no use storing this data if we can’t retrieve it. We’re going to write a function that retrieves this data when the “Retrieve form” button is clicked:
function retrieveResponses() { nameField.value = localStorage.getItem("name"); emailField.value = localStorage.getItem("email"); feedbackField.value = localStorage.getItem("feedback"); } retrieveButton.addEventListener("click", function(e) { e.preventDefault(); retrieveResponses(); });
Great! This code sets the value of each of our form fields to that which is stored in localStorage if a value is available. Now, let’s run our code and see what happens:
Our form looks the same. Try to insert some values into the form and press the button “Save form”. This will save your responses to the form.
Now, refresh your page and press “Retrieve form”. You’ll notice that your form responses were saved! The information in localStorage will persist even if we close the tab. It will not be deleted when the browser is closed either. We did it!
These form responses will be saved until you either save your form again or clear your cache.
Note: You should not use this technique to store sensitive information. All data in localStorage is stored in plain text, which means it is accessible to any user who reads it.
Conclusion
The localStorage method allows you to store data locally in JavaScript. For simple applications like a to-do list, saving user preferences, or saving a user’s form data (not including sensitive data), it’s much better than using a database. localStorage is easy to set up, easy to use and is 100% vanilla JavaScript.
Now you’re ready to start using the localStorage method like an expert JavaScript developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.