To get a JavaScript timestamp format can be achieved using the Date()
object, which holds the current time in a readable timestamp format. You can also create new Date()
objects with different timestamps, and get the current Unix time using the getTime()
method.
Managing dates and times is a crucial part of programming. In JavaScript, for example, you may have to create a dynamic copyright date, a schedule, or a list of events and their times, which all involve using the time/date to some extent.
To work with time in JavaScript, you can use the Date object and its related methods. The Date object allows you to store the date and time, and format the date and time based on your specific needs.
In this tutorial, we are going to explore the basics of creating a JavaScript timestamp using Date. We will also discuss how to format date and timestamps in JavaScript, and how to get UTC time values.
JavaScript Timestamp Object
The Date object is used in JavaScript to store the date and time. Without any arguments, the Date object will create an object with the current date and time. Here’s an example of the Date object being used to get the current date and time:
const currentTime = new Date(); console.log(currentTime);
In our code, we declare a variable called “currentTime,” which we assign a Date object. Then, we print out the variable “currentTime” to the console. Our code returns the following:
Wed Feb 05 2020 11:18:21 GMT+0000 (Greenwich Mean Time)
As you can see, our code has returned the current date and time in a way that we can read. We can break down the result of our Date object into a few components:
- Day: Wed
- Month: Feb
- Day: 14
- Year: 2020
- Hour: 11
- Minute: 18
- Second: 21
- Time Zone: GMT+000 (Greenwich Mean Time)
However, while the date object returns this value, JavaScript understands dates and times through Unix time. Unix time is a value that represents the number of milliseconds that have passed since midnight January 1, 1970, and is the universal standard for storing time in programming.
If we want to retrieve a Unix JavaScript timestamp, we can do so using the getTime() method like this:
console.log(currentTime.getTime());
Our code returns: 1580901774643
. While this number is not as attractive as the information we received above, it means exactly the same thing.
Creating New Date Objects from Existing Timestamps
So far, we have discussed how to create a Date object based on the current time. However, we can also create a Date object based on an existing JavaScript timestamp.
This can be useful if you already have a date value, but want to manipulate it using the JS Date object. For example, you may have a Unix timestamp that you want to appear in the long-form version of time that we showed above (the one that shows the day, time, timezone, and other relevant information).
You can create a new Date object from an existing timestamp in a couple of ways. You can use Date to create a new object based on:
- An existing Unix timestamp (new Date(timestamp))
- A date string (new Date(date string))
- A specific date and time (new Date(year, month, day, hours, minutes, seconds, milliseconds))
Here’s an example of each of these methods in action:
new Date(9231925020);
new Date(“April 17 1970 21:25:25”);
new Date(1970, 3, 17, 21, 25, 25, 0);
Each of these examples creates a Date object using the same information. So, if we print out the time using any of these date objects and the getTime() function like we did earlier, we will get this response:
Fri Apr 17 1970 21:25:25 GMT+0100 (Greenwich Mean Time)
It’s worth noting that, in the last method—where you specify the year, month, day, hours, minutes, seconds, and milliseconds—any value that is missing will be set to 0. In addition, our month is set to “3”
because the JavaScript Date object counts up from “0”
for the months.
Retrieving the Date
Now that we have a Date object, we can retrieve any part of the date or time that we need. We can use the get methods in JavaScript to return certain parts of the date relative to the local timezone. Here are the date methods we can use to retrieve the date:
- Year: getFullYear()
- Month: getMonth()
- Day of the Month: getDate()
- Day of the Week: getDay()
- Hour: getHours()
- Minute: getMinutes()
- Second: getSeconds()
- Millisecond: getMilliseconds()
Let’s create a new date object for January 1st, 2020:
const january2020 = new Date(2020, 1, 1);
Now, if we use the above methods to the the year, month, and day of the month, we’ll get the following:
january2020.getFullYear(); // 1980 january2020.getMonth(); 1 january2020.getDate(); 1
We could run any of the date methods we discussed earlier on our timestamp to get specific parts of the time.
In addition, we can use our Date object to compare times in JavaScript. So, if we wanted to see if it was 2020, we could use the following code:
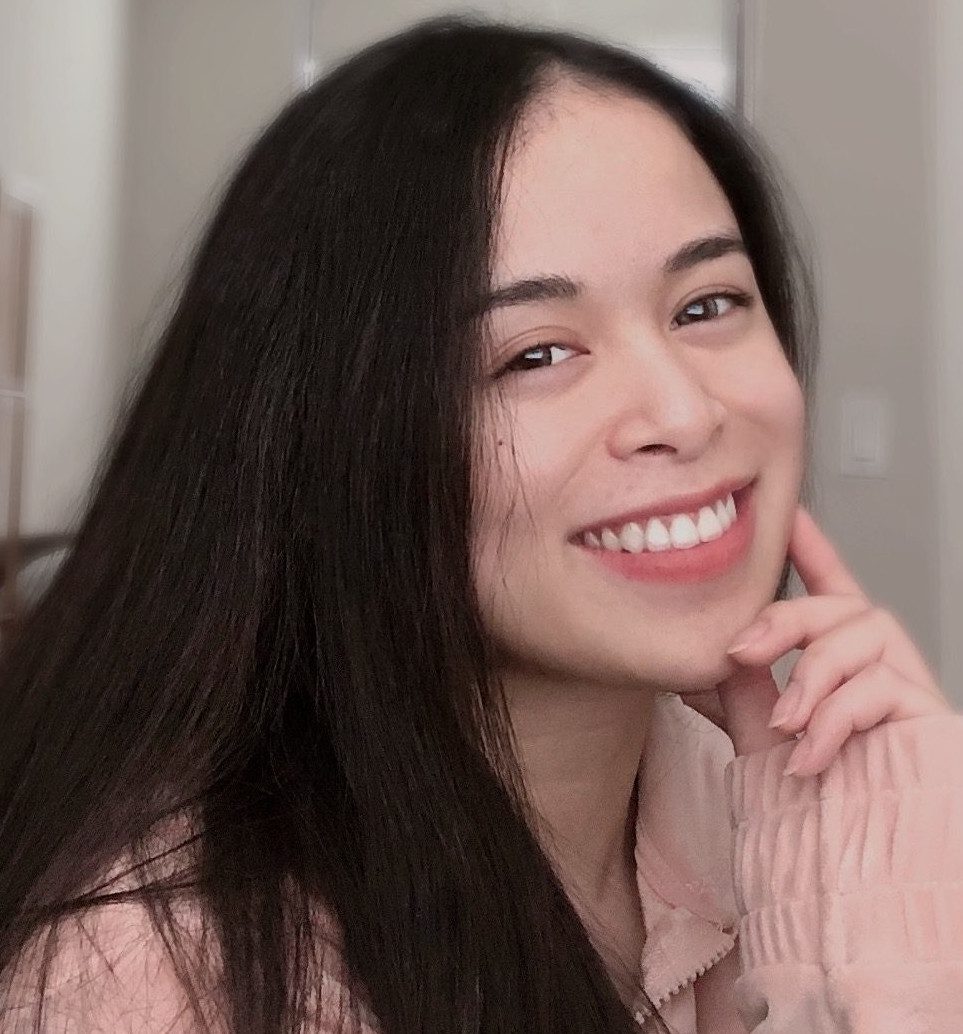
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
const currentDate = new Date(); if (currentDate.getFullYear() === 2020) { console.log("It's 2020!"); } else { console.log("It's not 2020!"); }
Because it is 2020 as this article is being written, our code returns: “It’s 2020!” We could also perform more advanced comparison methods, such as comparing the year and the day using the same approach as we did above.
UTC Methods
The values returned by the date retrieval functions that we discussed above will be based on your local timezone settings. If you want to get UTC timestamps—which calculate time based on Coordinated Universal Time—you should add “UTC”
after the word “get”
in the above functions.
Here’s an example of the getHours() and getUTCHours() methods being used to get the current hour and the hour in the UTC timezone:
const currentDate = new Date(); console.log(currentDate.getHours()); console.log(currentDate.getUTCHours());
Our code returns the following:
11
11
This code prints out both the hour in your current timezone, and the hour of the UTC timezone. If you are currently in UTC, these numbers will be the same (like they are above).
Using UTC can be useful because it is an internationally-accepted standard, and is often used when you are creating a website or an app that depends on multiple timezones.
Conclusion
Time is an important part of JavaScript that you are likely to encounter often when you’re coding. In this tutorial, we discussed how to create a Date object to store the current time. We also discussed how to create custom Date objects based on timestamps, date strings, and specific times, and we explored the built-in date retrieval methods in JavaScript.
Now you’re equipped with the skills you need to retrieve and manipulate timestamps in JavaScript like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.