The JavaScript switch case is a multiple if else
statement. It takes a conditional expression just like an if statement but can have many conditions—or cases—that can match the result of the expression to run different blocks of code.
Conditional statements are an essential part of programming and are used to control the flow of a computer program. The most common form of conditional statement in JavaScript is the “if”
and “if...else”
code block, which can be used to run code only if a certain condition or set of conditions are met.
There is another conditional statement built-in to JavaScript that can be used to evaluate an expression against multiple conditions: the switch case statement. Switch case statements can be used to check if a certain condition is met against multiple cases and will run the blocks of code where that condition is met.
In this tutorial, we are going to discuss how to use the JavaScript switch expression case statement to create conditional statements. We will also explore how to use multiple cases with the switch statement to create more advanced expressions.
JavaScript Conditional Statements Refresher
Conditional statements allow you to run code if a certain condition is met and not execute a block of code if that condition is not met. Conditional statements evaluate whether a condition is met based on the outcome of a true and false statement.
Conditional statements could be useful if you wanted to verify that a user had filled in all the forms on an order page, or to display a message to a user about a product offer only when they are signed in.
In JavaScript, the most common form of conditional statement is the “if”
statement. “if”
statements evaluate whether a statement is true or false, and only run if the statement evaluates to true. Here’s an example of an if statement in JavaScript:
var age = 17; if (age >= 16) { console.log("You are aged 16 or over!"); }
When we run our code, the following output is returned: “You are aged 16 or over!”
The “if”
statement also comes with an “else if”
statement which can be used to check for multiple conditions. If you’re interested in learning more about the JavaScript if and else statements, you can do so by following our tutorial on “if…else” statements.
Now that we know the basics of conditional statements in JavaScript, we can explore the “switch case”
statement.
JavaScript Switch Case
The JavaScript “switch”
statement evaluates a statement and executes a block of code if that statement evaluates to true. “switch”
statements work in a similar way to “if”
statements in that they check if a statement is true, and run if the statement is equal to true.
“switch”
statements are written using the following syntax:
switch (statement) { case a: // Run code break; case b: // Run code break; default: // Run code break; };
There is a lot going on here, so let’s break it down. This is the procedure our program will follow when reading our “switch”
statement:
- The switch expression is evaluated when the statement is run.
- The value of the statement is compared with each of the cases.
- If a case matches our statement, the relevant block of code is run.
- If none of our cases are met, the code in the
“default”
case block will execute.
Let’s use an example to illustrate how this works in action. So, let’s say that you are a coffee shop owner who wants to create a program so users can easily check the price of your popular drinks. In our example, we will evaluate the drink a user chooses against our list of coffees, then print out the price of the drink they have selected.
Here’s the code we would use to allow users to check the price of your popular drinks:
const drink_name = "Americano"; switch (drink_name) { case "Americano": console.log("The price of an Americano is $2.60"); break; case "Cappuccino": console.log("The price of a cappuccino is $2.75"); break; case "Macchiato": console.log("The price of a macchiato is $3.00"); break; case "Espresso": console.log("The price of an espresso is $2.40"); break; default: console.log("This drink is not available"); }
When we run our program with the variable “drink_name”
equal to “Americano,”
the following is returned:
The price of an Americano is $2.60.
If we set “drink_name”
to be equal to a different drink, the corresponding price for that drink would appear. In addition, if we had entered an invalid drink name, our code would have returned the contents of our “default”
statement, which in this case is a message that states “This drink is not available.”
Notice that we add a “break”
keyword at the end of every case statement. If we don’t include a break statement, our program will keep evaluating statements even after one has been met. This is inefficient, and we use the “break case”
keyword to ensure that when our expression is met, our program stops searching through cases.
JavaScript Switch Multiple Cases
In our above example, we used a single case for each expression, and each expression had a different output. But what if we want to have multiple cases yield the same output?
Let’s say that our barista has added two new drinks to the menu, and those drinks cost the same as a macchiato. Instead of writing multiple new cases with their own blocks of code, we can instead use more than one “case”
for each block of code.
We are going to use the above example to illustrate how to use multiple cases. However, in this example, we are going to change “The price of [drink name] is [price].”
to “The price of this drink is [price].”
so that we can reuse our console.log() statements.
We are also going to add two new drinks, a latte and mocha, each priced at $3.00 (the same as a macchiato).
Here is our revised code which includes our new drinks:
const drink_name = "Mocha"; switch (drink_name) { case "Americano": console.log("The price of this drink is $2.60"); break; case "Cappuccino": console.log("The price of this drink is $2.75"); break; case "Latte": case "Mocha": case "Macchiato": console.log("The price of this drink is $3.00"); break; case "Espresso": console.log("The price of this drink is $2.40"); break; default: console.log("This drink is not available"); }
When we run our code above with the “drink_name”
variable equal to “Mocha,”
the following response is returned:
The price of this drink is $3.00.
As you can see, our program has found that “Mocha” is listed as a case, and it has run the code in the relevant block.
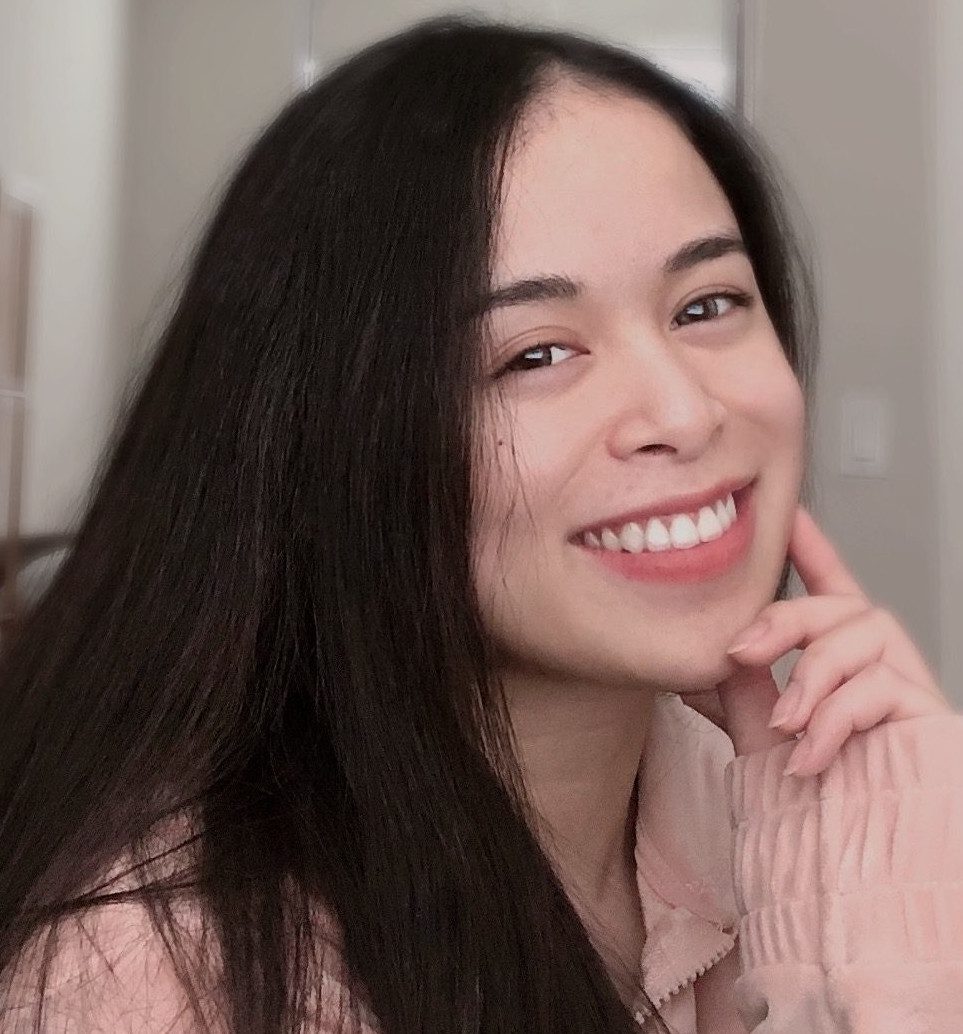
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conclusion
The “switch”
conditional statement can be used to evaluate an expression and return a value depending on whether that expression is met. Switch statements are useful if you want to evaluate a statement against multiple possible outcomes.
In this tutorial, we discussed the basics of conditional statements in JavaScript. We then explored how to use the “switch”
and “case”
statements and went through an example of how to use multiple “case”
statements in a “switch”
block.
Now you have the information you need to use the JavaScript “switch case” statement like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.