do…while loops can be used to execute a block of code once and will keep running if a statement continues to evaluate to true. while and do…while loops are useful if you want to execute a block of code while a certain condition is met.
Loops are a core feature of programming and are used to automate similar tasks. For example, say you want to print out every name in a list of students. You could create a loop to automate the task.
JavaScript offers two main types of loops which can be used to perform a repetitive task multiple times. The first type, known as a for loop, executes a statement a specific number of times. The second type is the while loop, which runs based on whether a condition evaluates to true.
In this tutorial, we are going to discuss the basics of the while loop in JavaScript. We will explore the syntax for while loops, how to create do…while loops, and explore a few examples of these loops in action.
JavaScript While Loops
A JavaScript while loop executes a block of code while a condition evaluates to true. while loops stop executing when their condition evaluates to false. A while loop lets you repeat a block of code multiple times without copy-pasting your code.
while loops are often used if you want to run code for an unspecified number of times. On the other hand, JavaScript for loops are used if you already know or can calculate how many times your loop should execute.
Here’s the syntax for a while loop in JavaScript:
while (condition_is_true) { // Run code }
The while statement looks very similar to a JavaScript for statement.
You can create an infinite loop by specifying a condition that will always evaluate to true. Make sure that your condition can evaluate to false unless you want your loop to continue until you manually stop your program.
JavaScript While Loop Example
Let’s say that we are hosting an exclusive party. We only want to invite 40 people to our party at any given time.
There are already 35 people at our party. Every time we invite someone we want to calculate how many more people we can invite before we reach the limit. Here’s an example of a program that uses a while loop to perform this calculation:
var limit = 40; var current_attendees = 35; while (current_attendees < limit) { var current_attendees = current_attendees + 1; var current_attendees = current_attendees + 1; var spaces_left = limit - current_attendees; console.log(`There are ${spaces_left} spaces left in the party.`); };
Our code returns the following:
There are 4 spaces left in the party. There are 3 spaces left in the party. There are 2 spaces left in the party. There are 1 spaces left in the party. There are 0 spaces left in the party.
On the first two lines of our code, we declare two variables. The limit variable is used to specify the maximum number of people who can attend our party. The current_attendees JavaScript variable keeps track of how many people are currently attending the party.
We create a while loop that executes as long as the number of current attendees is less than the limit we have set.
Then, our program adds 1 to the number of current attendees, and calculates the number of spaces left by subtracting current_attendees from limit. Finally, our program prints out a statement to the console which tells our party leader how many spaces are left.
Our while loop ran as long as there were under 40 people in our party. Each time the loop was executed our current_attendees increased by 1. As soon as our party reached 40 attendees, our loop stopped running.
while Loops: Another Example
Let’s use another example to illustrate the while loop in action. In the below example, we create a while loop which prints out every name in our array of party VIPs:
var vips = ['Robert De Niro', 'Will Smith', 'Brad Pitt', 'Denzel Washington']; var counter = 0; while (counter < vips.length) { console.log(vips[counter]); var counter = counter + 1; };
Our code returns:
Robert De Niro Will Smith Brad Pitt Denzel Washington
Our loop iterates through the vips array as long as our counter variable is less than the length of the JavaScript array.
JavaScript do…while Loops
A JavaScript do…while loop executes a statement once and then it checks if a condition is true. If the condition is true, the loop will be executed again. Otherwise, the code stops running.
There is one big difference between a while and a do…while loop. do…while loops execute at least once even if the condition they specify never evaluates to true. while loops, on the other hand, only execute if their condition evaluates to true.
Here’s the syntax for a do…while loop in JavaScript:
do { // Run code } while (condition_is_true);
The code in our do…while loop will always be executed at least once.
JavaScript do…while Loop Example
Let’s use an example to illustrate how the do…while loop works. Let’s say that we are creating a guessing game. We want our program to keep asking a user to guess a number until they guess the correct number.
Here’s an example program we could use to create this guessing game:
var number_to_guess = 7; var guessed_number = 0; do { var input = prompt("Enter a number between 1 and 10.") var input = prompt("Enter a number between 1 and 10.") var guessed_number = parseInt(input); } while (guessed_number != number_to_guess);
Our program will ask the user to insert a number between 1 and 10 as long as guessed_number is not equal to number_to_guess. But our program will execute the contents of our do statement before it evaluates the conditions for the first time.
When our condition is false, our loop will stop executing.
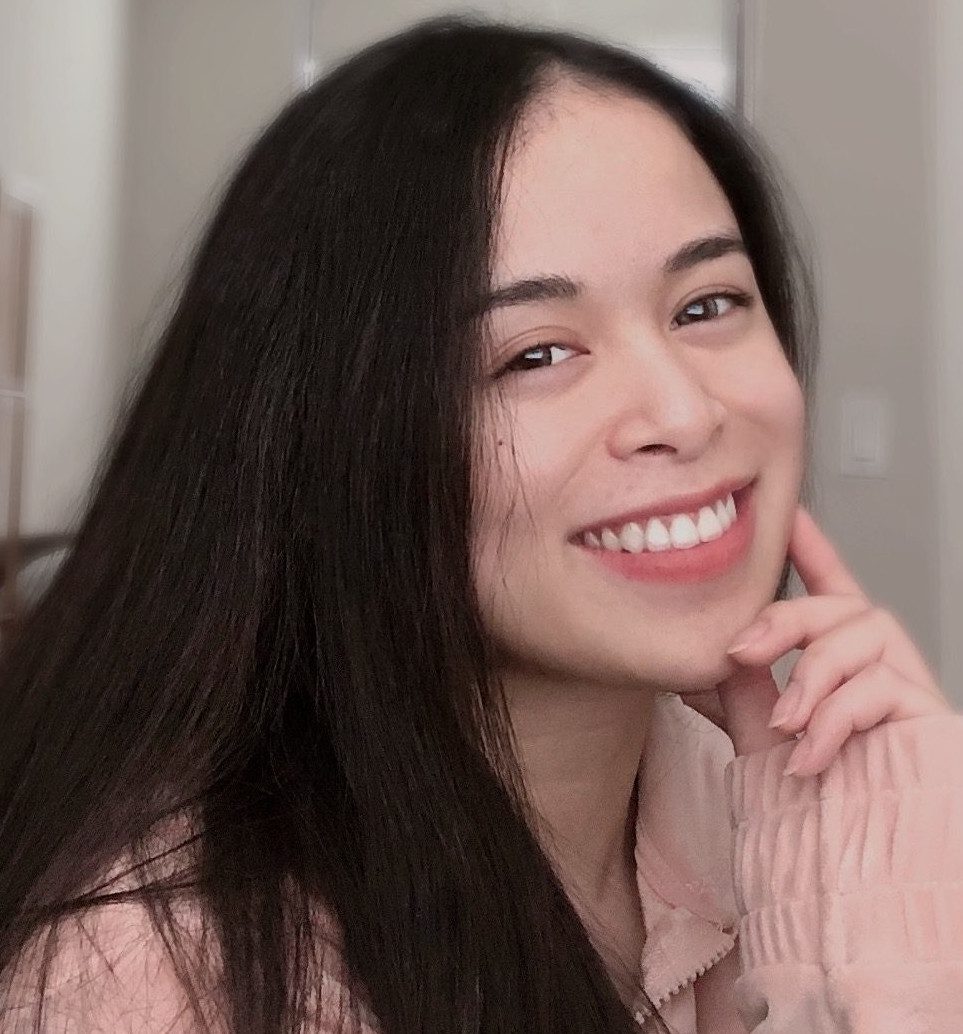
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conclusion
While loops can be used to execute a repetitive block of code while a statement evaluates to true. do…while loops can be used to execute a block of code once. They will keep running if a statement continues to evaluate to true.
While and do…while loops are useful if you want to execute a block of code while a certain condition is met.
In this tutorial, we discussed how to create a while and do…while loop in JavaScript. We also explored a few examples of these loops in action to illustrate where they may be useful. Now you’re equipped with the information you need to use while loops like a JavaScript expert!
For advice on top JavaScript learning resources and courses, check out our How to Learn JavaScript article.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.