The JavaScript Object.keys() method retrieves the keys in an Object and returns a list that contains those keys. The order of the keys in the final list is the order they appear in the original Object.
JavaScript (JS) Objects consist of two main parts: keys and values.
When working with an Object, you may want to retrieve a list of the keys associated with it. That’s where the JavaScript Object.keys() method comes in. This method allows you to generate a list of all the property names in an Object.
Using examples, this tutorial will discuss how to use the Object.keys() method to return a list of keys stored in an Object. We’ll also quickly discuss the structure of JavaScript Objects.
JavaScript Objects: A Refresher
Objects (with a capital “O”) are the mapping data type in JavaScript. They map keys to values. The values stored by an Object can include strings, numbers, Booleans, and other data types. Here is an example of an Object in JavaScript:
const job_description = { position: "Sales Assistant", floor_worker: true, hours_per_week: 38 };
The words to the left of the colons (:) are the keys in our dictionary. Words to the right of the colons are the values. You can think of JavaScript Objects as the equivalent of a Python dictionary, but in JavaScript.
JavaScript Object.keys()
The JavaScript Object.keys() method returns the keys inside a JavaScript Object, or a JSON Object. These keys are stored in the order they appear in the Object.
The syntax for the Object.keys() method is:
Object.keys(object_name)
The Object.keys() method accepts one parameter: the name of the Object whose keys you want to retrieve. This method returns the names of all keys in the Object you have specified, stored as a JavaScript list.
Notice that the method itself is called Object.keys(). This is because keys() is a method of Object. You must specify the Object whose keys you want to retrieve as a parameter of Object.keys(). You cannot append keys() to any Object because that Object will not have access to the keys() method.
Object.keys() JavaScript Example
Let’s walk you through an example to illustrate how this method works.
Earlier, we created a dictionary called “job_description” which outlines some information about a job available at a local superstore. Our Object contains a JavaScript boolean, a string, and an integer. Now, suppose we want to retrieve a list of the keys in that dictionary.
We could create this list using the following code:
const job_description = { position: "Sales Assistant", floor_worker: true, hours_per_week: 38 }; var job_keys = Object.keys(job_description); console.log(job_keys);
Our code returns:
["position", "floor_worker", "hours_per_week"]
First, we declared a constant called “job_description”, which stores an Object with information about an available job at a local superstore.
Then, we used Object.keys() to retrieve a list of the keys associated with the dictionary, and we assigned the list to the variable “job_keys”. Next, we printed out the contents of the “job_keys” list to the JavaScript console.
As you can see, our code returned a list that contains three values. Each value represents a unique key name in our Object.
JS Object.keys(): Another Example
Let’s discuss another example of how to use this method.
Suppose we want to print out a list of the keys in our Object, preceded by “Key Name: “. We could do so using this code:
const job_description = { position: "Sales Assistant", floor_worker: true, hours_per_week: 38 }; var job_keys = Object.keys(job_description); for (var key of job_keys) { console.log("Key Name: " + key); }
Our code returns:
Key Name: position Key Name: floor_worker Key Name: hours_per_week
We first defined our “job_description” Object whose keys we want to print to the console. Then, we used the JS Object.keys() method to retrieve a list of the keys in our Object.
Next, we used a “for…of” loop to loop through every key in our “job_description” Object.
For each key, we printed “Key Name: “, followed by the name of the key, to the console. If you’re interested in learning more about how “for…of” loops work, read our beginner’s guide to JavaScript for loops.
Conclusion
The Object.keys() method retrieves a list of keys stored in a JavaScript Object. The resultant keys are stored in a list. You cannot append keys() to the end of an Object to retrieve its keys. You must use the Object.keys() syntax.
This tutorial discussed the basics of JavaScript Objects and how to use the Object.keys() method. Now you’re ready to use Object.keys() to retrieve a list of keys in an Object like a professional JavaScript developer!
To learn more about coding in JavaScript, read our How to Learn JavaScript guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
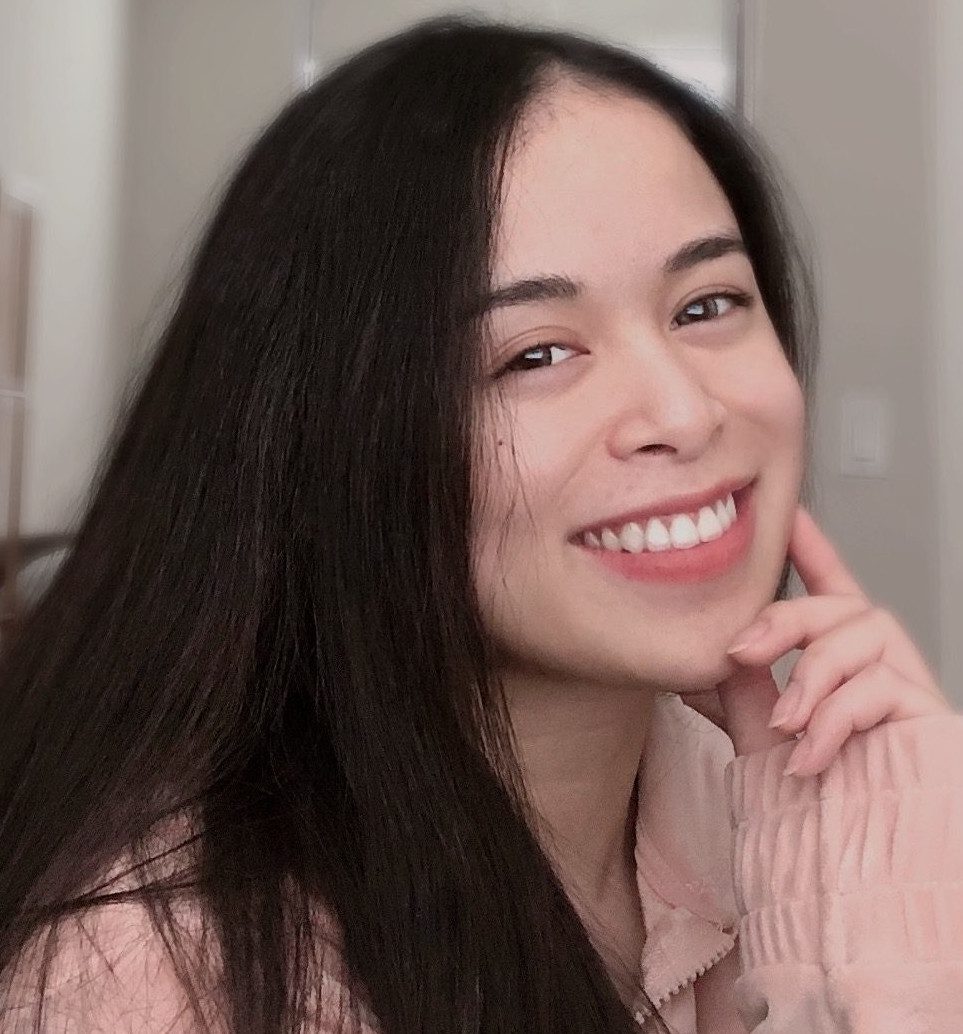
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot