The JavaScript indexOf() method returns the position of an item in a list or a substring in a string. The indexOf() JavaScript method returns -1 if a specified value does not appear in a list or string. Only the index value of the first occurrence of a value is returned.
Often, you may want to find out whether the string or array contains a particular value. For example, you may have a list of donut flavors sold in your donut store. You may want to check whether you removed the unpopular triple-chocolate donut from the menu.
That’s where the indexOf() JavaScript method comes in handy. The indexOf() method returns the first index at which a certain value can be found in an array or a string. If a value is not found, a special -1 value is returned.
In this tutorial, we are going to explore the basics of the indexOf() method in JavaScript. We’ll go through two examples to show this method in action to help build your understanding of the indexOf() method.
JavaScript indexOf() with Arrays
The Array.indexOf() built-in method searches through an array for a value. The method finds the index value of the first time a specified item appears in an array. If a specified item is found, the indexOf() method returns the index number of the first occurrence of the value. If the specified item is not found, indexOf() will return -1.
The indexOf() method is case-sensitive. This means indexOf() will return -1 if a value appears in a list with a different case than we have specified.
Here’s the syntax for the indexOf() method in JavaScript:
The indexOf()
method is case sensitive. Here’s the syntax for the indexOf()
function in JavaScript:
array_name.indexOf(item, start_position);
The item parameter is the item for which we are searching in our JavaScript array. This parameter is required. The start_position parameter is optional. start_position is the position in our array at which indexOf() begins searching.
An Example Scenario
Let’s say that we have run out of cinnamon. We want to check if cinnamon donuts are still listed on our shop’s menu. If donuts are on the menu, we will remove them. We could use the following code to perform this action:
var donut_flavors = ['Jam', 'Blueberry', 'Cinnamon', 'Apple Crumble', 'Frosted']; console.log(donut_flavors.indexOf('Cinnamon'));
The indexOf method returns: 2. As you can see, Cinnamon
is present in our array. So, our code has returned the index value of the first instance of Cinnamon
in our array, which in this case is 2
.
The indexOf method returns: 2. As you can see, Cinnamon is present in our array. So, our code has returned the index value of the first instance of Cinnamon in our array, which in this case is 2.
Our program would return 2 even if our array contained multiple instances of Cinnamon. This is because indexOf() only returns the index position of the first instance of an item.
Let’s say that our chef has invented a new Jam Supreme donut and added it to our donut_flavors array. However, she is unsure whether she called the donut Jam or Jam Supreme. She wants to check so that the menu is accurate.
Because Jam is already first in the array, indexOf() will return the position of Jam. However, if we use the start_position parameter, we can skip over the first position and run our search. Here’s the code our chef could use to check whether Jam appears after the index position 1 in the array:
var donut_flavors = ['Jam', 'Blueberry', 'Cinnamon', 'Apple Crumble', 'Frosted', 'Jam Supreme']; console.log(donut_flavors.indexOf('Jam', 1));
Our code returns: -1. While Jam does exist in our array, it comes before the index value 1. The value Jam does not exist in the area through which we are searching.
JavaScript indexOf() with Strings
indexOf() can be used to find a substring within a string. The string indexOf() method returns the index number at which a substring starts in another string.
String Refresher
Like arrays, strings in JavaScript also have their own index values. Strings are indexed from left to right, starting at the index value 0
. So, for our above example string, the following index values would be assigned:
E | x | a | m | p | l | e | ! |
0 | 1 | 2 | 3 | 4 | 5 | 6 | 6 |
So, if we wanted to get the character at the index position 4
, we could do so using the following code:
console.log(example_string[4]):
Our code returns: p
.
String.indexOf()
The String.indexOf() method can be used to check if a string contains a particular substring. If the substring can be found in the string, indexOf() will return the index position of the occurrence of the letter or string; if the substring cannot be found, indexOf() will return -1.
The String.indexOf() method uses the same syntax as the Array.indexOf() method:
string_name.indexOf(substring, start_position)
We must specify a substring argument. This is the value for which we want to search in our string. The start_position argument is optional and tells the program to start the search at a specific index. The start_position argument defaults to index 0.
Let’s say that we have a string with our donut names, and we want to check if that string contains Blueberry. We could use the following code to perform this action:
var donut_flavors = 'Jam Blueberry Cinnamon Apple Crumble Frosted'; console.log(donut_flavors.indexOf('Blueberry'));
Our code returns: 4. As you can see, our donut_flavors
string contains Blueberry
and substring starts at the index position 4.
Our code returns: 4. As you can see, our donut_flavors string contains Blueberry and substring starts at the index position 4.
But what if we wanted to check whether our donut_flavors string contained Blueberry after a specific index position? That’s where the start_position argument comes in.
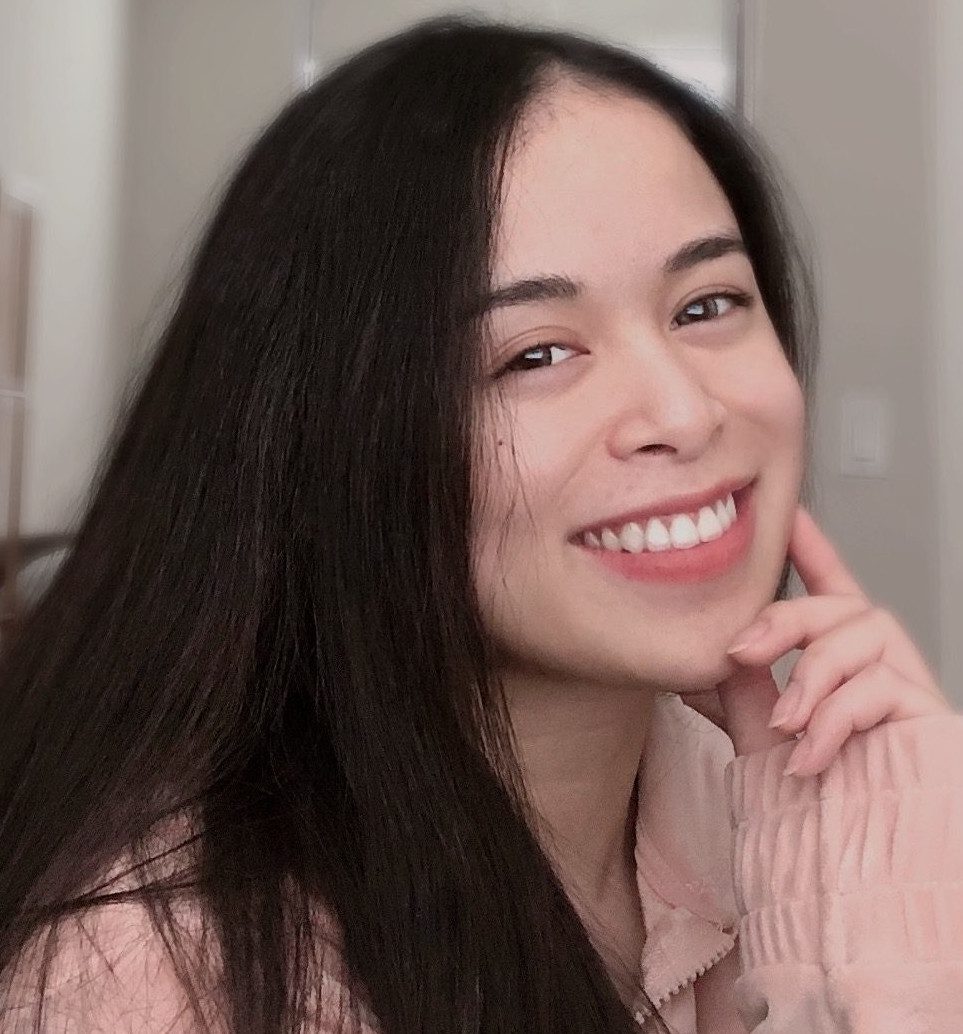
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Let’s search for the term Blueberry in our list. We shall begin our search at the index position 10 in our list:
var donut_flavors = 'Jam Blueberry Cinnamon Apple Crumble Frosted'; console.log(donut_flavors.indexOf('Blueberry', 10));
Our code returns: -1. While “donut_flavors” does include “Blueberry,” the string starts before the index value 10 in the array. Our code returns “-1”. This denotes that the substring could not be found.
Check if a Value Exists Using indexOf()
You can use an “if” statement with the indexOf() method to check if a value exists in a string or an array. For example, you could use indexOf() to check if the donut menu contains a particular value. Then, you could print a message to the console if that value is found.
Let’s write a program that prints a message to the console if “Chocolate” is found in a list of donuts. Here is our code:
var donut_flavors = ['Jam', 'Blueberry', 'Chocolate', 'Cinnamon', 'Apple Crumble', 'Frosted']; if (donut_flavors.indexOf("Chocolate") !== -1) { console.log("Chocolate donuts are on the menu!"); }
Our code returns: “Chocolate donuts are on the menu!”.
On the first line of our code, we declare our array of donut flavors called “donut_flavors,” which contains six values.
Then, we create an if statement that uses indexOf to check if “donut_flavors” contains “Chocolate.” This if statement evaluates whether indexOf returns a value equal to “-1.”
If indexOf returns “-1,” it means our value could not be found in the array. This means our if statement will not execute. If indexOf returns any other value, our if statement will execute.
In this case, indexOf() would return 2. This is because “Chocolate” is present in our “donut_flavors” array. Our program prints out “Chocolate donuts are on the menu!” because indexOf has returned a value that is not equal to -1.
Conclusion
The JavaScript indexOf() method checks whether a string or an array contains a particular value. The method returns the index number at which that value is found in the string or array. If the specified value is not found, indexOf() returns “-1.”
In this tutorial, we discussed the basics of arrays, strings, and how they are indexed. Then, we explored how to use the indexOf() method with both strings and arrays to check whether they contained a specific value.
We discussed how to use the indexOf() method and an “if” statement to check if a value exists in a list or string.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.