Data types are used to store a particular type of data in a programming language. For example, strings can be used to store text-based data in code, whereas numbers can be used to store integers and floating-point numbers.
When you’re working with data types in JavaScript, you may want to find out which type of data a particular value holds. That’s where the typeof method comes in. The JavaScript typeof operator can be used to determine the type of a particular item in your code. This can be useful if you want to check whether data holds the type your program expects, which is a common operation in programming.
In this tutorial, we are going to discuss data types in JavaScript and how you can use the typeof operator to find out the type of data a particular value holds. We will also explore a number of examples of the typeof operator being used in action.
JavaScript Data Type Refresher
JavaScript features a number of data types which can be used to classify a certain type of data. Each type of data is treated differently by JavaScript, and each data type has its own methods that can only be used with data structured in the appropriate format.
JavaScript uses dynamic data types, which means that the type of data is checked during runtime instead of when the program is being compiled. This means that a variable of the same name can be used to hold different types of data.
In JavaScript, there are six basic data types that can be used to hold particular types of data, which are: string
, number
, boolean
, object
, array
, and function
. Later in this article, we have a reference guide which explains how these values work.
Each of these six data types works in different ways. So, when you’re programming, you may want to determine how a particular value is stored so you know how you should be working with the data. For example, how you work with a string will be different to how you would work with a number.
JavaScript typeof
typeof
is a built-in JavaScript operator that returns a string indicating the type of data a particular value holds. The operator can be used to find the type of data held by any value, or it can be used to find the type of a variable.
Here is the syntax for the typeof
operator:
typeof data_object
So, let’s say that you have a variable called phone_name
and you want to check its data type. You could do so using the following code:
var phone_name = "iPhone 11"; console.log(typeof phone_name);
Our code returns: string
. On the first line, we declare a variable called phone_name
which holds the string value iPhone 11
. Then, on the next line, we use typeof
to check the type of data held in our variable. In this case, the program returns string
.
We can check any type of data in JavaScript. Here’s an example of typeof
being used to check the value of a variable that has been assigned a Boolean:
var phone_in_stock = true; console.log(typeof phone_in_stock);
The typeof
operator returns: boolean
.
The typeof
function is often used to compare the data type held by a variable. So, if you wanted to check whether or not phone_name
was a string, and run a block of code if phone_name
was a string, you could do so using typeof
. Here’s an example of an if statement that will execute a block of code if phone_name
is a string:
var phone_name = "iPhone 11"; if (typeof phone_name === "string") { console.log("The variable 'phone_name' is a string."); };
Our code returns:
The variable 'phone_name' is a string.
There’s a lot going on in our code, so let’s break it down. On the first line, we declare a variable called phone_name
and assign it the value iPhone 11
. Then, we create an if
statement that checks whether or not the typeof
phone_name
is equal to string
.
In this case, the variable phone_name
is a string, so the code within our if
statement is executed. Hence, the output of our program was The variable phone_name is a string
.
JavaScript Typeof Arrays and Objects
When you’re working with JavaScript arrays and object literals, the typeof
function will not return the specific type of object with which you are working. Instead, it will return the label object
. Here’s an example of typeof
being used on both an array and a dictionary:
var sandwich_fillings = ['Ham', 'Cheese', 'Egg Mayo']; var ham_sandwich = { name: 'Ham', price: 2.50 }; console.log(typeof sandwich_fillings); console.log(typeof ham_sandwich);
Our code returns:
object object
As you can see, our program has returned object
to describe both the types of data with which we are working, even though our sandwich_fillings
variable has been assigned an array, and ham_sandwich
has been assigned a dictionary. This is because object
is used as a generic value to describe more complex data types in JavaScript.
JavaScript Typeof Undefined and Null
The typeof
operator also returns different values for null and undefined. When you’re working with a null
value, typeof
will return an object
, and undefined
will return its own undefined
value. Here’s an example of these data types in action:
console.log(typeof null); console.log(typeof undefined);
Our code returns:
object undefined
As you can see, null
is considered an object
, and undefined
has its own value called undefined
. So, if you’re working with these types of data, remember that they will return these values.
JavaScript Data Types
As we discussed earlier, JavaScript offers six main data types which classify particular types of data. These six data types can be divided into three categories: primitive
, composite
, and special
.
Strings, numbers, and booleans are all primitive data types, which means they are primary data types. Objects, arrays, and functions are all considered composite data types, and are all types of objects. Undefined and null are considered special data types.
Let’s break down the main types of data in JavaScript to illustrate what you can expect when you’re working with the typeof
operator.
Numbers typeof
JavaScript has one number type which stores both integers and floating-point numbers. As a result, numbers are able to store both decimal and non-decimal numbers. Here’s an example of a number in JavaScript:
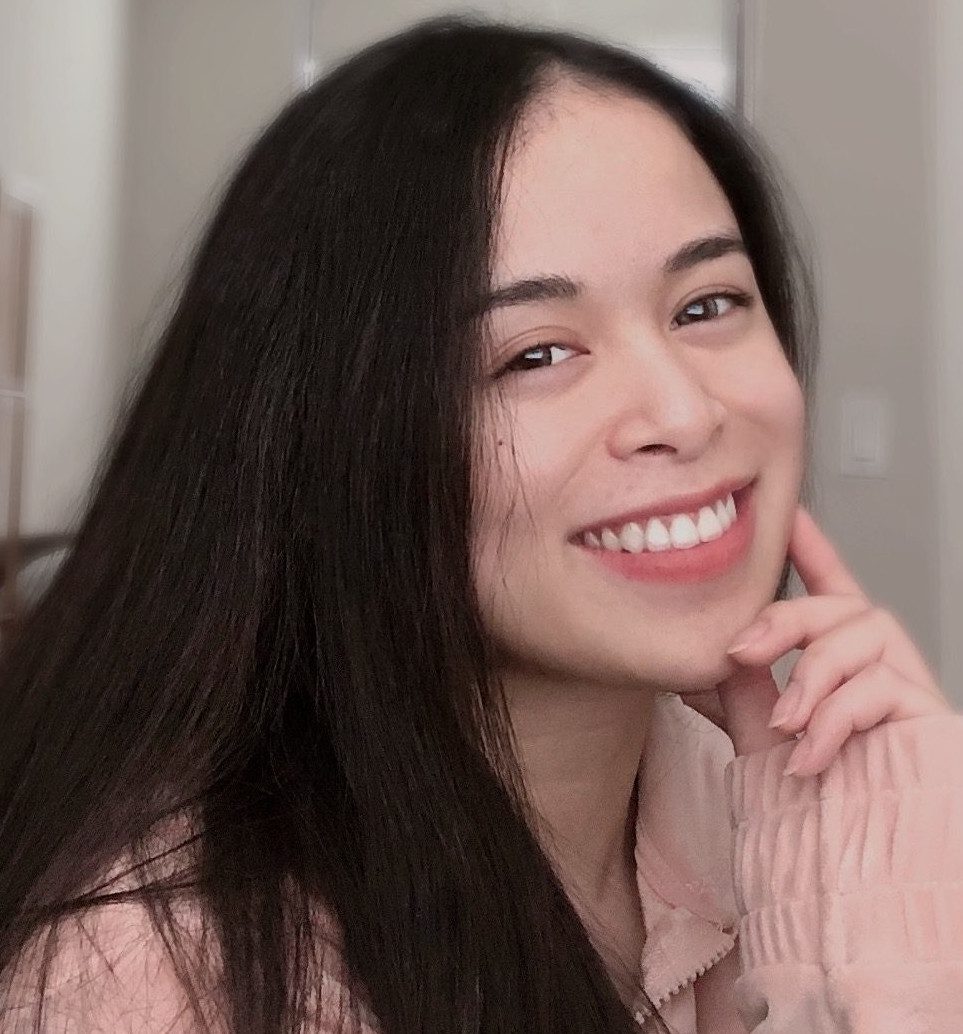
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
var espresso_price = 1.80;
When you use typeof
on a number, the following will be returned: number
.
Strings typeof
Strings are sequences of one or more characters and can include letters, numbers, symbols, and whitespaces. Strings represent text-based data, and can be declared using either single quotes (‘’
), double quotes (“”
), or backticks (``
).
Here’s an example of a string in JavaScript:
var espresso_name = "Espresso"; "typeof" returns "string" for any string data type.
Booleans typeof
Booleans can store one of two values: either true or false. Booleans are used to represent whether or not a condition has been met, and are often used in mathematical logic. Here’s an example of a boolean in JavaScript:
var espresso_in_stock = true; "typeof" returns "boolean" for the Boolean data type.
Arrays typeof
Arrays can store multiple values within a single variable, and are defined as a list of comma-separated values enclosed within square brackets ([]
). Here’s an example of an array in JavaScript:
var coffee_menu = ['Latte', 'Cappuccino', 'Espresso', 'Americano', 'Cortado']; "typeof" returns "object" for array data types.
Objects typeof
Objects can contain multiple values stores as key/value pairs. These can be used to store and access data in JavaScript. Objects are declared as a list of key/value pairs separated by commas and enclosed within curly braces ({}
).
Here’s an example of an object in JavaScript:
var latte_information = { name: 'Latte', price: 2.50, milk: true }; "typeof" returns "object" for JavaScript objects.
Undefined and Null typeof
The undefined
value is the default value for a variable with no value assigned. Here’s an example of a variable with the type undefined
:
var coffee;
This variable has no value, so it is considered undefined
by typeof
.
null
is an object that is used to describe a variable that has no value. Here’s an example of a variable with a null
value:
var coffee = null;
Conclusion
The JavaScript typeof
operator can be used to determine the type of data a particular value holds. typeof
returns the type of an expression or the type of a variable. The typeof
operator is useful if you want to figure out how data is stored so you know whether you need to convert data to another type in your program for it to work correctly.
In this tutorial, we explored how to use the typeof
operator in JavaScript. We also discussed how typeof
responds to arrays, objects, null, and undefined values. In addition, we briefly covered the main types of data in JavaScript and discussed how typeof
reacts to those data types.
Now you’re ready to start using the JavaScript typeof
operator like a professional!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.