When you’re working with data in JavaScript, you may want to convert a data type to a string. For example, you may have an array of student names that you want to appear as a string, or a number that you want to convert to a string.
That’s where the JavaScript toString() function comes in. toString() can be used to convert a number or an array to a string value. Converting another data type to a string can be useful when you want to use string methods on the data with which you are working.
In this tutorial, we are going to discuss how to use the JavaScript toString() method to convert a number or an array to a string. We will also go through a few examples to illustrate this method in action.
JavaScript String Refresher
Strings are sequences of one or more characters and can include numbers, letters, symbols, and whitespace characters. In JavaScript, strings are immutable, which means that they are unchanging after declaration. Strings are an important data type because they allow you to interact with text in your programs.
JavaScript offers three ways to declare a string: using single quotes (‘’), double quotes (“”), and backticks (“). Both single and double quotes function in the same way, although backticks have a few differences that make them useful in specific cases. In your programs, there is no need to use the same type of string throughout.
Here’s an example of a string in JavaScript:
var example_string = “This is an example string.”
In our code, we have assigned the string “This is an example string.”
to the variable “example_string.” Now that we have a variable that contains our string value, we can reference our string multiple times throughout our program. So, if we want to print out our string to the console, for example, we could use this code:
console.log(example_string);
Our code returns: “This is an example string.”
Strings come with a variety of template string methods that can be used to manipulate their contents. For example, contains() can be used to determine whether or not a string contains another value, and split() and slice() can be used to retrieve certain parts of a string. But if we want to make use of these methods, our data must first be represented as a string.
JavaScript toString
The JavaScript toString() string representation method allows you to convert a number or an array to a string. This is useful if you want to perform a string method on a number or a string because first, you will need your data to be structured as a string.
Here is the syntax for the toString() method:
data.toString()
The toString() method takes in no parameters and returns the data you specified as a string.
JavaScript Number toString
So, let’s say you have a number that you want to convert into a string. You could do so using the following code:
var seven = 7; console.log(seven.toString());
Our code returns the following: “7”
. While the contents of our data are still the same—it is still the number seven—our data is now stored as a string. We can check this using the “typeof”
keyword which prints out the type of a value. Here’s an example of using “typeof”
to check the type of our data:
var seven = 7; console.log(typeof seven); var seven_string = seven.toString(); console.log(typeof seven_string);
Our code returns the following:
number
string
As you can see, the result of our first “typeof” statement was “number,” because we had assigned the variable “seven” the value “7.” Then, after we converted seven to a string using toString(), we used “typeof” again to check the value of our new data. “typeof seven_string” returned “string” because we had converted our data to a string.
JavaScript Array toString()
The toString() method can also be used on a JavaScript array to convert the contents of the array to a string. The toString() method joins all elements in an array into one string and returns a new string with each array element separated by a comma.
Here’s the syntax for the Array.toString() method:
array.toString();
Let’s use an example to illustrate how this method works. Let’s say that you have an array of fruits that you want to convert into a string. Here’s the code you could use to convert the data:
var fruits = ['Apple', 'Pear', 'Mango', 'Raspberry', 'Blueberry', 'Banana']; console.log(fruits.toString());
Our code returns the following:
Apple,Pear,Mango,Raspberry,Blueberry,Banana
The contents of our data are the same—we have all of our fruits—but now they are stored in a string instead of an array. If we use the “typeof” method, we can see that our data has been converted to a string:
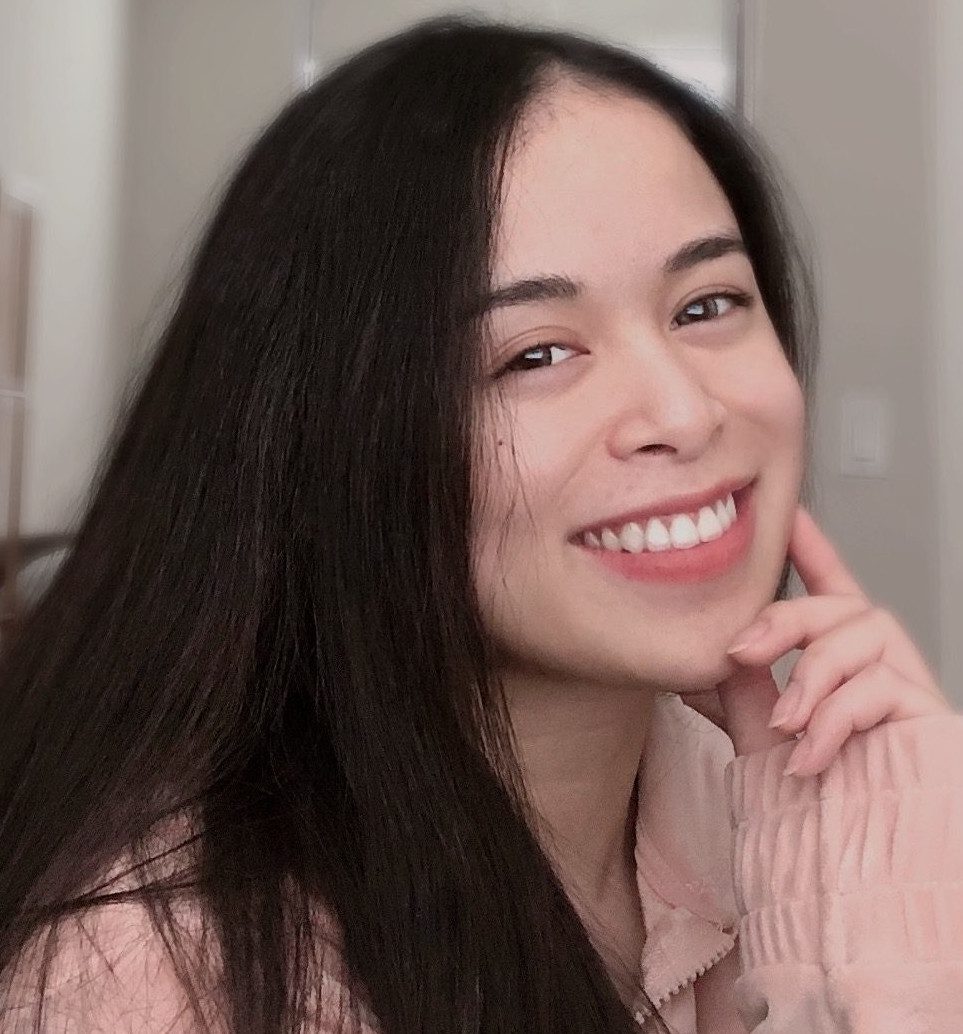
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
var fruits = ['Apple', 'Pear', 'Mango', 'Raspberry', 'Blueberry', 'Banana']; console.log(typeof fruits); var string_fruits = fruits.toString(); console.log(typeof string_fruits);
The output of our code is as follows:
object
string
The result of “typeof fruits”
was “object”
because our fruits were stored as an array. However, after we converted our array to a string using “fruits.toString(),” the “typeof string_fruits” function returned “string.”
Thus, as you can see, our data has been converted into a string.
In addition, the toString() method can be used to convert an array of arrays into a string. So, if we had an array of fruits with two items, an array of berries and an array of other fruits, we could still use the toString() function to convert our data into a string.
Here’s an example of toString() being used to convert an array of two arrays into one string:
var fruits = [['Apple', 'Pear', 'Plum', 'Mango'], ['Strawberry', 'Raspberry', 'Blueberry', 'Gooseberry']]; console.log(fruits.toString());
Our program converts our “fruits” array to a string and returns the following:
Apple,Pear,Plum,Mango,Strawberry,Raspberry,Blueberry,Gooseberry
Conclusion
The toString() method can be used to convert numbers and arrays to strings in JavaScript. This can be useful if you want to use a string method on a particular object that is not structured as a string, as the function allows you to perform the conversion you need.
In this tutorial, we have explored the basics of strings in JavaScript, and how to use the toString() method to convert numbers and arrays to strings. We have also discussed how to use toString() to convert an array of arrays to a string.
Now you know everything you need to know about the toString() method, so you’re ready to use it in your programs!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.