Variables are an integral part of almost every programming language, and are usually one of the first topics you’ll learn when you start coding. Variables can be used to store data in a program, such as strings, numbers, JSON objects, or boolean values.
In JavaScript, there are three different variable types: var
, let
, and const
. Each of these variables have several rules around how they should be used, and have different characteristics.
In this tutorial, we are going to explore the basics of variables in JavaScript. We’ll discuss how to name a JavaScript variable, when you should use var,
let,
and const
variables, and we will explore how hoisting and scope impact variables.
JavaScript Variables
Variables are used to store data values. For example, a variable may be used to store a user’s email address, or their name. In JavaScript, a variable can contain any types of data, such as a string, a true or false boolean, an object, or a number.
Before the ES6 specification was released, there was one way to declare a variable in JavaScript: var. The var
keyword can be used to declare a variable that is accessible throughout a program, and can be changed.
Here’s an example of a variable being declared using var
in JavaScript:
var full_name = "Alexander Smith";
Our variable can be broken down into a few components:
var
is used to declare our variablefull_name
is the name of our variable=
tells our program that we want to assign a value to our variable (this is called anassignment operator
)Alexander Smith
is the value our variable will store
Now that we have shown off creating a variable in JavaScript, we can use it in our code. Here’s an example of a JavaScript program that uses our variable:
var fullName = "Alexander Smith"; console.log("Your name is:") console.log(fullName)
Our code returns:
Your name is: Alexander Smith
To declare a variable that has no value, you can use the var variableName
code, but without any assignment. Here’s an example of a variable being declared with no value:
var ourExampleVariable;
Then, if we want to assign our variable a value, we can use this code:
ourExampleVariable = "Example!"
Optionally, we can add var
to the start of our assignment, like this:
var ourExampleVariable = "Example!"
Now, if we print ourExampleVariable
to the console, we get the following response: Example!
Our variable can store any data type, as we discussed earlier. Here’s an example of a few variables that have been assigned different data types:
var students = [ { name: "Hannah", age: 12 }, { name: "Alex", age: 13 } ]; var name = "Alexander Smith"; var grade = 82; var passed = true;
How to Name JavaScript Variables
Each programming language has its own rules around how to name variables, and JavaScript is no different. Here are the main rules you should take into account when naming variables in JavaScript:
- Variable names can contain numbers, letters, underscores (_), and dollar signs ($)
- Variable names cannot contain whitespace characters
- Variable names are case sensitive
- Variable names cannot contain any reserved words or reserved keywords (i.e.
break
,default
,with
) - Variable names should not begin with numbers
In addition, JavaScript uses camel case to declare variable names. This refers to writing the first word of a variable in lower case, then capitalizing every future word in the variable. Here’s an example of a variable being declared in camel case:
var fullName = "Bill Jones";
If we only have one word in our variable, every letter should be in lowercase.
Further, if you declare a variable using the const
keyword, every letter should be in uppercase.
While this is a lot of information to learn, over time you’ll naturally be able to figure out how your variables should be named. All you need to do is practice!
Var, Let, and Const Variables
There are three different keywords used to declare a variable in JavaScript. These are: var
, let
, and const
.
Here is a table that breaks down the differences between these three types of variables:
Keyword | Variable Scope | Reassign? | Redeclare? | Hoist? |
var | Function | Yes | Yes | Yes |
const | Block | Yes | No | No |
let | Block | No | No | No |
The following are general rules of thumb for using these types of variables:
- Use const as much as possible, unless you need to redeclare or hoist a variable.
- Use let if you are working with loops.
- Only use var if:
- You are working on legacy code,
- You need a variable that you can redeclare, or
- You need a variable accessible everywhere in the program (i.e., globally).
If you’re interested in learning more about these variable types, check out our tutorial on how to use the JavaScript let
variable here.
This table contains a lot of information, so let’s break down each of the main differences between these variable types: scope
, reassignment
, redeclaration
, and hoisting
.
Scope
Scope is used to refer to where a variable can be accessed within a program. There are two scopes in JavaScript: global scope
, which is where a variable is declared outside a block of code; and local scope
, which is where a variable is declared inside a block of code.
Here’s an example of a global variable in JavaScript:
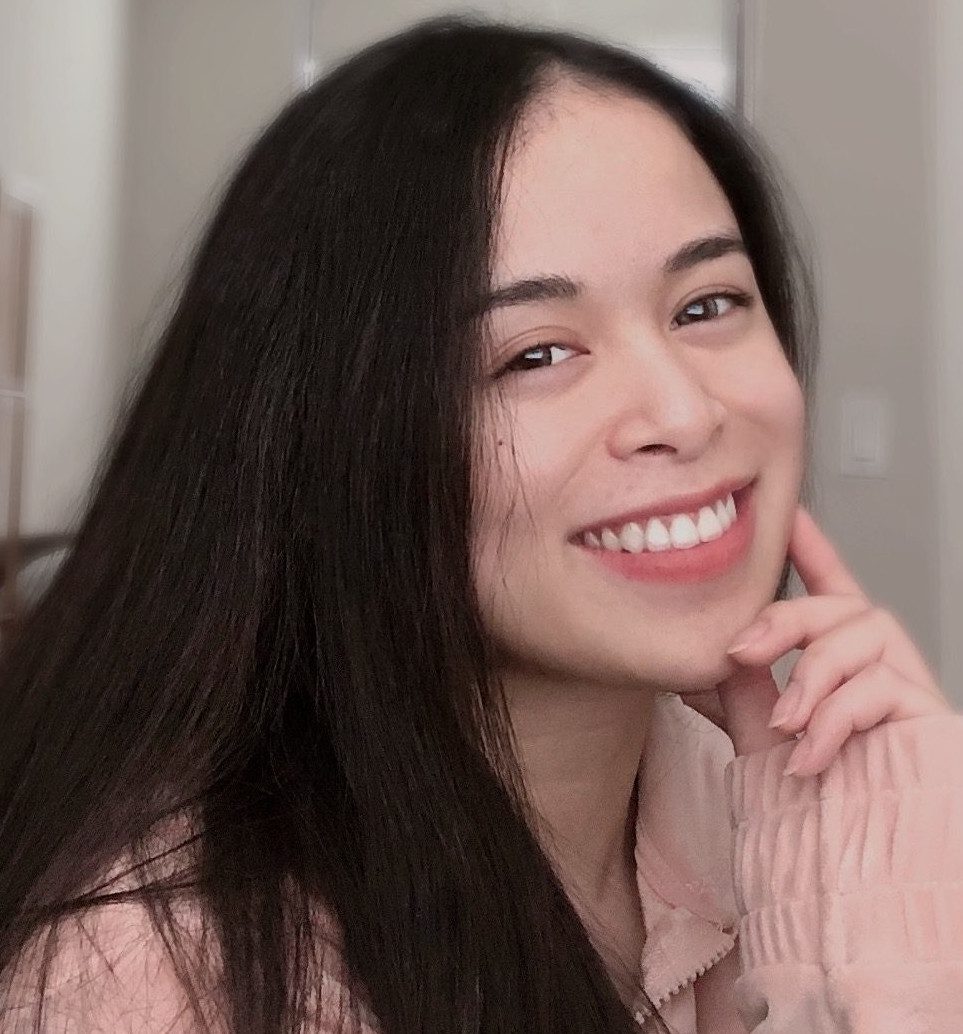
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
var name = "Jimmy Peterson";
This variable can be accessed throughout our entire program. So, if we want to access our name
variable in later functions, we can do so.
Local variables are declared within the scope of a certain block of code. In order to declare a local variable, you should use let
and const
, which are given block scope. Here’s an example of let
being used in a program:
let day = "Monday"; let flavor = "Vanilla"; if (day === "Monday") { let flavor = "Choc-Chip"; console.log(`It is Monday. Have a scoop of ${flavor} ice cream at lunch.`); } console.log(`It is not Monday. Have a scoop of ${flavor} ice cream at lunch.`);
Our code returns the following:
It is Monday. Have a scoop of chocolate ice cream at lunch. It is not Monday. Have a scoop of vanilla ice cream at lunch.
As you can see, because day
is equal to Monday
, our program runs the contents of our if
statement. So, our program changes the value of the flavor
variable to Choc-Chip
and prints out the first message we saw above.
But after the if
statement has executed, the value of flavor
returns to Vanilla
, which was declared in global scope at the start of our program. So, on the final line of code, our program prints out a message asking us to have a scoop of vanilla ice cream, because flavor
was assigned the value Vanilla
in global scope.
Redeclaring Variables
In JavaScript, only var
variables can be redeclared. This means that you can create a new variable with the same name and keep its value, or assign the variable a different value.
Here’s an example of a program that declares a variable called radioShow
, then redeclares the variable:
var radioShow = "KACL"; var radioShow; console.log(radioShow);
Our code returns: KACL
. In our code, we have declared radioShow
twice. Although it is not useful in the above example, if we had a longer program, we may want to redeclare a variable. As a result, if we expect to want to redeclare a variable, we should use var
to declare it.
Hoisting Variables
In JavaScript, a variable can be declared after it has been used, which means that you can use a variable before it has been declared. This behavior is referred to as hoisting.
Let’s use an example to illustrate how hoisting works. Let’s say that we declare a variable called students
which contains a list of student names, but we declare that variable after we ask our program to print it:
console.log(students); var students = ['Andy', 'April', 'Donald'];
Our program returns:
undefined
But if we tried to declare our variable without the var
keyword, our program would return the following:
Uncaught ReferenceError: students is not defined
This shows hoisting in action. Variables can be declared after they are referenced using the var
keyword. In simple terms, our program interpreted our above example like this:
var students; console.log(students); students = ['Andy', 'April', 'Donald'];
Unlike variables declared using var
, let
and const
variables cannot be hoisted. Here’s an example of a program that uses let
to declare a variable:
let name = "Graham Rand"; function exampleFunction() { if (name === "Mark Swinton") { let name = "Mark Swinton"; } console.log(name); } exampleFunction();
When our code runs, the following result will be returned: Graham Rand
. The let name = Mark Swinton;
declaration is enclosed within our function, which means that it has local scope. Because we use the let
keyword, our variable is not hoisted.
In summary, variables using var
are subject to hoisting, which saves variable declarations in memory. This may result in problems if you define and assign your variables in the wrong order.
let
and const
variables are not subject to this feature, however, which means that an error will be returned if you try to declare a variable more than once, or reference a variable that has not yet been declared in the relevant scope.
Conclusion
Variables are an important feature of programming and are used to store data values. In this article, we discussed the basics of JavaScript variables, and listed the rules to follow when naming variables.
We also discussed the three main types of variables in JavaScript — var
, let
, and const
— and explored how they can be used. Finally, we discussed the role of scope, redeclaring variables, and hoisting in JavaScript variables.
Now you have the knowledge you need to declare and use variables like a JavaScript expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.