A JavaScript if…else statement evaluates whether a condition is true or false. If a condition is executed, the code within the if block executes. Otherwise, the contents of the else block execute. Else…if statements let you evaluate multiple conditions.
There will be times when you want different blocks of code to run, depending on certain factors.
This is where conditional statements come in. Conditional statements are a crucial part of all programming languages. They run blocks of code if a particular condition is met.
In this tutorial, we’re going to look at how to use if…else statements in our code.
Conditional Statements Refresher
As we’ve discussed, conditional statements are an important part of every programming language. You’ll need to use a conditional statement to run code if a particular condition is met. Here are a few scenarios where using a conditional statement would be useful:
- Check if a user’s password matches the one on file.
- Check if a user has an account listed under a certain email address.
- Submit a form if every field has been filled out.
- Display a list of holiday discounts if a user is signed in.
The key to all of these examples is that they use the word if. If something happens, then something else should happen. Let’s explore how we can use this same logic in JavaScript.
JavaScript if Statement
A JavaScript If statement evaluates whether a statement is true or false. An if statement will only run if the statement is true. If the statement is false, nothing happens.
If statements use the if keyword. After the if statement, you should specify a condition—or a set of conditions—in regular brackets. The contents of an if and else statement appear enclosed within curly braces.
Here’s the syntax for an if statement in JavaScript:
if (ourConditions) { // The code that will execute if ourConditions are true }
if Statement JavaScript Example
Let’s use an example to illustrate the if statement in action. We’re building an e-commerce site, and we need to ensure that users are over the age of 16 before making a purchase. Here’s an example of how we could verify the age of users before allowing them to purchase an item at our store:
const userAge = 15; if (userAge < 16) { console.log("You are not old enough to make a purchase!"); }
Our code returns the following:
You are not old enough to make a purchase!
Using the less-than operator, we check whether our user’s age is less than 16. Our user is 15, which means that userAge < 16 is true. The code within our if statement runs.
But what if our user’s age was 16? If that were the case, our code would return nothing because userAge < 16 would be false. The code within our if statement is ignored. Our program would keep running.
JavaScript if…else Statement
A JavaScript if…else statement evaluates whether a condition is equal to true. If a condition is equal to false, the else statement executes. Otherwise, the contents of the “if” statement run.
The syntax for the if…else statement is:
if (ourCondition) { // Code will execute if the condition is true } else { // Code will execute if the condition is false }
The else statement appears after all the if conditions in a statement. Only one else statement can appear in an if statement.
To learn more about JavaScript true and false, read our guide to JavaScript Booleans.
if…else JavaScript Example
Let’s use the same example from our if statement discussion where we check a user’s age. We will add an else statement to tell a user that their purchase is going through.
const userAge = 17; if (userAge < 16) { console.log("You are not old enough to make a purchase!"); } else { console.log("Your purchase is being processed."); }
Our code returns the following:
Your purchase is being processed.
We declare a JavaScript variable called “age” which tracks our user’s age. Because our user is older than 16, the code skips over the if statement and executes the else clause. If our user age was 12, the code within our else clause would not run.
Else statements can be useful in a variety of contexts. For example, you can use an else statement to handle an error if one comes up. Or you can use an else statement to verify the contents of a form. You could show users a message if they have made a mistake or not filled in the form.
JavaScript else…if Statement
The JavaScript else…if statement evaluates whether one of multiple conditions is true. Each if…else statement evaluates a new condition. If no conditions are met, nothing happens.
Here is the syntax for using multiple else…if statements:
if (condition one) { // Code will run if condition one is true } else if (condition two) { // Code will run if condition two is true } else { // Code will run if all the above conditions are false }
Our program will run all of the statements in order—starting with condition one—and if none of the statements are true, the code within our else
block will run.
We have specified one else…if statement. You can use as many else…if statements as you want with an if statement. Within each statement you should specify the code to be executed if that particular condition is met.
else…if JavaScript Example
Let’s use an example to illustrate an else…if conditional expression in action. Say you are running a movie theater and want to give customers different prices depending on their age. Here are the prices you want to use:
- Aged 10 or under: $5.
- Aged 11-17: $8.
- Aged 18-64: $12.
- Aged 65+: $8.
Here’s an example of a program that will check a customer’s age and return the price they should pay for a ticket:
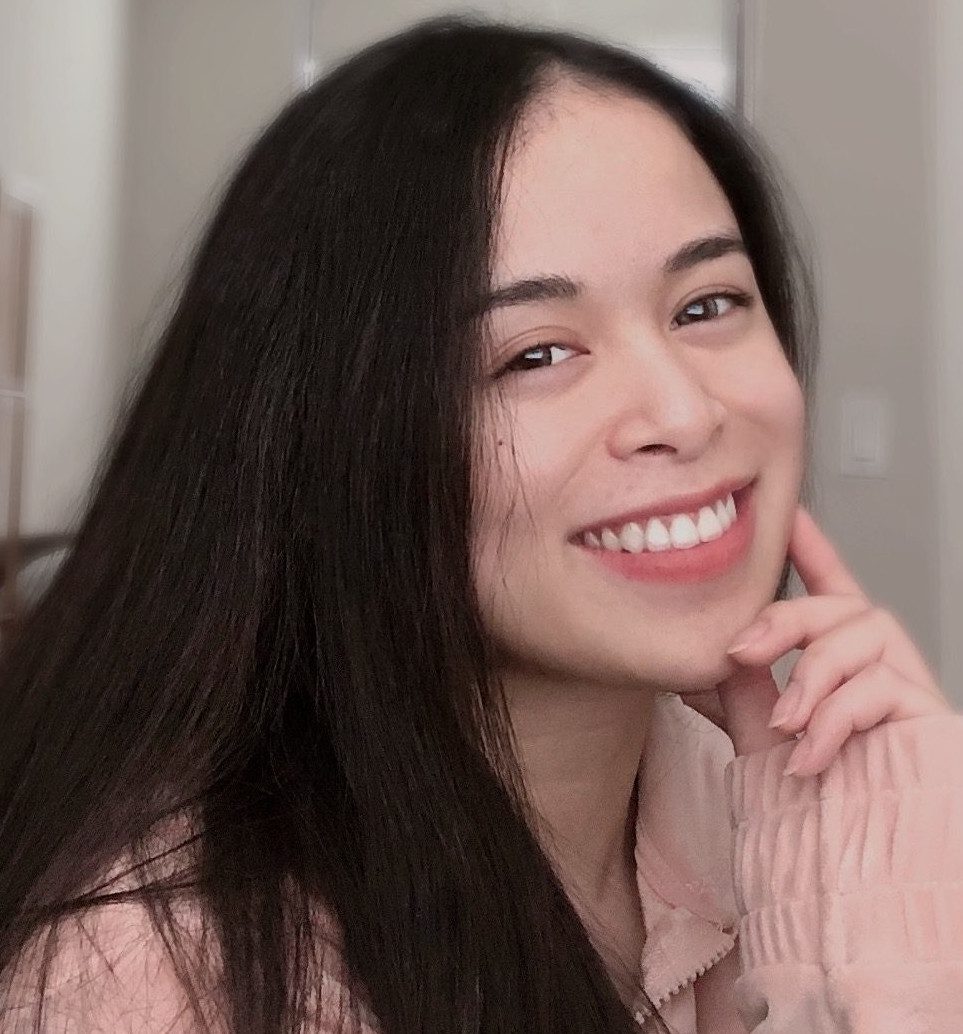
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
var age = 27; var ticketPrice = 0; if (age <= 10) { var ticketPrice = 5; } else if (age >= 11 && age <= 17) { var ticketPrice = 8; } else if (age >= 18 && age <= 64) { var ticketPrice = 12; } else { var ticketPrice = 8; } console.log(ticketPrice);
Our code returns the following: 12.
We check if a user’s age is equal to 10 or is below 10. After that, we check if our user is between the ages of 11 and 17. We then check if the user is between the ages of 18 and 64. Because our user’s age is 27, the code in that block executes. Thus, the ticket price becomes $12.
Conclusion
JavaScript if else statements allow us to determine which code in a program should be run depending on certain factors being met. Every programming language uses conditional statements to some degree to provide logic in the code.
In this guide, we discussed how to use the if, if else, and else…if statements in JavaScript. Now you’re ready to start using conditional operators like a pro!
If you’re looking for more learning resources and guidance on top JavaScript courses, read our How to Learn JavaScript guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.